In the previous chapters, we discussed Protractor Browser Commands and Windows Command. In this, we will take a look at Protractor Browser Navigation Commands. Navigation commands in Protractor provide four methods to navigate. Let us discuss one by one in this article
- The "back" method in Protractor
- The "forward" method in Protractor
- The "refresh" method in Protractor
- The "to" method in Protractor
Protractor Browser Navigation Commands
Navigation methods help to browse different pages. With the help of the browser navigation method, one can simulate the user's browser navigation behavior using a protractor. The browser.navigate() has 4 methods, all the methods belong to class Navigation.
Protractor browser navigation commands - The "back" method
Purpose: The protractor command back() is used to move backward in the browser history.
Syntax: browser.navigate().back(): promise.Promise<void>
Returns: This command returns a promise that will be resolved when the navigation event has completed.
Code Example:
//spec.ts
import {browser} from 'protractor';
describe('Browser Navigation', () => {
it('Exampe of back method', () => {
browser.get('https://material.angularjs.org')
.then(()=>(browser.get('https://www.protractortest.org/#/faq')))
.then(()=>(browser.sleep(5000)))
.then(()=>(browser.navigate().back())) //Back method used here
.then(()=>(browser.sleep(10000)));
});
});
Note: This command works only if the following condition met: Browser back button is enabled and this will happen only if at least one previous page browsed.
Protractor browser navigation commands - The "forward" method
Purpose: The protractor command forward() is used to move forward in the browser history.
Syntax: browser.navigate().forward(): promise.Promise<void>
Returns: This command returns a promise that will be resolved when the navigation event has completed.
Code Example:
//spec.ts
import {browser} from 'protractor';
describe('Browser Navigation', () => {
it('Exampe of forward method', () => {
browser.get('https://material.angularjs.org')
.then(()=>(browser.get('https://www.protractortest.org/#/faq')))
.then(()=>(browser.sleep(5000)))
.then(()=>(browser.navigate().back()))
.then(()=>(browser.navigate().forward())) //Forward method used here
.then(()=>(browser.sleep(5000)));
});
});
Note: This command works only if the User is navigated back from the previous screen and the browser navigation button is enabled.
Protractor navigation commands - The "refresh" method
Purpose: The protractor command refresh() is used to refresh the current page.
Syntax: browser.navigate().refresh(): promise.Promise<void>
Returns: This command returns a promise that will be resolved when the navigation event has completed.
Code Example:
//spec.ts
import {browser} from 'protractor';
describe('Browser Navigation', () => {
it('Exampe of refresh method', () => {
browser.get('https://material.angularjs.org')
.then(()=>(browser.sleep(5000)))
.then(()=>(browser.navigate().refresh()))
.then(()=>(browser.sleep(5000)));
});
});
Protractor - The "to" method
Purpose: The to() method is used to navigate to a new URL.
Syntax: browser.navigate().to(url:string): promise.Promise<void>
Parameter: This command accepts one parameter i.e URL to be navigated. Basically, this URL is a string.
Returns: This command returns a promise that will be resolved as URL as loaded.
Code Example:
import {browser} from 'protractor';
describe('Browser Navigation', () => {
it('Exampe of to method', () => {
browser.get('https://material.angularjs.org')
.then(()=>(browser.sleep(5000))) //Sleep is added to pause the execution for sometime
.then(()=>(browser.navigate().to("https://protractortest.org/#/style-guide"))) // to() method is used here
.then(()=>(browser.sleep(5000)));
});
});
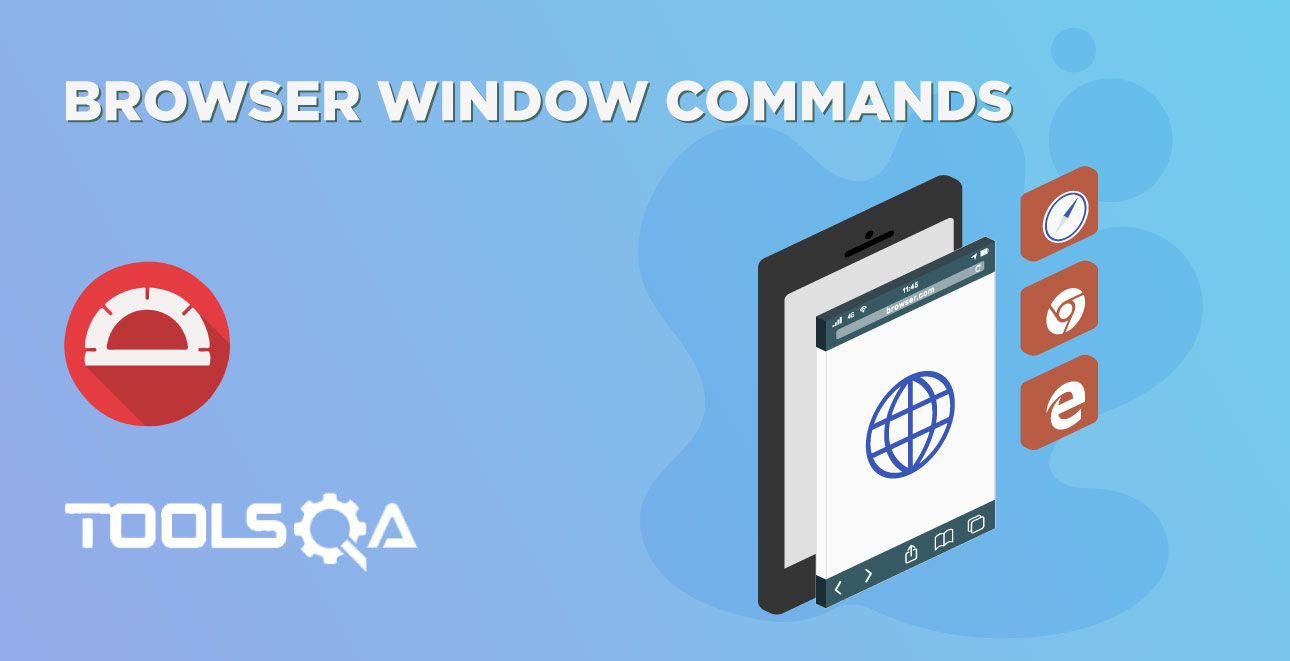
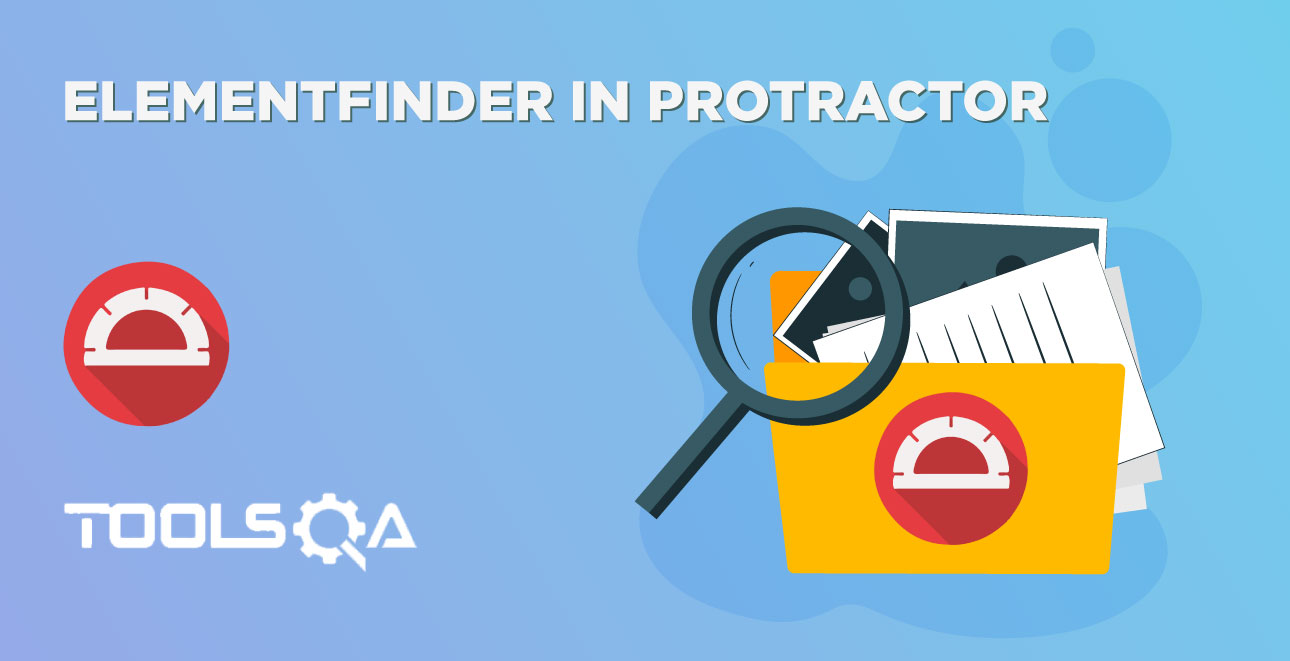