What is Unit testing?
Unit testing is a testing methodology where individual units are tested in isolation from other units. This is usually done by developers. A unit can be considered as a class or method inside a class which needs to be tested individually. It is also known as White Box Testing, as developer is able to see the code for the functionality.
What is Junit?
To do Unit testing in Java we have an excellent framework called Junit. Junit is a unit testing framework. This framework provides us with the following facilities
- Base classes and Annotations to write unit tests
- Base class support to run tests, TestRunner class.
- Base class and Annotation support to write test suites, @RunWith(Suite.Class)
- And of course reporting of test results.
This tutorial has the following sections
- Downloading Junit jars
- Adding reference to the Junit jar in your project.
- Defining and writing our application under test.
- Writing our first simple JUnit test.
Choice of development environment is Eclipse.
Step 1: Downloading JUnit Jars
To use Junit we need to have 'hamcrest-core-1.3-sources.jar'& 'junit-4.10.jar' Jar files included in the java project. You can download these Jars from 'https://github.com/junit-team/junit/wiki/Download-and-Install'.
Step 2: Setting up your project in Eclipse IDE
I hope you have gone through our tutorials in installing and setting up Eclipse here. Create a project in eclipse and name it 'JUnit Project'.
Step 3: Add Junit Jar files
Well, there is nothing like installing Junit. All you have to do is to reference your Junit jars to your project. This can be done following these steps:
- Right-click your project in the Package Explorer and Go to properties.
- Select Java Build path from the left pane.
- Click on Add External Jars in the right pane.
- Browse to the path where you have downloaded the jar files and include both the jars.
What is our application under test?
To perform unit testing you need to have an application (Development code you may say). Lets first define a simple application which will be our Application under test (AUT). Let's say that we have a small Math class which provides us with the following methods:
- Add
- Multiply
- Subtract
These methods will do exactly as they say. They will add, multiply and subtract two numbers. Now, this Math class is our AUT. Here is my simple implementation of this class.
Math Provider Class
package Application;
/*
* This is our sample class that we would like to unit test
* To do this we will create a sample functionality in the
* class
* Let the class give us these 3 basic functionalities
* Substract()
* Add()
* Multiply()
*
* Our goal is to have unit tests around these
* basic functionalities
*/
public class MathProvider {
public MathProvider(){}
public int Substract(int firstNumber, int secondNumber)
{
return (firstNumber - secondNumber);
}
public int Add(int firstNumber, int secondNumber)
{
return (firstNumber + secondNumber);
}
public int Multiply(int firstNumber, int secondNumber)
{
return (firstNumber * secondNumber);
}
}
We have to unit test MathProvider. What is it that typically to be tested inside the 'MathProvider' class? We can see that this class is providing three core functionalities:
- To add
- To Subtract
- To Multiply
This will be our tests. We will verify that this class is able to give correct addition, subtraction and multiplication results. Copy this class and add it inside your project in Eclipse. Also, for the sake of arranging tests and application code part of the project lets create two folders inside
- Application
- UnitTests
Add the MathProvider class inside the Application folder. This sets us up for writing our first unit test in Junit framework. Please follow us to the next tutorial.
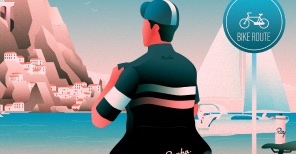
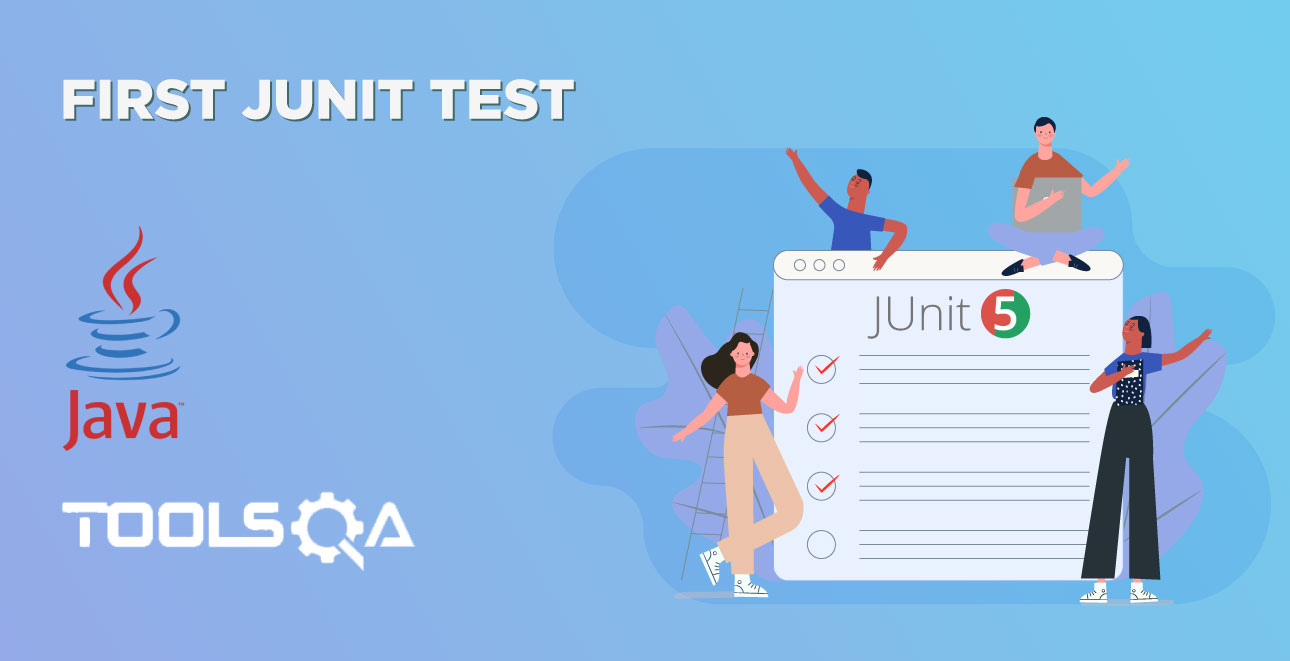