Till now we have learned how to write a unit test and run that test. But running a test one by one is a pain and it is not even manageable as in any project there will be many Junit tests. Junits can be in different classes and different methods. To overcome this situation Junits Framework gives the ability to call all the tests from a main[ ] test which is called Test Runner.
Now let's see what a Test Runner class is and how we can use it. Test Runner is a class that runs a test or group of tests. This class is nothing but a class with main[ ] method which calls the JunitCore class of junit framework. JUnitCore class is responsible for running tests. This class contains a method called JUnitCore.RunClasses(Class Testclass)
Step 1: Create a JUnit Test Runner Class
- First Import all the relevant namespaces
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
First Import - junit.runner.JunitCore : This statement is loading JUnitCore class.
Second Import - junit.runner.Result : This statement is to load the Result class to get results of the test run.
Third Import - junit.runner.notification.Failure : This statement is to load the Failure class to get all the failures.
- Write the code in the main[ ] method.
public static void main(String[] args) {
//This will run JunitMathProvider_1 class.
Result result = JUnitCore.runClasses(JunitMathProvider_1.class);
System.out.println("Total number of tests " + result.getRunCount());
System.out.println("Total number of tests failed " + result.getFailureCount());
for(Failure failure : result.getFailures())
{
System.out.println(failure.getMessage());
}
System.out.println(result.wasSuccessful());
}
NOte : Here we used the JunitCore class to run Classes. From our previous tutorial, we had created our test class named 'JunitMathProvider_1' and to run that class the Statement JUnitCore.runClasses(JunitMathProvider_1.class) is used.
Complete Test Runner class will look like this:
package junits;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class TestRunner {
public static void main(String[] args) {
//This result object has many methods and it is very useful
//Type result and press dot, all the methods will display
//This statement is to load all type of results in the result object
Result result = JUnitCore.runClasses(JunitMathProvider_1.class);
//Here it is getting the run count from the result object
System.out.println("Total number of tests " + result.getRunCount());
//This is to get the failure count from the result object
System.out.println("Total number of tests failed " + result.getFailureCount());
for(Failure failure : result.getFailures())
{
//This will print message only in case of failure
System.out.println(failure.getMessage());
}
//This will print the overall test result in boolean type
System.out.println(result.wasSuccessful());
}
}
Note: How does the runner finds all the tests inside the test case class (JunitMathProvider_1)?
Well, if you remember we had a @Test annotation that we used above the methods which were test methods. This is how TestRunner finds all the tests in a class.
Your test, project window and test result would look like this:
I hope you are clear with what a TestRunner is and how you can use it. Now lets move on to write some more test classes.
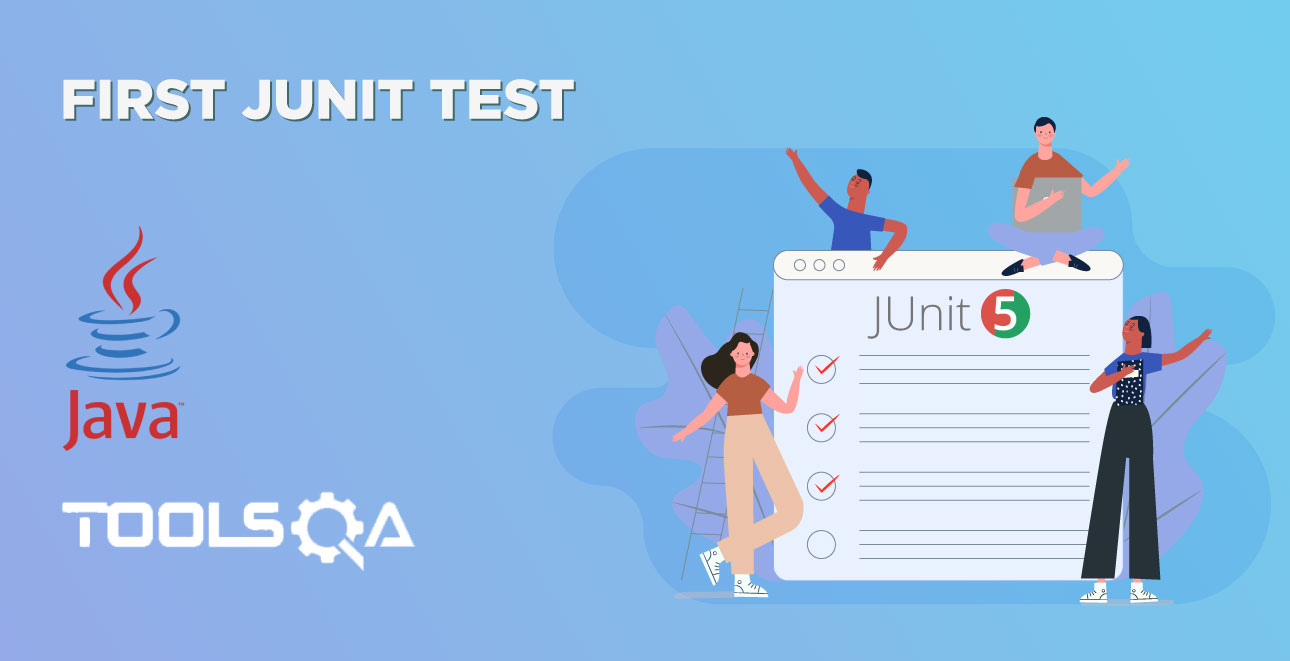
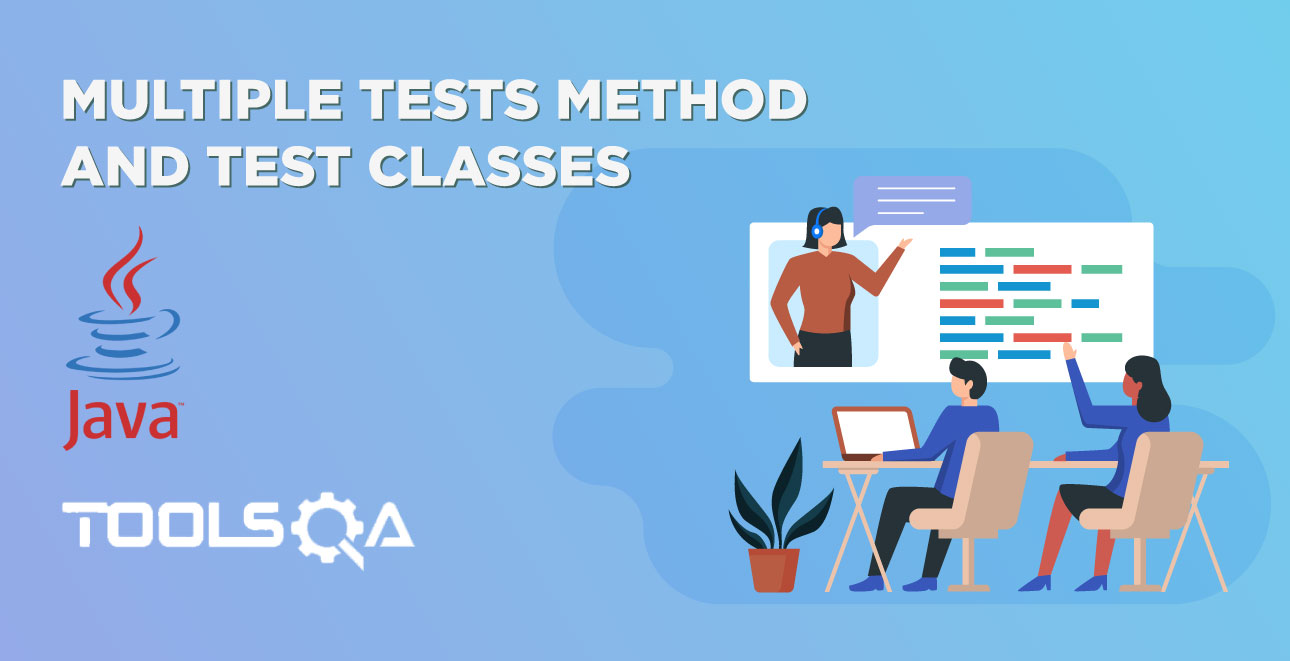