During the test development in SoapUI, there will be specific scenarios when the test assertions using the SoapUI are not sufficient enough for all the validations. Therefore, to handle such situations, SoapUI provides the capabilities to implement the validations using Groovy Scripts. These validations are known as "Script Assertions in SoapUI". In this article, we will deep dive into understanding the implementation of the Script Assertions in SoapUI for SOAP services by covering the details under the following topics:
- What are script assertions in SoapUI?
- How to add script assertions in SoapUI?
- How to check the existence of a string in the SOAP response?
- Also, how to validate that a string is not present in the SOAP response?
- How to validate the value of a node in the SOAP response?
- Also, how to validate the headers of the SOAP response?
- How to validate the response time of the SOAP response?
- How to validate the values for a range in the SOAP response?
What are Script Assertions in SoapUI?
Script assertions in SoapUI are one of the most used techniques of assertions, which helps implementation and managing of assertions very easy. It gives the programming control to the test developers, which in turn makes them more capable of controlling and validate the tests flows programmatically. Moreover, SoapUI majorly uses "Groovy Script" for the implementation of the scripted assertions, but also provides support for "JavaScript". Implementing the assertions with scripts provides following extended capabilities to a test developer:
Capability | Description |
---|---|
Pre and Post Operations | Scripting gives the freedom to perform certain operations before and after the TestCase. |
Handle Dynamic Response | Scripting provides the capability to handle and validate responses of APIs, which changes dynamically. |
Custom Assertions | Using scripts, you can create custom assertions specific to your project and use them on a need basis. |
Note: As mentioned above, in this article, we will be covering the implementation details of the Script assertions in SoapUI for the SOAP services only, and for that, we will be using ToolsQA BookStore WSDL. Additionally, for creating a new SOAP project, you can refer to the article SoapUI Projects.
Let's now see, how we can implement various script assertions on the response of the SOAP web service:
How to add Script Assertions in SoapUI?
To add script assertions simply go to the SOAP request on which we want to add assertion and click on '+' icon on the top left corner of the request window as shown below:
Let's understand the steps as highlighted above:
- Firstly, go to your SOAP request and double click on the step to open the request.
- Secondly, click on the '+' icon on the top left or the bottom left of the request window. You can see it highlighted in the snippet above.
- Finally, select Script assertion and click on 'Add'.
Now let's see a few types of assertions on the SOAP service response, which we can implement with the help of Script assertions in SoapUI:
How to check the existence of a string in the SOAP response?
Suppose we need to validate that the response of BookStore SOAP Service, contains a book with the author name "Richard E. Silverman". Let's validate the same with the help of Script assertions.
We can use the following code snippet for the validation on the service response:
import com.eviware.soapui.support.XmlHolder
def holder = new XmlHolder( messageExchange.responseContentAsXml )
def responseXml = holder.getPrettyXml()
assert responseXml.contains('Richard E. Silverman')
Let's understand the above code snippet in detail:
-
The first line imports the required library XmlHolder, which parses XML in SoapUI.
-
We then initialize an XmlHolder object and pass the response of the service as a parameter to it as shown below:
def holder = new XmlHolder( messageExchange.responseContentAsXml )
messageExchange.responseContentAsXml returns the response of the SOAP call in XML format.
3. Next, we need to convert the XML response to the string, and we can achieve the same can be achieved with the help of getPrettyXml() as shown below:
def responseXml = holder.getPrettyXml()
4. Then we can use the Groovy Script's assert method to check for existence if the string value in the response.
assert responseXml.contains('Richard E. Silverman')
We can evaluate the 'assert' expression as a boolean expression. If the value of the expression returns false, then the script assertion fails and vice-versa.
The below figure shows a sample placement and execution of above Script assertion in SoapUI window:
As we can see, the script gets added in the script area highlighted by step 2, and we can execute it using the play icon (highlighted by step 3). We can see that script passed the assertion as highlighted by step marked as 1.
How to validate that a string is not present in the SOAP response?
Next, if we want to validate that a particular string should not be part of the response of the target service, we can use the "not contains" assertion to evaluate the same. The not contains assertion, as the name suggests precisely opposite of contains assertion. It checks the absence of a specific string.
Suppose we need to validate that the response of BookStore SOAP Service contains a book with the author name "Mark Twain". Let's validate the same with the help of Script assertions.
We can use the following code snippet for the validation on the service response:
import com.eviware.soapui.support.XmlHolder
def holder = new XmlHolder( messageExchange.responseContentAsXml )
def responseXml = holder.getPrettyXml()
assert !responseXml.contains('Mark Twain')
As we can see, the above code also looks for the string 'Mark Twain' using the contains the method of the string. The only difference we will find is the logical, not operator (!) used before the contains() method. It will negate the result and will pass the assertion only when the expected string is not present in the response.
How to validate the value of a node in the SOAP response?
As we know, the XPath Match assertion allows you to use an XPath expression to select the content from the target response node and compare it with the value you expect. To explain the usage of how to implement this assertion with the help of script assertions, we will use the BookStore SOAP Service.
Suppose you want to validate that the title of the second book in the response of BookStore API is not null. We can validate the same using the script assertions. Let's see how to achieve the same.
Before writing the assertion, let's have a quick look into the XML response of the BookStore SOAP Service to figure out the XPath of the book title.
We'll need to write following script assertion for validation of the title in the XML response:
import com.eviware.soapui.support.XmlHolder
def holder = new XmlHolder( messageExchange.responseContentAsXml )
def responseXml = holder.getPrettyXml()
def title = holder.getNodeValue('//ns1:BooksResult/ns1:Books/ns1:CustomBookModel[2]/ns1:Title')
assert title.equals('Learning JavaScript Design Patterns')
Let's understand the above code snippet in more detail:
Apart from using the other methods of the Xmlholder class, we have used the "getNodeValue" method to get the value of the specific node, as shown below:
def title = holder.getNodeValue('//ns1:BooksResult/ns1:Books/ns1:CustomBookModel[2]/ns1:Title')
Here, we have mentioned the XPATH of the title of the second book. The 'ns1' refers to the namespace in the XML response. The first namespace in the XML is referred to as 'ns1' by default. If there would have been a second namespace, then it would have been 'ns2' and so on.
We have used 'ns1: CustomBookModel[2]' to get the title of the second book. Pay attention that the first element of the list starts at index 1 and not index 0.
And then we have used the equals() to validate the value of the title as shown below:
title.equals()
So, this way, we can validate the value of any node in the XML response of the SOAP service.
How to validate the headers of the SOAP response?
Like other assertions, the script assertions can assert response headers in SoapUI. Let's add another script to verify the response status from headers of the BookStore SOAP Service.
Before adding assertions for headers, let's have a look on bookstoreAPI response headers as shown below:
The below code snippet validates the header '#status#', which contains the response code of bookstore API.
def status = messageExchange.responseHeaders["#status#"]
assert status.toString().equals('[HTTP/1.1 200 OK]')
As we can see, the responseHeaders method of messageExchange interface fetches response headers. Once, we have all the headers; we can put any assertions for validating the values, as in the above case, we converted it to string and checked the value to be equal to "[HTTP/1.1 200 OK]"
How to validate the response time of the SOAP response?
We can also use the script assertions to validate the response time of the service as well. As we know, response time is the total time it takes to respond to the request of the service. Let's see how we can do this using scripts in SoapUI:
assert messageExchange.timeTaken < 900
The messageExchange.timeTaken is the property used to get the response time of the SOAP request.
How to validate the values for a range in the SOAP response?
While we can add assertions to validate the exact match of a value, it is also possible to apply fuzzy assertions. In other words, we can add an assertion to check for values that are in between certain limits, and we can achieve this with the help of script assertions in SoapUI.
Suppose we need to check whether the pages of our book are within range of 200-300 pages. We can use the following script assertion to validate the same:
import com.eviware.soapui.support.XmlHolder
def holder = new XmlHolder( messageExchange.responseContentAsXml )
def responseXml = holder.getPrettyXml()
def pages = holder.getNodeValue('//ns1:BooksResult/ns1:Books/ns1:CustomBookModel[2]/ns1:Pages')
log.info pages
assert pages.toInteger() > 200 && pages.toInteger() < 300
Let's understand what we trying to do here is:
- We have got all the pages in the XML response using the "getNodeValue" method for the "Pages" as shown below:
def pages = holder.getNodeValue('//ns1:BooksResult/ns1:Books/ns1:CustomBookModel[2]/ns1:Pages')
- Then we converted the value to "integer" using the "toInteger" method and compared with values 200 and 300 as shown below:
assert pages.toInteger() > 200 && pages.toInteger() < 300
So, we can retrieve any value from the XML response and validate it against any expected values using the script assertions in SoapUI.
Key Takeaways
- Firstly, Script assertions can be used to put validations programmatically on any values of the SOAP service response.
- Also, using the scripting language for assertions, give more control and freedom to test developers for all kinds of assertions.
- Additionally, the messageExchange object available in the script exposes a bunch of properties related to the last request/response messages, which can be used to get the response body and headers.
- Finally, the script assertions can be used to validate all the sections of the response, such as body, headers, etc.
Conclusively, we all should have a sound understanding of how to implement script assertions in SoapUI for the SOAP services. Let's now move to the next article where we will understand how we can apply script assertion in SoapUI for the REST services.
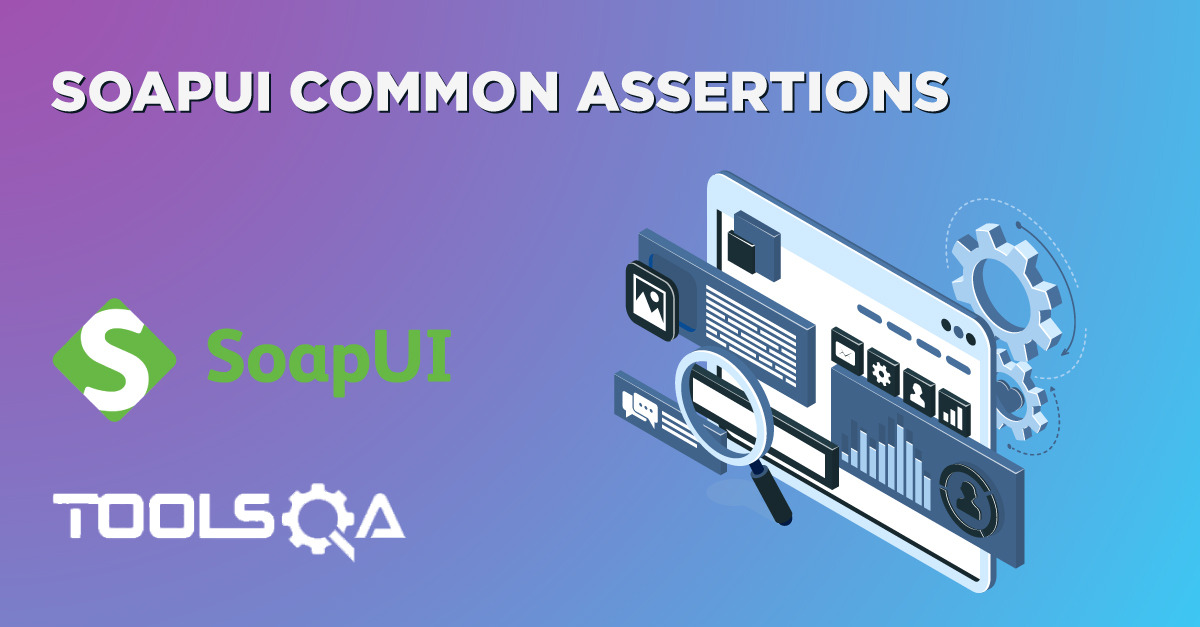
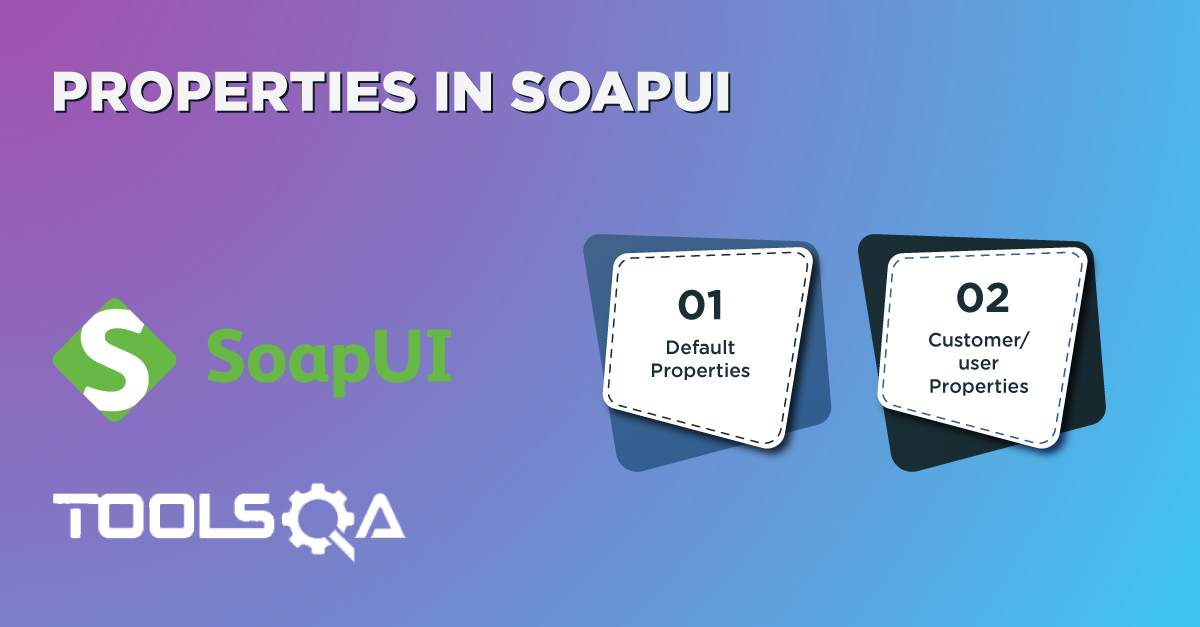