This chapter is about File Reader Manager as Singleton Design Pattern. Sometimes it's appropriate to have exactly one instance of a class. These are accessed by disparate objects throughout a software system, and therefore require a global point of access. In our case, we have ConfigReaderFile, which should be accessed globally. But later on in this Selenium Cucumber Framework series, we will be having many more file readers. So it is better to have a File Reader Manager above all the File Readers. And it is better to make the manager class as singleton.
What is Singleton Design Pattern?
The Singleton's purpose is to control object creation, limiting the number of objects to only one. Since there is only one Singleton instance, any instance fields of a Singleton will occur only once per class, just like static fields.
How to implement Singleton Pattern?
To implement Singleton pattern, we have different approaches but all of them have the following common concepts.
- Private constructor to restrict instantiation of the class from other classes.
- Private static variable of the same class that is the only instance of the class.
- Public static method that returns the instance of the class, this is the global access point for outer world to get the instance of the singleton class.
File Reader Manager as Singleton Design Pattern
- Create a New Class and name it as FileReaderManager, by right click on the managers package and select New >> Class.
FileReaderManager.java
package managers;
import dataProviders.ConfigFileReader;
public class FileReaderManager {
private static FileReaderManager fileReaderManager = new FileReaderManager();
private static ConfigFileReader configFileReader;
private FileReaderManager() {
}
public static FileReaderManager getInstance( ) {
return fileReaderManager;
}
public ConfigFileReader getConfigReader() {
return (configFileReader == null) ? new ConfigFileReader() : configFileReader;
}
}
Explanation
- The FileReaderManager class maintains a static reference to its own instance and returns that reference from the static getInstance() method.
- FileReaderManager implements a private constructor so clients cannot instantiate FileReaderManager instances.
- getConfigReader() method returns the instance of the ConfigFileReader but this method is not static. So that client has to use the getInstance() method to access other public methods of the FileReaderManager like FileReaderManager.getInstance().getConfigReader()
- Now start using FileReaderManager in test code.
Steps.java
package stepDefinitions;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import cucumber.api.java.en.Given;
import cucumber.api.java.en.When;
import managers.FileReaderManager;
import managers.PageObjectManager;
import pageObjects.CartPage;
import pageObjects.CheckoutPage;
import pageObjects.HomePage;
import pageObjects.ProductListingPage;
public class Steps {
WebDriver driver;
HomePage homePage;
ProductListingPage productListingPage;
CartPage cartPage;
CheckoutPage checkoutPage;
PageObjectManager pageObjectManager;
@Given("^user is on Home Page$")
public void user_is_on_Home_Page(){
System.setProperty("webdriver.chrome.driver", FileReaderManager.getInstance().getConfigReader().getDriverPath());
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(FileReaderManager.getInstance().getConfigReader().getImplicitlyWait(), TimeUnit.SECONDS);
pageObjectManager = new PageObjectManager(driver);
homePage = pageObjectManager.getHomePage();
homePage.navigateTo_HomePage();
}
@When("^he search for \"([^\"]*)\"$")
public void he_search_for(String product) {
homePage.perform_Search(product);
}
@When("^choose to buy the first item$")
public void choose_to_buy_the_first_item() {
productListingPage = pageObjectManager.getProductListingPage();
productListingPage.select_Product(0);
productListingPage.clickOn_AddToCart();
}
@When("^moves to checkout from mini cart$")
public void moves_to_checkout_from_mini_cart(){
cartPage = pageObjectManager.getCartPage();
cartPage.clickOn_Cart();
cartPage.clickOn_ContinueToCheckout();
}
@When("^enter personal details on checkout page$")
public void enter_personal_details_on_checkout_page(){
checkoutPage = pageObjectManager.getCheckoutPage();
checkoutPage.fill_PersonalDetails();
}
@When("^select same delivery address$")
public void select_same_delivery_address(){
checkoutPage.check_ShipToDifferentAddress(false);
}
@When("^select payment method as \"([^\"]*)\" payment$")
public void select_payment_method_as_payment(String arg1){
checkoutPage.select_PaymentMethod("CheckPayment");
}
@When("^place the order$")
public void place_the_order() {
checkoutPage.check_TermsAndCondition(true);
checkoutPage.clickOn_PlaceOrder();
driver.quit();
}
}
HomePage.java
package pageObjects;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.PageFactory;
import managers.FileReaderManager;
public class HomePage {
WebDriver driver;
public HomePage(WebDriver driver){
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void perform_Search(String search) {
driver.navigate().to(FileReaderManager.getInstance().getConfigReader().getApplicationUrl() + "/?s=" + search + "&post_type=product");
}
public void navigateTo_HomePage() {
driver.get(FileReaderManager.getInstance().getConfigReader().getApplicationUrl());
}
}
The rest of the classes will remain the same.
Run the Cucumber Test
Run as JUnit
Now we are all set to run the Cucumber test. Right, Click on TestRunner class and Click Run As >> JUnit Test. Cucumber will run the script the same way it runs in Selenium WebDriver and the result will be shown in the left-hand side project explorer window in JUnit tab.
Project Explorer
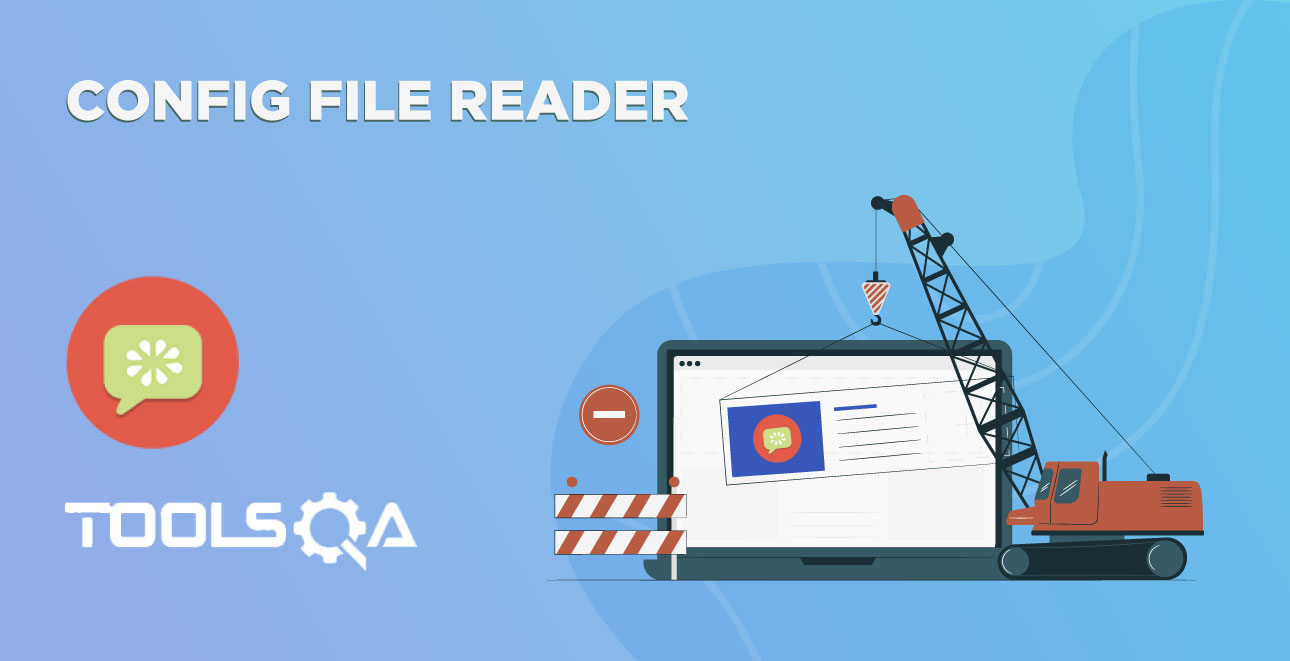
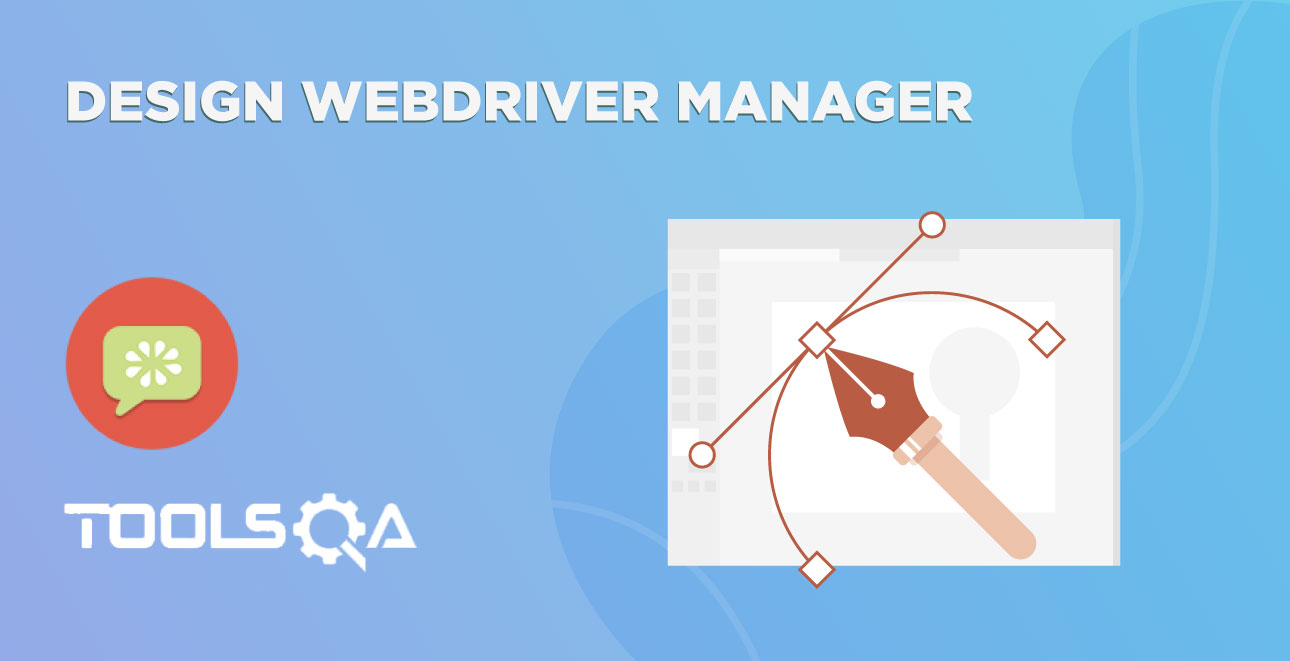