The HashTable Class
The HashTable class extends the Dictionary abstract class to implement a hash table. In the table, keys are segmented into buckets based on their hash code. Thus, a key must expose the methods hashCode() (for bucket segment) and equals (to compare the two keys in the same bucket). For example, String has both method hashCode and equals, so it can be used as key type in a HashTable.
Let us declare a HashTable that maps a key of type String to a value of type String
Hashtable<String, String> contacts = new Hashtable<String, String>();
In the following examples, the contacts variable is the data structure that we use to associate a person's name and his or her email address. Let us write methods to carry out common tasks involving the contacts.
Method: containsKey()
To know whether a name exists in the contact book, we use containsKey method
public boolean hasName(String name) {
return contacts.containsKey(name);
}
Method: containsValue()
To know whether an email address was already added in the contact book, we use containsValue method, which traverses the value collections of the Hashtable and compares the values.
public boolean hasEmailAddress(String emailAddress) {
return contacts.containsValue(emailAddress);
}
Method: get()
To find the email address of a person given his or her name, we use the get method.
public String findEmailAddress(String name) {
return contacts.get(name);
}
Method: put()
To add a new contact, first, we check whether the email address exists or the name exists. If not, a new contact is added using the put method.
public int addContact(String name, String emailAddress) {
if (hasEmailAddress(emailAddress) || hasName(name)) {
return 0;
} else {
contacts.put(name, emailAddress);
return 1;
}
}
Method: remove()
To delete a contact, first, we check whether the contact exists. If it does exist, the contact is deleted using the remove method.
public int deleteContact(String name) {
if (hasName(name)) {
contacts.remove(name);
return 1;
} else {
return 0;
}
}
Method: keySet()
To list all contacts in the book requires a little more effort. First, we get the Set of all names in the contact list via keySet() method. Traversing the name Set, we find their associated email addresses via using the get method. For simplicity, we use the enhanced `for:loop to traverse the key set.
// Get the name Set
for (String name : contacts.keySet()) {
String emailAddress = contacts.get(name);
System.out.printf("%-10s|%-20s\n", name, emailAddress);
}
Complete Hashtable example code:
To conclude this article, the following ContactBook class, and ContactBookTest class demonstrates all the above usage of a HashTable.
// File: ContactBook.java
import java.util.Hashtable;
class ContactBook {
Hashtable<String, String> contacts;
ContactBook() {
contacts = new Hashtable<String, String>();
}
/*
* Has the contact added to the phone book
* @param: String name
* @return: true if the contact was added, false otherwise
*/
public boolean hasName(String name) {
return contacts.containsKey(name);
}
/* Find a contact by email address
* @param: String emailAddress
* @return: true if the contact with the email address was added, or false if not
*/
public boolean hasEmailAddress(String emailAddress) {
return contacts.containsValue(emailAddress);
}
/*
* Find a contact
* @param: String name
* @return: the contact with the above name, or null if not found
*/
public String findEmailAddress(String name) {
String emailAddress = contacts.get(name);
if (emailAddress != null) {
System.out.println("[" + name + "]: [" + emailAddress + "]");
return emailAddress;
}else {
System.out.println("Contact for [" + name + "] not found");
return null;
}
}
/*
* Add a new contact
* @param: String name, String emailAddress
* @return: 1 if a new contact is created, 0 otherwise
*/
public int addContact(String name, String emailAddress) {
if (hasEmailAddress(emailAddress)) {
System.out.println("Cannot add [" + name + "] Email address [" + emailAddress+ "] existed");
return 0;
} else if (hasName(name)) {
System.out.println("Name [" + name + "] existed");
return 0;
} else {
contacts.put(name, emailAddress);
System.out.println("A new contact for [" + name + "] added");
return 1;
}
}
/*
* Delete a contact
* @param: String name
* @return: 1 if one contact was deleted, 0 otherwise
*/
public int deleteContact(String name) {
if (hasName(name)) {
contacts.remove(name);
System.out.println("Contact for [" + name + "] removed");
return 1;
} else {
System.out.println("Contact for [" + name + "] not found");
return 0;
}
}
/*
* Print all contacts
*/
public void listAllContacts() {
System.out.printf("> Current contact list\n");
System.out.printf("%-10s|%-20s\n", "Name", "Email Address");
for (String name : contacts.keySet()) {
String emailAddress = contacts.get(name);
System.out.printf("%-10s|%-20s\n", name, emailAddress);
}
}
}
ContactBookTest class
// File ContactBookTest.java
public class ContactBookTest {
public static void main(String[] args) {
ContactBook book = new ContactBook();
book.addContact("Alice", "[email protected]");
book.addContact("Bob", "[email protected]");
book.addContact("Cathrine", "[email protected]");
book.addContact("Jane", "[email protected]");
System.out.println("> Find contact");
book.findEmailAddress("Alice");
book.findEmailAddress("Jane");
book.listAllContacts();
System.out.println("> Remove contact");
book.deleteContact("Alice");
book.listAllContacts();
}
}
The above code produces:
A new contact for [Alice] added
A new contact for [Bob] added
A new contact for [Cathrine] added
Cannot add [Jane] Email address [[email protected]] existed
> Find contact
[Alice]: [[email protected]]
Contact for [Jane] not found
> Current contact list
Name | Email Address
Bob | [email protected]
Cathrine | [email protected]
Alice | [email protected]
> Remove contact
Contact for [Alice] removed
> Current contact list
Name | Email Address
Bob | [email protected]
Cathrine | [email protected]
Summary
- The Dictionary class is an abstract class that associates keys with values.
- The HashTable extend the Dictionary class to implement the abstract methods including get, put, remove, containsKey and containsValue.
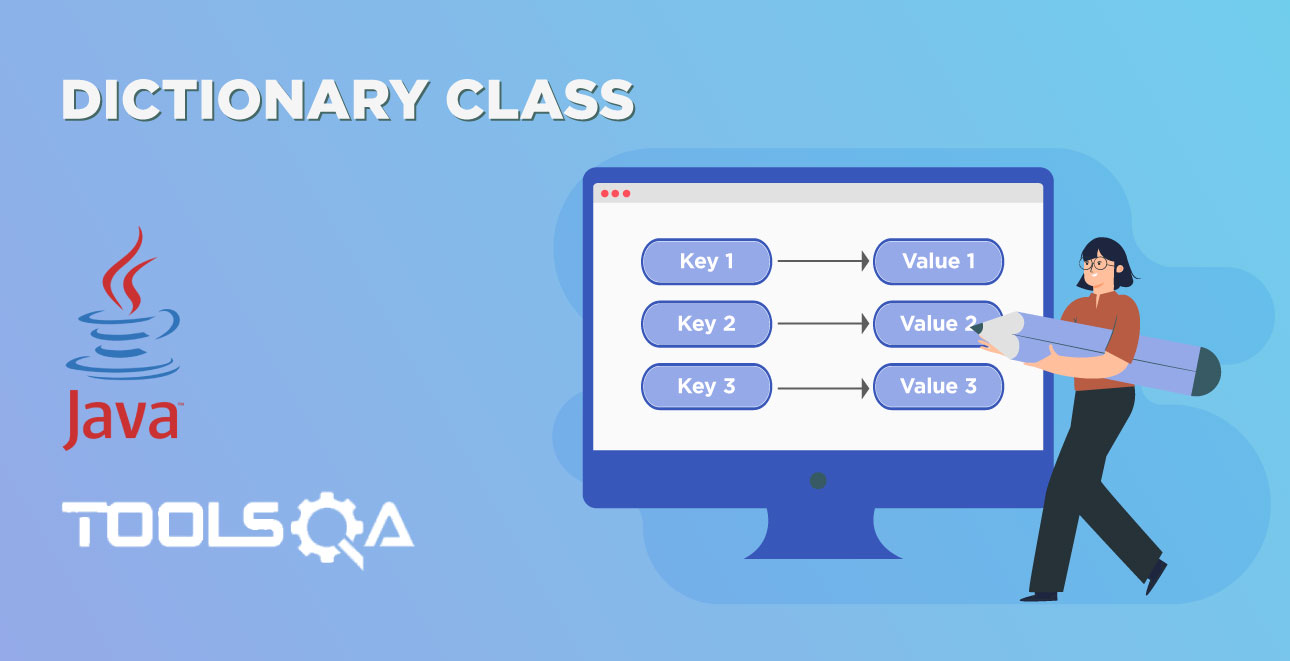
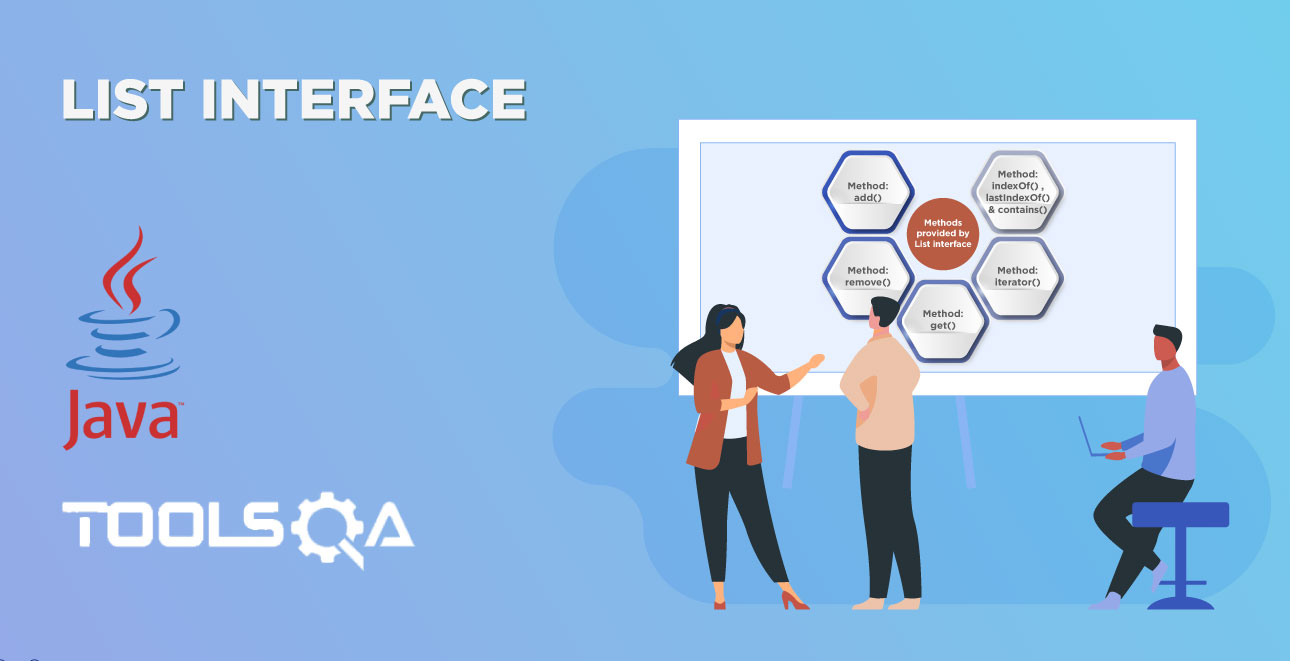