Decision-making is one of the critical facilities which a programming language has to provide. To understand Java better we have to understand this chapter and practice by writing some decision-making code.
Decision making in java
There are two types of decision-making statements in Java. One is very commonly used which is If statement and you will find it almost in every piece of code. The second is Switch statement.
Java if...else statement
We use the if statement to control the flow of the program. For instance, if a certain condition is met, then run a specific block of code. Otherwise, run another code.
Here are the various forms of if...else statements in Java:-
- if statement
- if...else statement
- if...else if...else statement
Java if (If-then) Statement
The if-then statement is a control flow statement. Which actually specifies that the block of code is only executed when the test specified by the if part evaluated to be true. The if statement executed from top to down. As soon as one of the conditions is true, a statement associated with that if statement executed. Keyword then is not used in Java and it is denoted as {}. The syntax for the if-then statement is:
if(boolean-expression){
statement; //This part of code will only execute when the condition returns true
}
Example
package javaTutorials;
public class IfThen_Statement {
public static void main(String[] args) {
String sDay = "Sunday";
int iDay = 7;
if(sDay.equals("Sunday")){
System.out.println("Today is Sunday");
}
if(iDay==7){
System.out.println("Today is Sunday");
}
}
}
Output
Today is Sunday
Today is Sunday
Java If...else (if-then-else) Statement
If the statement can be executed in different ways, such as if-then-else & if-else_if-else. The optional else block is executed when the if part evaluates to false. The syntax for the if-then-else statement is:
if (boolean-expression) {
// Execute if boolean-expression is true
} else {
// Execute if boolean-expression is false
}
Example
package javaTutorials;
public class IfThenElse_Statement {
public static void main(String[] args) {
String sDay = "Saturday";
int iDay = 6;
if(sDay.equals("Sunday")){
System.out.println("Today is Sunday");
}else{
System.out.println("Today is not Sunday");
}
if(iDay==7){
System.out.println("Today is Sunday");
}else{
System.out.println("Today is not Sunday");
}
}
}
Output
Today is not Sunday
Today is not Sunday
Java if..else if..else Statement
Like this, we can handle multiple conditions as well with the help of if-else_if-else statements. The syntax is:
if (boolean-expression) {
// Execute if boolean-expression one is true
} else if (boolean-expression2) {
// Execute if boolean-expression two is true
} else {
// Execute if boolean-expression is false
}
Example
package javaTutorials;
public class IfThenElseIf_Statement {
public static void main(String[] args) {
String sDay = "Monday";
int iDay = 1;
if(sDay.equals("Sunday")){
System.out.println("Today is Sunday");
}else if(sDay.equals("Saturday")){
System.out.println("Today is not Saturday");
}else{
System.out.println("Today is a Weekday");
}
if(iDay==7){
System.out.println("Today is Sunday");
}else if(iDay==6){
System.out.println("Today is Saturday");
}else{
System.out.println("Today is a Weekday");
}
}
}
Output
Today is a Weekday
Today is a Weekday
Note: The else if part is optional but it can appear many times between the if and else parts. The else part is optional but it can appear only once after if and all else if parts. If none of the conditions is true then else statement will be executed, only one of the statements executed from the list of else if statements.
The switch Statement
Switch statement provides an easy way to dispatch execution to different parts of your code based on the value of an expression. It is used to replace the multilevel if-else-if statement. But the switch statement can only be used if the conditions are based on the same constant value. The syntax for the switch statement is :
switch(expression){
case value1:
//Execute if the variable is matched with the case (value1)
break;
case value2:
//Execute if the variable is matched with the case (value2)
break;
default:
//Execute if the variable is not matched with the any case
break;
}
There are few rules which apply to switch statement:
- The "expression" must be of type byte, short, int, or char.
- A switch statement can have an optional default case, which must appear at the end of the switch. The default case can be used for performing a task when none of the cases is true. No break is needed in the default case.
- Each case must end with colon (:)
- Each case value must be a unique literal(constant not a variable). Duplicate case value not allowed.
- Typically each case has a trailing break and a break acts as an exit point.
Example
package javaTutorials;
public class Switch_Statement {
public static void main(String[] args) {
int iDay = 1;
String sDay = "Monday";
switch(iDay){
case 1:
System.out.println("Today is Monday");
break;
case 3:
System.out.println("Today is Tuesday");
break;
case 4:
System.out.println("Today is Wednesday");
break;
case 5:
System.out.println("Today is Thursday");
break;
case 6:
System.out.println("Today is Friday");
break;
case 7:
System.out.println("Today is Saturday");
break;
default:
System.out.println("Today is Sunday");
break;
}
switch(sDay){
case "Monday":
System.out.println("Today is Monday");
break;
case "Tuesday":
System.out.println("Today is Tuesday");
break;
case "Wednesday":
System.out.println("Today is Wednesday");
break;
case "Thursday":
System.out.println("Today is Thursday");
break;
case "Friday":
System.out.println("Today is Friday");
break;
case "Saturday":
System.out.println("Today is Saturday");
break;
default:
System.out.println("Today is Sunday");
break;
}
}
}
Output
Today is Monday
Today is Monday
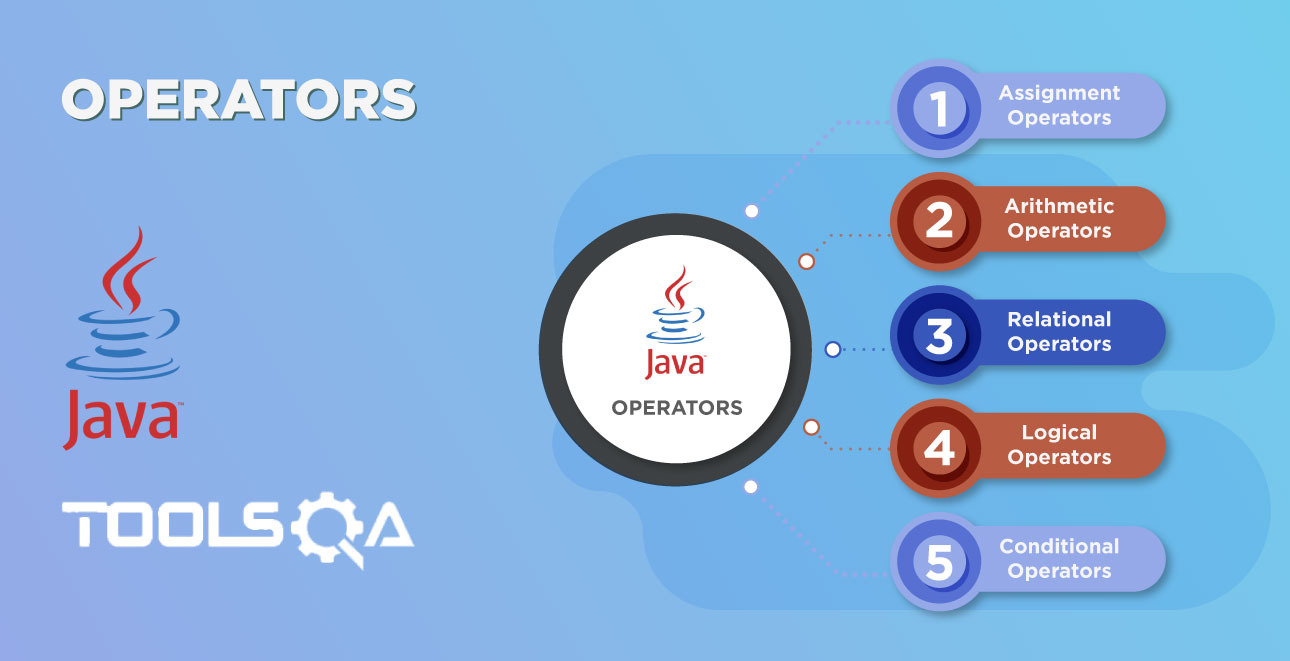
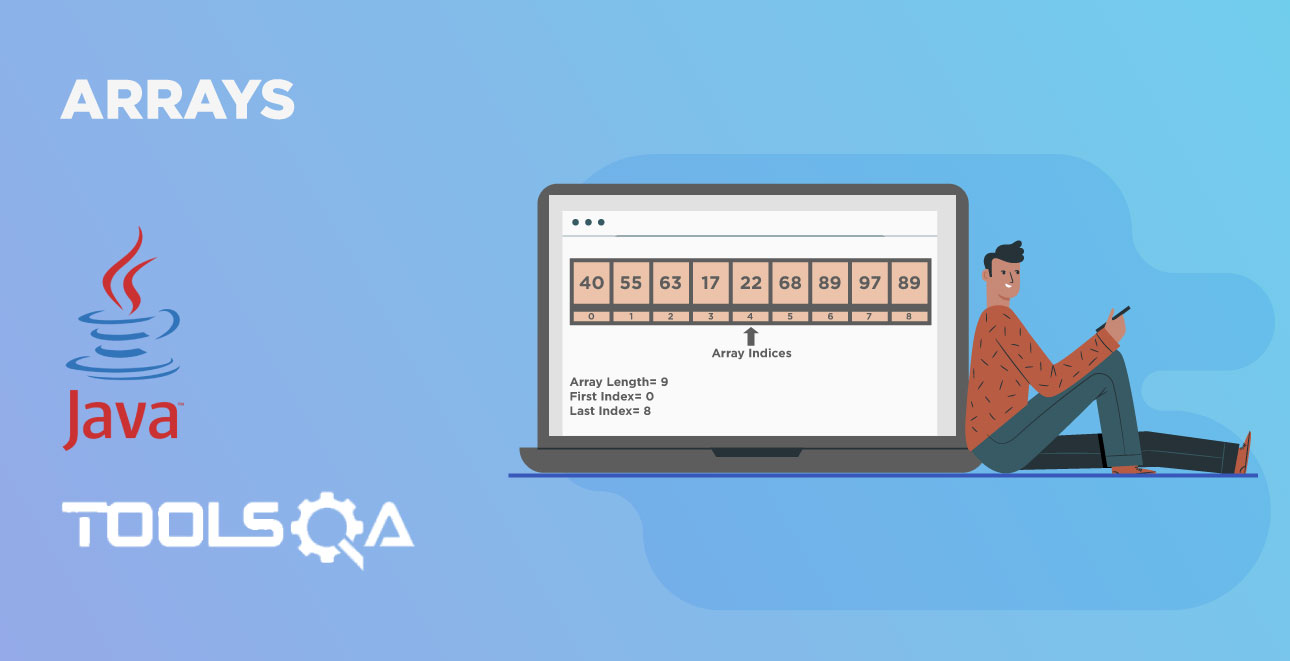