In this tutorial, we are going to cover some of the Robot Class Keyboard Events.
As discussed in the Robot class Introduction tutorial, the Robot class provides methods that can be used to simulate keyboard and mouse actions, e.g., simulation on OS popups/alerts and even on OS applications like Calculator, Notepad, etc.
Keyboard methods in Robot class
To simulate keyboard actions, following Robot class methods should be used :
Keyboard methods:
- keyPress(int keycode): This method presses a given key. The parameter keycode is an integer value for the key pressed. For example, to press a key for alphabet A, the value that has to pass is KeyEvent.VK_A i.e., keyPress(KeyEvent.VK_A).
- KeyEvent is generally a low-level event. In Java AWT, low-level events are events that indicate direct communication from the user like a keypress, key release or a mouse click, drag, move, or release, etc. KeyEvent indicates an event that occurs on pressing, releasing, or typing a key on the component object like a text field.
- This KeyEvent class has various constant fields like VK_0, VK_1 till VK_9 of type integer. These values are the same as ASCII code for numbers '0' till '9'. Similarly, for alphabets, this class has constant fields like VK_A, VK_B till VK_Z. It also has constant fields for representing special characters like VK_DOLLAR for "$" key, modifier keys like VK_SHIFT for Shift key, etc.
- keyRelease(int keycode): This method releases a given key. For Example, the Shift key pressed using the *keyPress(KeyEvent.VK_SHIFT *) method needs to release using the keyRelease (KeyEvent.VK_SHIFT ) method.
Practice Exercise to Perform Keyboard events using java Robot Class in Selenium
Let’s discuss an example from an already available demo page on Toolsqa as “https://demoqa.com/keyboard-events/“.
Here, to Upload any file, select the file first from Open popup which is Desktop Windows popup. Which, in turn, requires the filename to enter, e.g., D1.txt in this example.
Let's understand how to use the Robot class method to enter a filename.
- Instantiate Robot Class
Robot robot = new Robot();
- Invoke the keyPress method to enter the text
robot.keyPress(<keycode integer value>);
If a user needs to enter "D1.txt" in a textbox on a Webpage, he/she can use the sendKeys method, and just a single method call sendKeys("D1.txt") will suffice the purpose. But, to enter text in Desktop Windows popup using keyPress() method, the method needs to be invoked for each character of the input string, like keyPress(KeyEvent.VK_D), keyPress(KeyEvent.VK_1), etc.
Let us automate the following scenario :
- Launch the web browser and launch our practice page https://demoqa.com/keyboard-events/
- Click on ‘Click here to browse’ button
- Press Shift Key
- Enter d to type it as D as the modifier Shift key press
- Release Shift Key
- Enter remaining part of the file name, i.e., 1.txt to display it as D1.txt
- Press Enter key
- Click on the Upload button and close the alert
- Close the browser to end the program
Prerequisite: Create a file C:\demo\D1.txt
Following is the sample code:
package com.toolsqa.tutorials.actions;
import java.awt.AWTException;
import java.awt.Robot;
import java.awt.event.KeyEvent;
import java.io.IOException;
import org.openqa.selenium.Alert;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class RobotKeyboardDemo {
public static void main(String[] args) throws InterruptedException, AWTException, IOException {
System.setProperty("webdriver.gecko.driver","C:\\Selenium\\lib\\geckodriver-v0.24.0-win64\\geckodriver.exe");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
String URL = "https://demoqa.com/keyboard-events/";
//Start Browser
driver.get(URL);
//maximize browser
driver.manage().window().maximize();
Thread.sleep(2000);
// This will click on Browse button
WebElement webElement = driver.findElement(By.id("browseFile"));
//click Browse button
webElement.sendKeys(Keys.ENTER);
//Create object of Robot class
Robot robot = new Robot();
//Code to Enter D1.txt
//Press Shify key
robot.keyPress(KeyEvent.VK_SHIFT);
//Press d , it gets typed as upper case D as Shift key is pressed
robot.keyPress(KeyEvent.VK_D);
//Release SHIFT key to release upper case effect
robot.keyRelease(KeyEvent.VK_SHIFT);
robot.keyPress(KeyEvent.VK_1);
robot.keyPress(KeyEvent.VK_PERIOD);
robot.keyPress(KeyEvent.VK_T);
robot.keyPress(KeyEvent.VK_X);
robot.keyPress(KeyEvent.VK_T);
//Press ENTER to close the popup
robot.keyPress(KeyEvent.VK_ENTER);
//Wait for 1 sec
Thread.sleep(1000);
//This is just a verification part, accept alert
webElement = driver.findElement(By.id("uploadButton"));
webElement.click();
WebDriverWait wait = new WebDriverWait(driver, 10);
Alert myAlert = wait.until(ExpectedConditions.alertIsPresent());
//Accept the Alert
myAlert.accept();
//Close the main window
driver.close();
}
}
Note: Even though Robot class specifies to follow keyRelease for each keyPress event, Alphabets and numbers don't have any side effects on the next statements. Therefore, generally, users skip the keyRelease event for Alphabets and numbers. On the other hand, all the modifier keys such as SHIFT, ALT, etc. will always have a side effect on the next statements. As a result, it is still mandatory to specify keyRelease for each keyPress event of the modifier keys.
Just Try commenting out robot.keyRelease(KeyEvent.VK_SHIFT); in the above sample code and run the script. You will notice the file name types as, 'D!.TXT'. It is because the key pressed effect for the SHIFT key gets carried forward to the next typed text and types it in the uppercase.
Summary
To conclude, in this tutorial, we have covered Robot keyboard methods used to interact with operating system specific popup or applications in Selenium script.
- keyPress(int keycode): Method to press a given key.
- keyRelease(int keycode): Method to release a given key.
As already briefed in the Robot introduction tutorial, the Robot class also provides methods to simulate mouse actions. We will cover those methods in detail in the next tutorial, i.e., Robot Class Mouse Events.
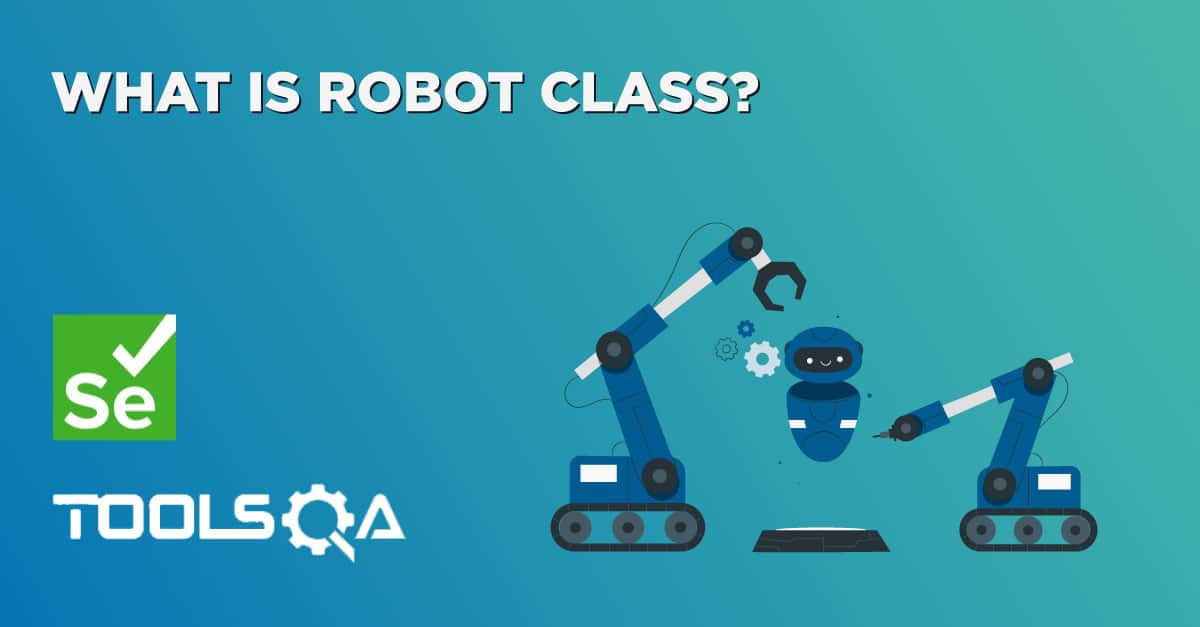
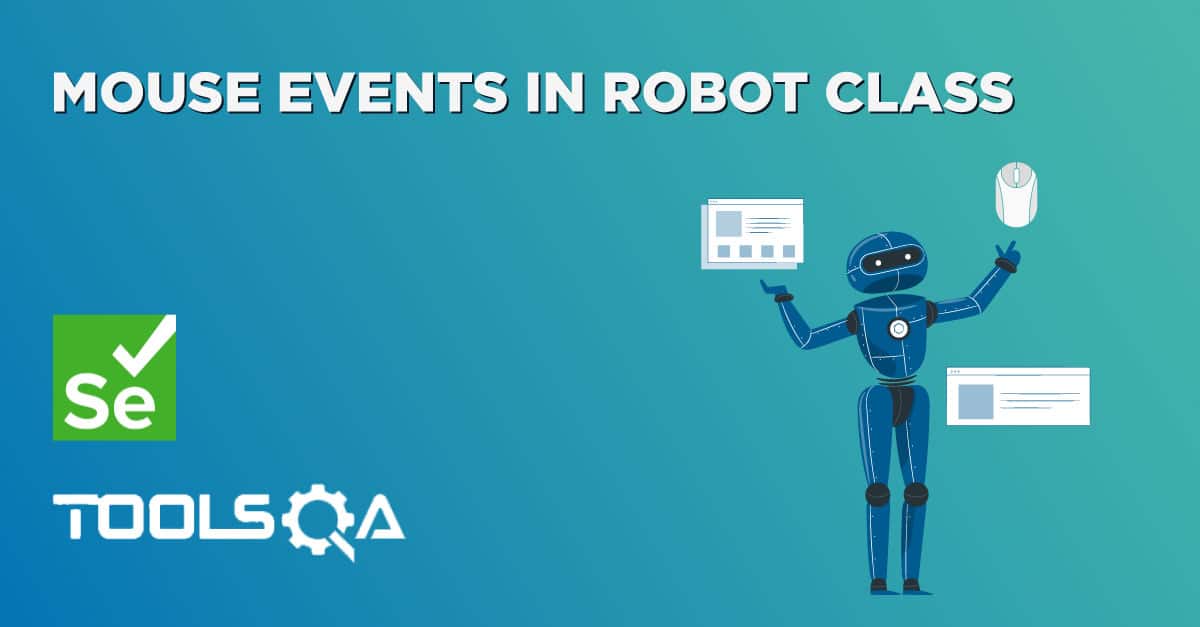