In the protractor basics article, we have got a glimpse of jasmine framework. In this article we discuss alot on different ways of Jasmine Test
- What is "describe-block" in Jasmine?
- What is "it-block" in Jasmine?
- How to execute specific describe-blocks and it-blocks?
- How to disable specific describe-blocks and it-blocks?
- What is "beforeAll" and "afterAll" functions in Jasmine?
- What is "beforeEach" and "afterEach" functions in Jasmine?
- What is "expect" in Jasmine?
Note: We have already discussed jasmine installation, Please install if you have not done already.
Jasmine Test for Automating Angular JS application
Jasmine is a test framework, which provides BDD (Behavior Driven Development) functionalities for your automation framework. It is an independent framework i.e there is no dependency with other framework and doesn’t require DOM. A describe-block, it-block, and an expectation with matcher makes one complete test script.
"describe" block in Jasmine
A describe-block is like a test suite in Jasmine Test, it holds a set of test cases that are called "it". Any test scripts begin with a keyword describe, it’s a global function provided by jasmine. This takes two parameters string and function:
- The first parameter string is just the name of the test script.
- The second parameter is a function that consists of the whole bunch of code snippets or implementation of the test case.
Describe-Block acts as a container for it-blocks. Typically, a describe-block contains one or more it-blocks. Describe block can be considered as a test suite as it holds multiple test cases.
describe("Sample Test", () => {
//it block goes here
});
"it" block in Jasmine
It-block is placed inside the describe-block in Jasmine Test, one it-block is equivalent to one test case. Jasmine doesn’t restrict a number of it-blocks. There can be any number of it-blocks inside the describe-block. It is a global function in jasmine, Just like describe-block, it-block takes two parameters one is a string and the other is function.
- The first parameter string is just the name of the test case.
- The second parameter is the function which consists of the whole bunch of code snippets or implementation of the test case.
describe('A Sample Test', () => {
it('First Testcase', () => {
console.log('First Testcase');
});
it('Second Testcase', () => {
console.log('Second Testcase');
});
});
Note: It-Block is also known as a "spec" in Jasmine. Few people may call it a "test" as well.
"beforeEach" and "afterEach" function blocks in jasmine
As the name implies, the beforeEach function is called once before each spec/test/it-block in describe-block. And afterEach function also behaves the same as beforeEach function but it executed once after each it-block. Typically if anything needs to be executed before or after each test case those set of code will be placed here.
describe('Describe', () => {
beforeEach(()=> { //beforeEach function
Console.log('Before Each Called');
});
it('Sample 1', () => {
Console.log('First it block');
});
it('Sample 2', () => {
Console.log('Second It block');
});
afterEach( () =>{ //afterEach function
Console.log('After Each Called');
});
})
In the above example before and after each block will be called two times as there are two it-blocks.
"beforeAll" and "afterAll" function blocks in jasmine
The beforeAll function is called only once before all the spec in describe-block are run, and the afterAll function is called after all specs finish. These functions can be used to speed up test suites with expensive setup and teardown.
describe('Describe', () => {
beforeAll(() => { //beforeAll function
console.log('Before All Called');
});
it('Sample 1', () => {
console.log('First it block');
});
it('Sample 2', () => {
console.log('Second It block');
});
afterAll(() => { //afterAll function
console.log('After All Called');
});
})
Multiple independent describe-block in Jasmine Test
A spec file will have multiple describe-block however those are independent of each other. That means the outcome of one describe-block doesn't depend on others. Put the describe-block one after the other to create multiple describe-block as shown in the below example.
Consider you have a file called sample-spec.ts it can have below code:
describe('First Describe', () => {
it('Sample 1', () => {
console.log('Inside First describe');
});
});
describe('Second Describe', () => { //Second describe outside first
it('Sample 2', () => {
console.log('Inside second describe');
});
});
Executing test-spec.ts executes both the describe blocks.
Nested describe-block in Jasmine Test
Nesting is one inside the other, same is applicable for describe also. A describe-block can have other describe-block inside it. Consider below example there are two nested describe block inside the single spec file (ex: test-spec.ts)
describe('First Describe', () => {
it('Sample 1', () => {
console.log('Inside First describe');
});
describe('Second Nested Describe', () => { //Describe inside the describe
it('Sample 2', () => {
console.log('Inside second describe');
});
});
});
Executing test-spec.ts executes both the describe blocks.
Execute specific describe-block
Jasmine provides the functionality to the user, that one can execute specific test cases or test suites. The character "f" is prefixed with either describe-block or it-block. Prefixing "f" will make execution focus on only that block i.e executes only that test case.
fdescribe('First Describe', () => { //f is prefixed to desscribe
it('Sample 1', () => {
console.log('Inside First describe');
});
});
describe('Second Describe', () => {
it('Sample 2', () => {
console.log('Inside second describe');
});
});
In the above line of code, there are two describe block but the requirement is to execute only the first test case this can be achieved by prefixing the f.
Executing specific it-block
Jasmine provides the functionality to execute only specific spec, for example, if there are two it blocks inside describer and if there is a need to execute only one it-block, that can be done by prefixing ***f ***to it
describe('A Describe block', () => {
fit('Sample 1', () => { //f is prefixed to this it
console.log('First it block');
});
it('Sample 2', () => {
console.log('Second it block');
});
});
Disable test suite (describe-block) in Jasmine Test
Let's consider the scenario, where there are multiple describe-block. Our requirement is not to execute just one particular scenario out of hundreds. Basically, disabling that one scenario and this can be achieved by prefixing "x" to describe or it-block.
xdescribe('First Describe', () => { //x is prefixed to describe
it('Sample 1', () => {
console.log('Inside First describe');
}
);
});
describe('Second Describe', () => {
it('Sample 2', () => {
console.log('Inside second describe');
});
});
Take a look at the above example, since x is prefixed at first, describe-block will never get executed. Only second describe-block gets executed. x can be prefixed to any number of describe-block. Keep in mind that those test cases will never get executed until x prefix is removed.
Disable test cases (it-block) in Jasmine Test
Protractor provides the capability to disable test cases, i.e it-blocks. In order to disable the block just prefix it with x. But there is a noticeable difference between disabling the it-block and disabling the describe-block. Disabled describe-block will not be shown in results but disabled it-block will be shown as pending.
Below is the example which shows disabling it block.
describe('First Describe',()=>{
it('Sample 1',()=>{
console.log('Inside First it');
});
xit('Sample 2',()=>{ // x is prefixed to this it
console.log('Inside Second it');
});
});
Expectation and Matchers in Jasmine Test
Expectations are built with the function "expect" which takes a value, called the actual value. It is chained with a Matcher function, which takes the expected value.
Each matcher implements a boolean comparison between the actual value and the expected value. It is responsible for reporting to Jasmine if the expectation is true or false. Jasmine will then pass or fail the spec.
describe('Expectaion Demo', () => {
it('Expectaion Example', () => {
let a = 10;
let b = 20;
expect(a).toBe(b);
expect(a).toBeDefined();
});
});
Note: expect keyword is used to define the expectation in jasmine.
In the above example, there are two expect statements but both are associated with different matches.
- First matcher checks for equality that is whether a is equal to b or not. If yes then the test will be marked as pass else test will be marked as a fail.
- Similarly, the second matcher checks whether the value of a is defined or undefined.
Below are the most commonly used matchers in Jasmine.
Matcher | Example | Description |
---|---|---|
toBe(expected) | expect(a).toBe(b); | Checks a and b are equal ( similar to a===b) |
toBeFalsy() | expect(a).toBeFalsy() | Expects value of a is false (similar to expect(a).toBe(false) |
toBeTruthy() | expect(a).toBeTruthy() | Expects value of a is true (similar to expect(a).toBe(true) |
toEqual(expected) | expect(object1). toEqual (object2) | Expects object1 and object2 to be equal |
toContain(expected) | expect(array).toContain(anElement);expect(string).toContain(substring); | Expects the actual value to contain a specific value. |
toBeGreaterThan(expected) | expect(result).toBeGreaterThan(0); | Expects the actual value to be greater than the expected value. |
toBeGreaterThanOrEqual(expected) | expect(result).toBeGreaterThanOrEqual(1); | expect the actual value to be greater than or equal to the expected value. |
toBeLessThan(expected) | expect(result).toBeLessThan(0); | expect the actual value to be less than the expected value |
toBeLessThanOrEqual(expected) | expect(result).toBeLessThanOrEqual(123); | expect the actual value to be less than or equal to the expected value |
toBeNaN() | expect(thing).toBeNaN(); | expect the actual value to be NaN (Not a Number). |
toBeNegativeInfinity() | expect the actual value to be -Infinity (-infinity). Example | expect(thing).toBeNegativeInfinity(); |
toBeNull() | expect(result).toBeNull(); | expect the actual value to be null. |
toBePositiveInfinity() | expect(thing).toBePositiveInfinity(); | expect the actual value to be Infinity (infinity). |
toBeUndefined() | expect(result).toBeUndefined(): | expect the actual value to be undefined. |
toMatch(expected) | expect("my string").toMatch(/string$/); | expect the actual value to match a regular expression |
toThrow(expected) | expect(function() { return 'stuff'; }).toThrow();expect(function() { return 'things'; }).toThrow(‘toolsqa’); | expect a function to throw something. |
toThrowError(expectedopt, messageopt) | expect(function() { return 'things'; }).toThrowError(MyCustomError, 'message'); | expect a function to throw an Error. |
Note: Points to Remember
- Describe block holds one or more it blocks
- Multiple describe blocks can be nested or can be made independent in single file
- BeforeEach and AfterEach block can be used to
- execute a specific set of code before or after every test cases respectively.
- BeforeAll and AfterAll block can be used to set up asks that execute once per test suite or describe block
- Any test suite or test case can be executed specifically without executing all, prefix with f to describe or it block
- Any test suite or test case can be disabled by prefixing with x (ex: xit, xdescribe)
- It function without a body will not be executed and results will be marked as pending.
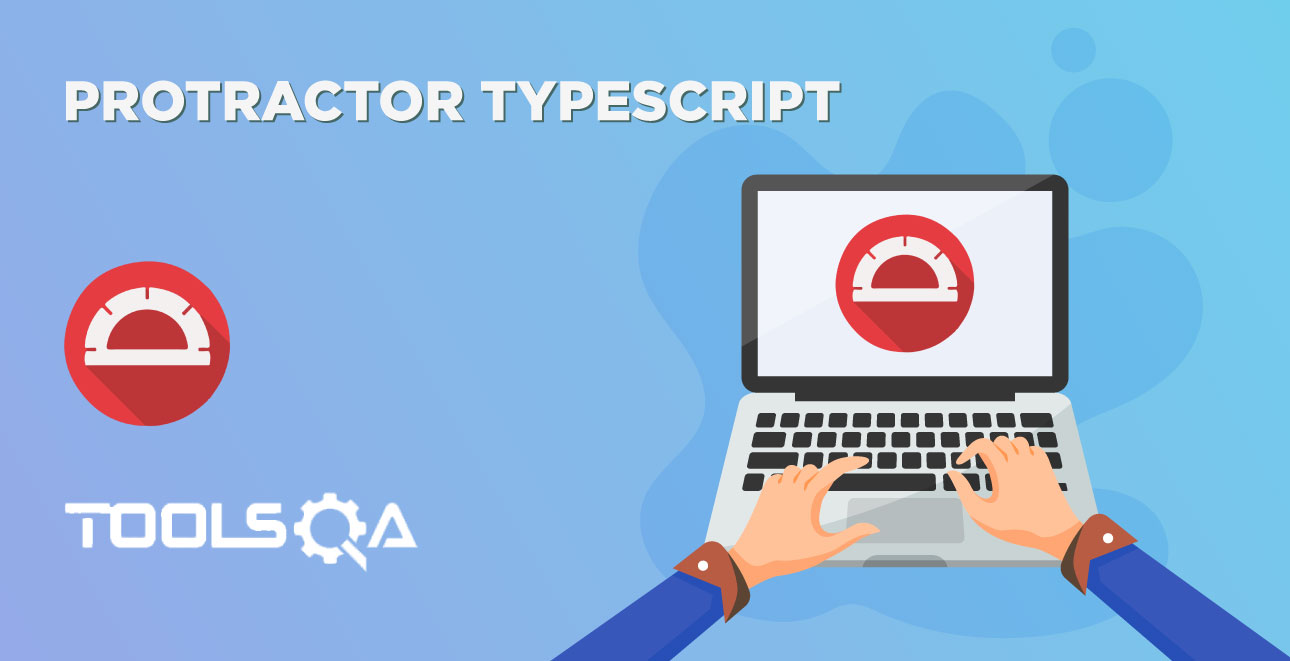
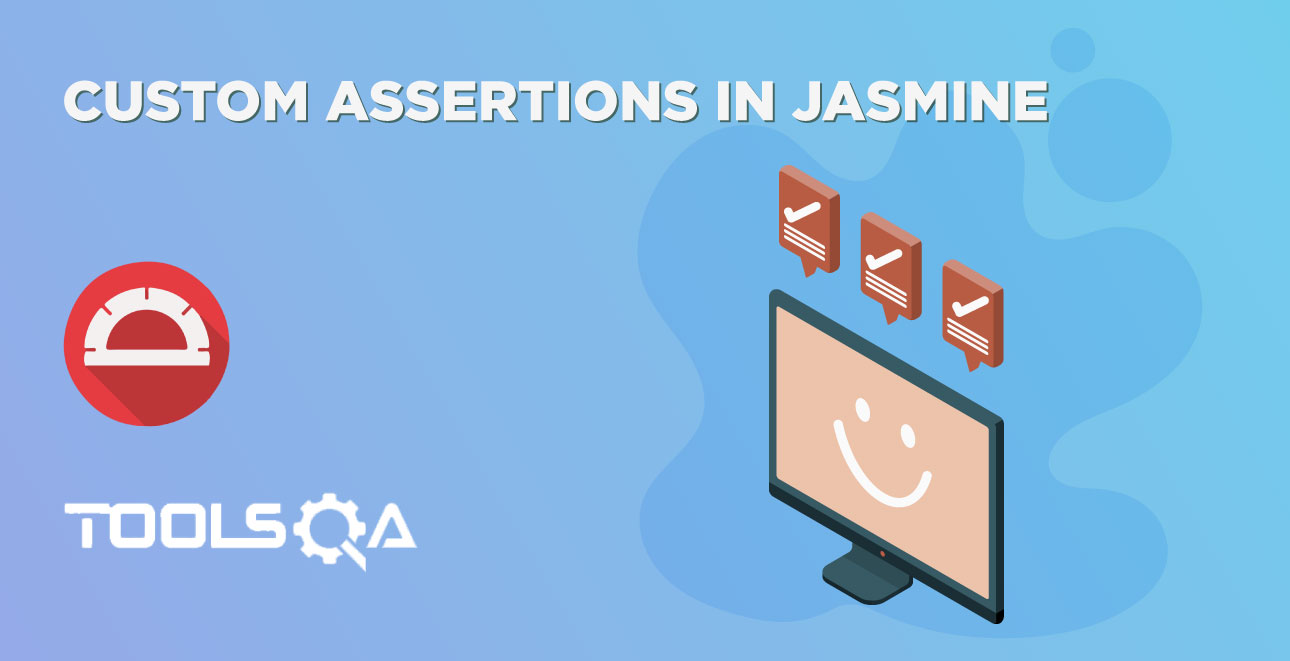