In this course, while exploring the python bitwise operators, python boolean operators and python comparison operators, you must have noticed one thing: the conditional statements. In the examples in which we dealt with these operators, the operators were absorbed inside "if ", "else-if " and other conditional statements in python. These are called conditional statements because they represent the conditions similar to the diamond box in the flowchart depiction. This post will explore various conditional statements in Python. Additionally, we will understand their usage, examples, and comparisons with other languages if any. The table of contents is as follows:
- What is a Conditional Statement in Python?
- If Conditional Statement in Python.
- Else Statement in Python.
- Elif Conditional Statement in Python.
- Python Cascaded If Statements
- Switch Case in Python.
- Python Pass Statement.
What is a conditional statement in Python?
A conditional statement in python, also called a condition constructs, is a statement that accommodates a condition inside itself. This condition is constructed using the bitwise, boolean, and comparison operators in Python. We already talked in great detail about it in previous posts. A conditional statement always generates a boolean output that is either true or false. (Note that true is represented as True in Python and false as False). After generating the output, the statement decides whether to move inside the conditional block or leave it as it is. The code inside the conditional block is executed if and only if the conditional statement returns true. (from expressions inside the construct)
The next sections will clear the doubts on conditional statements in Python. We will start our learnings on conditional statements with the most used conditional statement not only in Python but in any language i.e. the if statement.
If Conditional Statement in Python
The simplest and most used conditional statement in Python is the "if " statement. A lot of the times, you have to decide whether to do "A " or do "B ". The "if " statement, as its name suggests evaluates the expression. Moreover, it executes the conditional block only when the statement is true. You can remember the statement as:
if (something is True)
//execute the statements
So, for example, I have a variable age whose value is 40. Then, with the given below code, the print statement will be executed only if the age is 40.
age = 40
if(age == 40):
print("The age is 40")
To refresh your memories, recall that Python does not use braces to represent the code blocks and uses colon instead. All the code intended to be inside the conditional statement should b indented properly and in alignment. Misalignment of even one space can lead to exceptions and errors.
Executing the above code will return the following output:
Great! This was what we expected. Now the next step is, what if the condition turns out to be False and we then want to print something *(or anything else)? *This comes out as the "else " conditional statement in Python.
Else Statement In Python
Else conditional statement is a simple response to the "if " conditional statement such as if this does not happen what else would? Therefore, whenever the "if " statement returns False and conditional block from "if " skips, the conditional block from "else " executes.
Continuing from the previous example, now my age is 35 and I have an "if " statement which prints the age. If my age is 40, it will print otherwise another print statement will execute, telling that my age is not 40.
age = 35
if(age == 40):
print("The age is 40")
else:
print("Age is not 40")
The above execution would return the following results:
Alright! We now know how to execute some code based on certain conditions. In other words, if the condition is true (execute if block) otherwise execute else block if the condition is false. But there is one more use case in the conditional statements. What if we want to put up one more check once the "if " condition fails and not execute the else statement directly?
Elif Conditional Statement in Python
Elif is a rather unique term as other programming languages don’t use it. Other programming languages use "else if " to put up one more condition. While Python combines "else and if " and makes it an "elif " conditional statement. The usage of the "elif " statement will get more clear through the following given code.
Continuing on the same example, we will try to put up one more check in case the "if " conditional statement fails. Guess the output for the following code:
age = 35
if(age == 40):
print("The age is 40")
elif(age == 35):
print("Age is 35")
You are right if you guessed the following output:
Since the indentation in Python is similar to the "{ " in other programming languages, we can skip the indentation if there is only a single statement that we have to execute. The same practice we follow in other languages too! So, writing the "if " statement as follows:
if(condition): print("If statement Executed")
is equivalent to the following:
if(condition):
print("If statement Ecxecuted")
In addition to this, you can also combine various "if-elif-else " statements giving rise to a cascading conditional block which we will discuss in the next section.
Python Cascaded If Statements
The term "cascade " means a waterfall-like structure where we follow one step after the other. To make use of cascade statements in Python, we make use of "if-elif-else " statements that we have discussed above in great detail.
if(condition):
#code
elif(condition):
#code
elif(condition):
#code
else:
#code
Note: In the above statement, I have used two "elif" statements. Practically, you can use as many as you want although we always recommend lesser numbers.
Python cascaded statements are a good way to build up your logic in minimum lines keeping in mind the consistency of the structure maintains. The "if " block works as an "entry " gate of the complete conditional block in Python. The "if " block will always test first when we run the condition block. If the condition is satisfied, the code within "if " is executed and we exit out of the conditional block. If the condition does not satisfy, we move ahead to the first "elif " block and the same process follows till the end.
Therefore, only one of the code block executes, when any of the "if-elif" statement satisfies, we execute the code and skip the rest of the conditional block.
The else block is the last block that will execute when no other statement satisfies. This is totally optional and if your code does not require the execution of a statement necessarily, you don't need to put up an else statement.
The following code snippet prints the range of the age of the user. Try to understand the code with respect to the cascading conditional statements (conditional statement inside another conditional statement).
age = 35
if(age > 60):
print("Senior Citizen Benefits Applicable")
elif(age > 21):
if(age > 40):
print("Benefits Renewed Automatically")
else:
print("Your Benefits Have Lapsed, Please Renew")
else:
if(age > 13):
print("Benefits Covered Under Parental Scheme")
else:
print("Benefits Covered Under Government Childcare Scheme")
Run the above code and verify your guessed output with the console output. Always remember that the indentation of the statements should be proper otherwise Python would mix up the structure and the output would not be as expected. Also, try to change the variable values and see what output generates. Feel free to extend the code and share it with us for other learners.
Switch Case In Python
If you are coming from a C++ or Java background like myself, this statement is going to look a bit odd. Python does not have a switch statement. If Python is your first language and you have no idea about the switch statement, you can learn more about the conventional switch case statement, by visiting the Switch Case Implementation. I know it feels odd because even though Python has been around for a long while now, "switch case in Python " is still a popular search term on Google. So, if Python does not have a switch statement, what do we have for replacement?
The intuitive way that comes to the mind is to use a series of "if-elif-else " statements and implement the logic. But "if-elif-else " is prone to errors since more conditions lead to more complex nested statements. If you are comfortable, you can achieve the same using the "if-elif-else " statements.
Another way is to go for the dictionary switcher method. In this, we can create a function with a dictionary called switcher. Moreover, we can construct the dictionary using the key and value pairs just like a case statement in the conventional switch method. The explanation of the same is there using the code below:
def season(i):
switcher={
0:'Winter',
1:'Summer',
2:'Spring',
3:'Autumn',
4:'Monsoon',
5:'Fall',
}
return switcher.get(i,"Invalid Season")
print(season(2))
Just like case (i ), we can put the function name in place of the case. With the same intentions, we will get the following output:
And hence, you can use the switch statement with some minor tweaks. This was just to widen your knowledge spectrum in Python and is not a part of the PCEP certification course.
Python Pass Statement
The final section for our conditional statements in Python is the pass statement. Just like the switch statement in Python, the pass statement is also specific to it. Other programming languages don’t use it.
The Pass statement in python resolves the problem of an empty code block or a stub code. In programming, we often leave some functionality blocks empty without any implementation. Because, at that point, we are not sure what would come in those blocks. Since other programming languages use "{ " or such delimiters to determine the start and end of a code block under conditional statements. Python does not have this construct.
With predefined delimiters, we can just leave the code part and we should understand it implicitly. Doing the same results in an error in Python. For example, in the below code I have left the if conditional block without any implementation:
age = 40
if(age == 40):
print("If Statement Executed")
Executing the above code gives the following error:
This error means that Python needs something inside that indented if conditional statement and it cannot be left empty. To resolve this issue, we use the "pass " keyword. Using the "pass " keyword is equivalent to leaving the code blank. Additionally, it symbolizes that from the point of "pass " till the end of the indented block, we don't want any executions. Let's put "pass " in our code as follows:
age = 40
if(age == 40):
pass
print("If Statement Executed")
Execute the above code and verify whether the error resolves or not.
As expected, the conditional code block skipped and the next statement executed. With this, we conclude this post on the conditional statements in Python. Apart from the topics discussed, there are no other questions that will trouble you either in PCEP or programming in Python. You can refer to the below given key highlights to revise the content of this post.
Key Takeaways:
- A conditional statement in Python also called a control statement or conditional construct. It is a statement that encapsulates the conditional expressions and evaluates the result in terms of True or False.
- Below are the types of conditional statements in Python:
- If conditional statement.
- Elif conditional statement.
- Else conditional statement.
- There is no switch conditional statement in Python and hence we create a switch statement using the dictionary.
- Python does not allow to skip an empty block of code. Therefore, in such situations, we use the "pass" keyword.
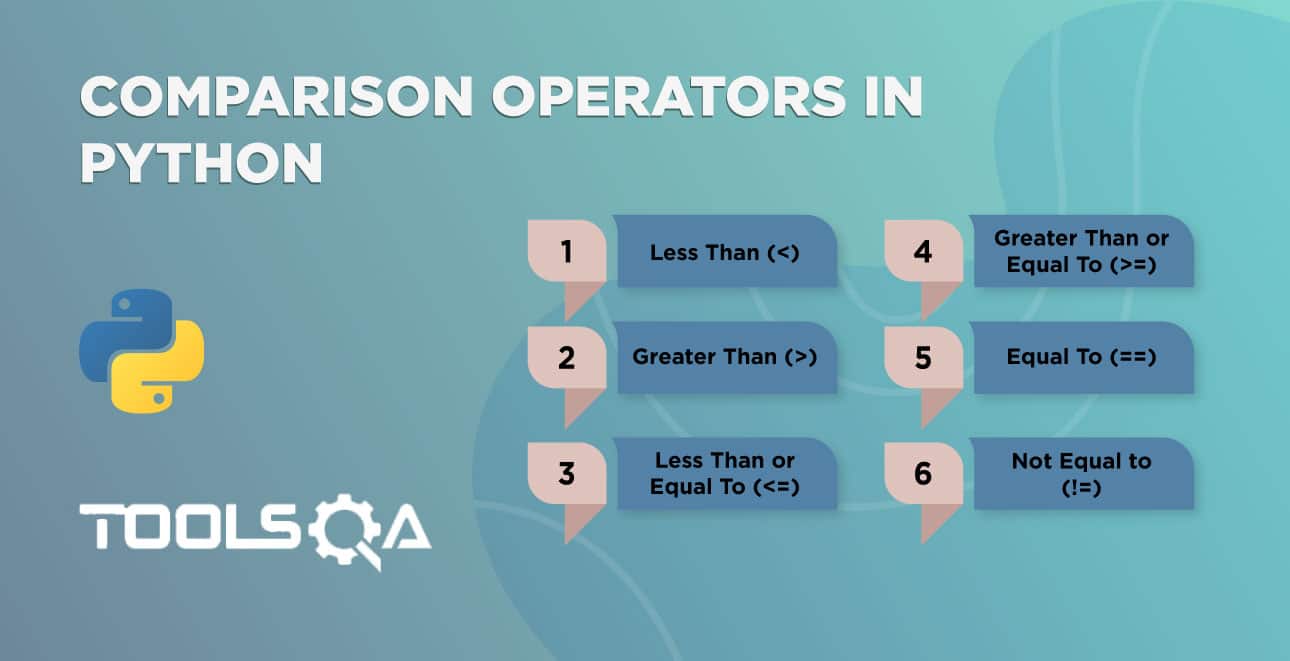
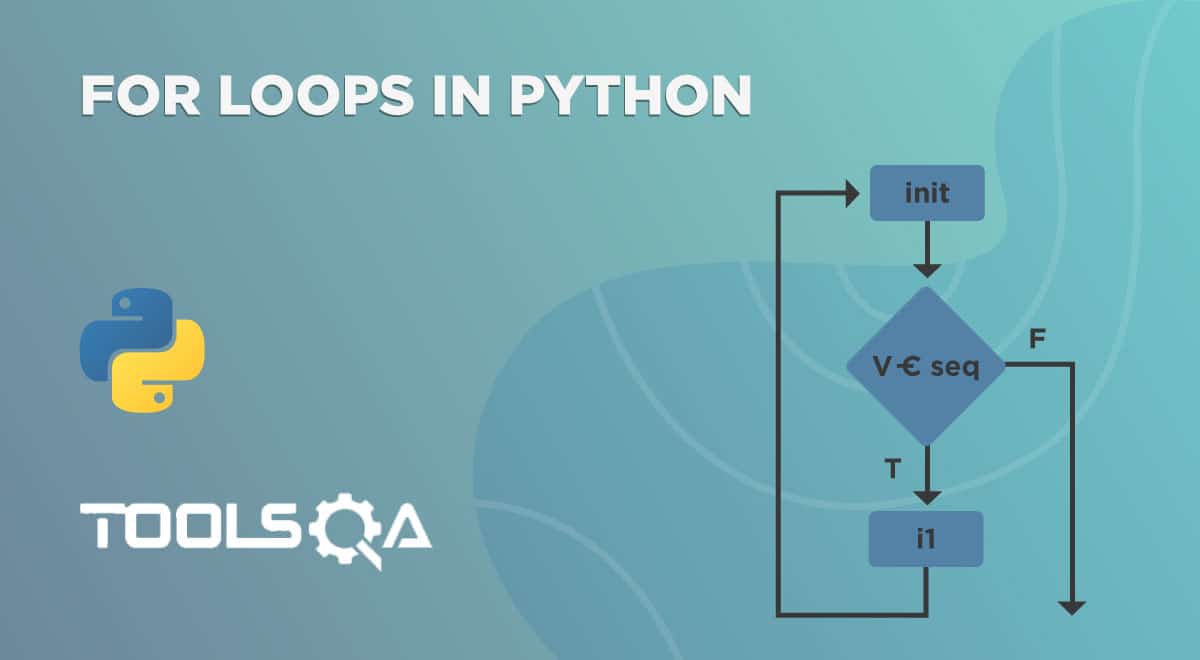