Till now we know how to add tests and how to run them. We also learned how to create multiple test classes. Now, let's find out a way to group our tests to create test suites. Test suite is a really important concept, in the sense that it allows us to group multiple tests based on some common criteria. For e.g. you can group all your Database tests together, similarly, all your test specific to a module can be grouped together.
Create a JUnit Test Suite Class
To create a test suite we will use the annotation @RunWith and @Suite.SuiteClasses. We will create a TestSuite class and annotate it with these two annotations.
Test Suite Class:
package junits;
import org.junit.runner.RunWith;
import org.junit.runners.Suite;
/*
* This is a test suite class that we will create to run all our tests
* Follow along to see how its done Pay special attention to annotations
*/
@RunWith(Suite.class)
@Suite.SuiteClasses({
JunitMathProvider_1.class,
JunitMathProvider_2.class
})
public class TestSuite {
}
Let's go line by line to understand what is happening.
- Imports
import org.junit.runner.RunWith;
import org.junit.runners.Suite;
Here we are importing both @RunWith and @Suite annotations
- Test Suite Annotations
@RunWith(Suite.class)
Here we have just declared an empty class, but annotated it with @RunWith(Suite.class) and Suite.SuiteClasses({}). RunWith annotation actually tells the Junit runner that this class (TestSuite) will not use the default Junit runner but will like to delegate the run to the class mentioned in the parameter of @RunWith annotation. So with this in mind, if we look at what we have written @RunWith(Suite.class). It says to the Junit runner that don't run the class with default test runner delegate it to a class called Suite. Suite is a class in the Junit framework which helps us run build test by adding multiple test classes.
Now let see the second line
@Suite.SuiteClasses({
JunitMathProvider_1.class,JunitMathProvider_2.class
})
Here we are saying the Suite class should add Junit test classes named JunitMathProvider_1 and JunitMathProvider_2. These two tests are grouped together by this statement and while running tests will be executed together. Once you add this class you will see that Junit will run the test in this class also. For your exercise, run your tests after adding a test suite.
I hope that these tutorials have given you a start on the Junit framework. These tutorials have set a base for you, on which you can build up to increase your knowledge in the Junit framework.
Hope to see you soon in my next tutorials, you can suggest me some topic to write on at [email protected]
Happy learning!
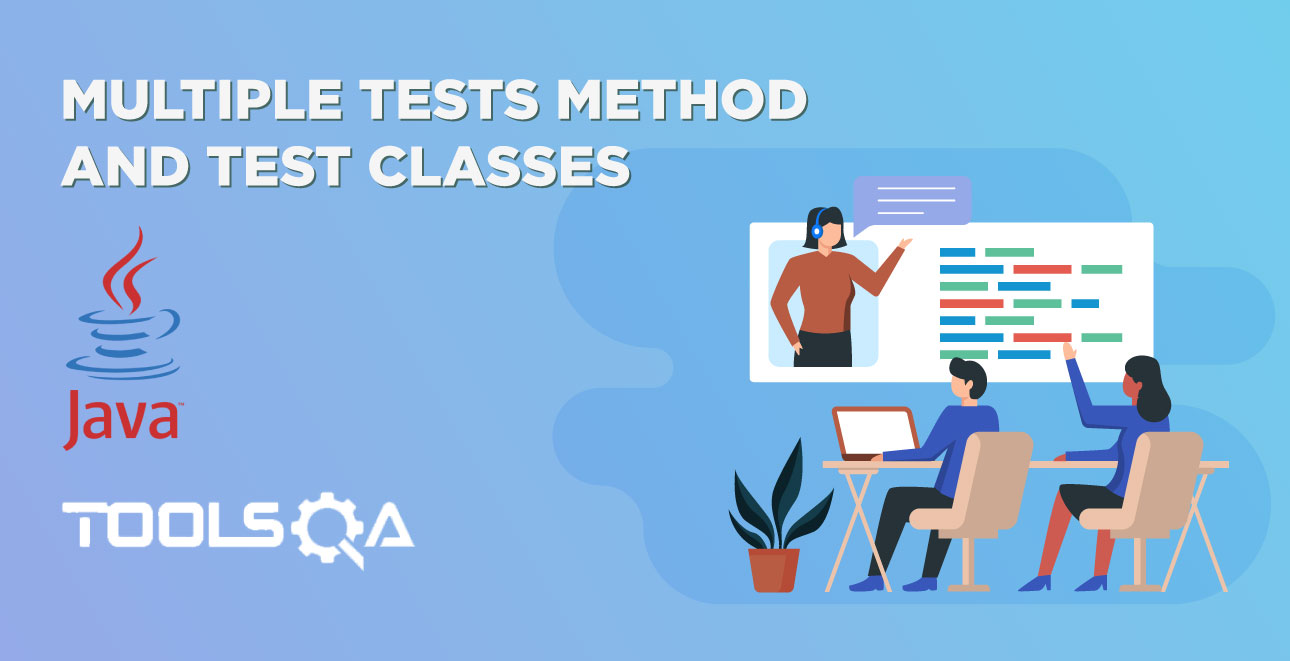
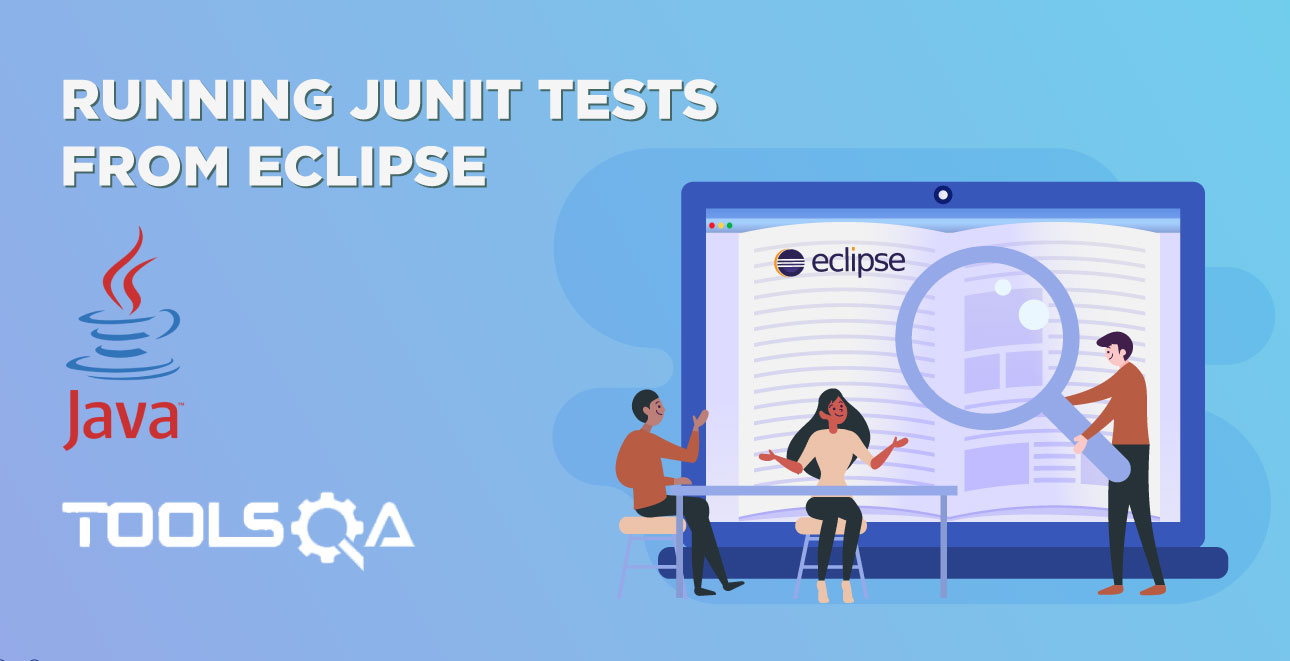