Organizing things in proper order is generally the nature of most of the human beings. While organizing things, people usually tend to group similar things and tagging each group with a specific heading. Similarly, applying the same theory to data, while organizing data in computing, it is always good to combine a similar set of data and provide them with a single name. So, how to do the same in programming languages*? Arrays are the answer to that. Let's discuss the concept of Array in JavaScript covering across the following topics:
- What is an Array in programming languages?
- What is an Array in JavaScript?
- How to declare and initialize an Array in JavaScript?
- How to access the elements of an Array in JavaScript?
- What are the standard operations on Arrays in JavaScript?
What is an Array in programming languages?
The array is one of the most used data structure. Additionally, it stores a collection of similar kinds of objects. Moreover, it helps to organize the data so that values can be easily maintained, searched, and sorted.
For instance, let's take a situation where we need to store five integer numbers. If we use simple variable and data type concepts of programming language, then we need five variables of int data type. Therefore, the program will be as follows:
var number1 = 10;
var number2 = 20;
var number3 = 30;
var number4 = 40;
var number5 = 50;
It was easy because we had to store just five integer numbers. Now let's assume we have to store 5000 integer numbers. Are we going to use 5000 variables?
The majority of the programming languages provide a concept called "Array" to handle such situations. Therefore, instead of declaring individual variables, such as number1, number2, ..., number99, you declare one array variable number of integer type and use number[0], number[1], and ..., number[99] to represent individual variables. Here, 0, 1, 2, .....99 are index associated with array variable. Moreover, they are being used to describe individual elements available in the array.
All arrays consist of contiguous memory locations. Additionally, the lowest address corresponds to the first element and the highest address to the last element. It looks like below in the form of a diagram:
Now, let's move to the next section to understand the details of arrays from a JavaScript perspective.
What is an Array in JavaScript?
In most of the programming languages, the array is a reference to the multiple variables. However, in JavaScript array is a single variable that stores various elements. That is to say, neither the length of the array nor the types of its elements it stores, are fixed. In other words, an array's length can change at any time. Moreover, the data can store at non-contiguous locations in the array. Therefore, unlike other programming languages, arrays in JavaScripts are not guaranteed to be dense. Let's understand further how arrays can be declared and initialized in JavaScript:
Procedure to declare and initialize an Array in JavaScript?
As discussed above, JavaScript array is an object that represents a collection of similar type of elements. Now to initialize that array object, JavaScript provides three ways:
- First, Array declaration and initialization using JavaScript Array Literals.
- Second, it's declaration and initialization using JavaScript Array directly (new keyword).
- Third, it's declaration and initialization using JavaScript Array constructor (new keyword).
Let's discuss all these methods of array initialization in detail:
Array declaration and initialization using JavaScript Array Literals:
One of the easiest ways to initialize arrays in JavaScript is using the literals such as String, Number, etc. In other words, in this method, the array declaration, and initialization happens in the same step. Its syntax looks like below:
Syntax:
var variableName = [literalValue1,literalValue2,literalValue3 ….. literalValueN]
The variableName is the name of the array. Moreover, all the variables in square bracket([]) are the elements of the array.
The below code snippet shows a working example for the initialization of arrays using the "String" literals.
<html>
<body>
Demonstrating Array initialization using Literals.
</br>
<script type="text/javascript">
var arrayValue = ["Tools", "QA", "is", "best", "tutorial", "site"]; // Initialize the array.
document.write(arrayValue); // Prints complete array.
document.write("</br>");
</script>
</body>
</html>
Save the file with name arrayLiteralInitialization.html. In addition to this, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we can see in the above example, arrayValue is the array declared and initialized using String literals. In this way, the comma-separated values of the array are provided directly in the square brackets ([ ]). Moreover, they are initialized to the array variable using the equal(=) operator. So, in this case, the declaration and initialization of array has been done in the same step.
Array declaration and initialization using JavaScript Array directly (new keyword)
Arrays in JavaScript can also be declared using the new keyword. After that, the initial values can be initialized by assigning the values to the individual index of the array. Its syntax looks like below:
Syntax:
var variableName = new Array();
variableName[0] = "value"; // The first element of array has been assigned value in this step
The below code snippet shows a working example for the declaration of arrays using the "new" keyword.
<html>
<body>
Demonstrating Array initialization using new Keyword:
</br>
<script type="text/javascript">
var arrayValue = new Array(); // Declare array
arrayValue[0] = "Tools"; // Initialize values to initidividual elements.
arrayValue[1] = "QA";
arrayValue[2] = "Tutorial";
document.write(arrayValue);
document.write("</br>");
</script>
</body>
</html>
Save the file with name arrayDemoUsingNewKeyword.html. Thereafter, open it in a browser. It should show the output as:
As we can see in the above example, arrayValue is the array declared using the new keyword. Moreover, the individual values initialize using String literals.
Array declaration and initialization using JavaScript Array constructor (new keyword):
JavaScript also provides a way in which arrays can be declared and initialized in the same step using the "new" keyword. In other words, the data of the array elements can be initialized by passing values to the constructor itself. Its syntax looks like below:
Syntax:
var variableName = new Array(value1,value2, …., valueN);
The below code snippet shows a working example for declaration and initialization of arrays using the "new" keyword
<html>
<body>
Demonstrating Array initialization using new Keyword and passing values with constructor:
</br>
<script type = "text/javascript">
var arrayValue = new Array("Tools", "QA","Tutorials");
document.write(arrayValue);
document.write("</br>");
</script>
</body>
</html>
Save the file with name arrayInitializationUsingConstructor.html and open it in some browser. It should show the output as:
As we can see in the above example, arrayValue is the array declared and initialized using the new keyword in the same step.
How to access elements of an Array in JavaScript?
As we know that JavaScript arrays are a particular type of object, and like all the programming languages, the [] operator can access an array item. Moreover, JavaScript arrays are zero-index arrays. In other words, the first element of an array is at index 0. Similarly, the last element is at the index equal to the value of the array's length minus 1. Using an invalid index number returns "undefined". The [] operator first converts the expression inside the square brackets to a string then uses that string to access the array elements.
The below code snippet shows an example of how to access the elements of the array using indexes:
<html>
<body> Demonstrating Accessing Array Elements using index </br>
<script type="text/javascript">
var firstArray = ["Tools", "QA", "is", "best", "tutorial", "site"];
document.write("Elements of First Array are : ");
document.write(firstArray[0] + ', ');
document.write(firstArray[1] + ', ');
document.write(firstArray[2] + ', ');
document.write(firstArray[3] + ', ');
document.write(firstArray[4] + ', ');
document.write(firstArray[5]);
document.write("</br>");
var secondArray = new Array();
secondArray[0] = "Tools";
secondArray[1] = "QA";
secondArray[2] = "Tutorial";
document.write("Elements of Second Array are : ");
document.write(secondArray[0] + ', ');
document.write(secondArray[1] + ', ');
document.write(secondArray[2]);
document.write("</br>");
var thirdArray = new Array("Tools", "QA","Tutorials");
document.write("Elements of Third Array are : ");
document.write(thirdArray[0] + ', ');
document.write(thirdArray[1] + ', ');
document.write(thirdArray[2]);
document.write("</br>");
</script>
</body>
</html>
Save the file with name accessingArrayElements.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, no matter what way creates an array, the index of individual elements can access its elements.
What are the standard operations on Arrays in JavaScript?
Apart from the creation and accessing elements of the arrays, JavaScript provides specific methods that perform some everyday operations on the array and its elements. Few of them are:
- Iterate all the elements of an array.
- Add a new element to the end of the array.
- Add a new element to the front of the array.
- Remove an element from the end of the array.
- Remove and element from the front of the array.
- Find the index of an element in the array.
- Remove an element from the specified index of the array.
Let's discuss all these array operations in detail and understand how to accomplish the these in JavaScript:
How to Iterate all elements of an array in JavaScript?
JavaScript arrays provide a "forEach" method, using which a user can iterate and access all the elements of the array.
The below code snippet shows an example of how to achieve the same:
<html>
<body> Demonstrating Iterating over Array Elements:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA", "is", "best", "tutorial", "site"];
document.write('Elements and indexes of array elements are: </br>')
firstArray.forEach(function(item, index, array) { // Iterate over each array element
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with name iteratingOverArrayElements.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, using the "forEach" method, a user can quickly iterate over all the elements of the array.
How to add a new element to the end of the array in JavaScript?
Arrays in JavaScript provides an inbuilt method "push", using which helps the user to directly add an element to the end of the given array.
The below code snippet shows an example of how to push an element to the end of the array using JavaScript:
<html>
<body> Demonstrating pushing an element to the end of the array:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA"];
document.write('Elements and indexes of array elements before append at end: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
firstArray.push("Website");
document.write('Elements and indexes of array elements after append at end: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with the name pushElementToArray.html. Thereafter, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As seen above, the "push" method inserts a new element at the end of the array.
How to add a new element to the front of the array in JavaScript?
Arrays in JavaScript provides an inbuilt method "unshift", using which a user can directly add an element to the front of the given array.
The below code snippet shows an example of how to add a new element to the start of the array:
<html>
<body> Demonstrating Appending an element to the start of the Array:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA"];
document.write('Elements and indexes of array elements before append at start: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
firstArray.unshift("Website"); // Add new element to start of the array
document.write('Elements and indexes of array elements after append at start: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with the name unshiftElementToArray.html. Thereafter, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the "unshift" method has inserted a new element (Website) at the front of the array.
How to remove an element from the end of the array in JavaScript?
Arrays in JavaScript provides an inbuilt method "pop", using which a user can directly remove an element to the end of the given array.
The below code snippet shows an example of how to remove an element from the end of the array:
<html>
<body> Demonstrating Removing element from end of the Array:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA", "Website"];
document.write('Elements and indexes of array elements are before pop: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
firstArray.pop();
document.write('Elements and indexes of array elements are after pop: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with the name popElementFromArray.html. Thereafter, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the "pop" method has removed the last element ("Website") from the end of the array.
How to remove an element from the front of the array in JavaScript?
Arrays in JavaScript provides an inbuilt method "shift", using which a user can directly remove an element to the front of the given array.
The below code snippet shows an example of how to remove an element from the front of the array:
<html>
<body> Demonstrating Removing element from start of the Array:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA", "Website"];
document.write('Elements and indexes of array elements are before shift: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
firstArray.shift(); // Removes element from the start of the array
document.write('Elements and indexes of array elements are after shift: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with name shiftElementFromArray.html. Thereafter, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the "shift" method has removed the first element ("Tools") from the start of the array.
How to find the index of an array element in JavaScript?
Arrays in JavaScript provides an inbuilt method "indexOf", using which a user can get the index of any element in the array.
The below code snippet shows an example of how to find the index of the given element in the array:
<html>
<body> Demonstrating how to get index of an Array element:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA"];
document.write("Index of element -- Tools --", firstArray.indexOf('Tools'), '</br>');
document.write("Index of element -- QA --", firstArray.indexOf('QA'), '</br>');
</script>
</body>
</html>
Save the file with name indexofElement.html. Thereafter, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the "indexOf" method returns the numerical indexes of the elements of the Array.
How to remove an item from the index position of an Array?
Arrays in JavaScript provides an inbuilt method "splice", using which a user can directly remove an element from a given index array.
The below code snippet shows an example of how to remove an element from a specified index of the array:
<html>
<body> Demonstrating Removing element from specified index of the Array:</br>
<script type="text/javascript">
var firstArray = ["Tools", "QA", "Website"];
document.write('Elements and indexes of array elements before splice: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
firstArray.splice(firstArray.indexOf("QA"),1); // Remove the QA element from array.
// First parameter specified the index of element
// and 2nd parameter specifies the number of elements to be removed
document.write('Elements and indexes of array elements after splice: </br>')
firstArray.forEach(function(item, index, array) {
document.write(item, '---', index, '</br>');
})
</script>
</body>
</html>
Save the file with name spliceElementFrom Array.html. After that, open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the "splice" method takes two parameters. The first parameter is the index of the array. Which, in turn, tells from where to start removing the elements. And, the second parameter is the number of elements that need to remove from the array.
Key Takeaways
- Arrays in JavaScript are a single variable that internally stores multiple elements.
- Moreover, they can declare and initialize either using direct string literals within square brackets ([ ]) or by using the "new" keyword.
- In addition to this, Arrays in JavaScript provide various array manipulation functions such as "push, pop, shift and unshift".
To conclude, let's now move to the next article to understand various other advanced in-built methods provided by Arrays. Which, in turn, will simplify the array operation to a great extent.
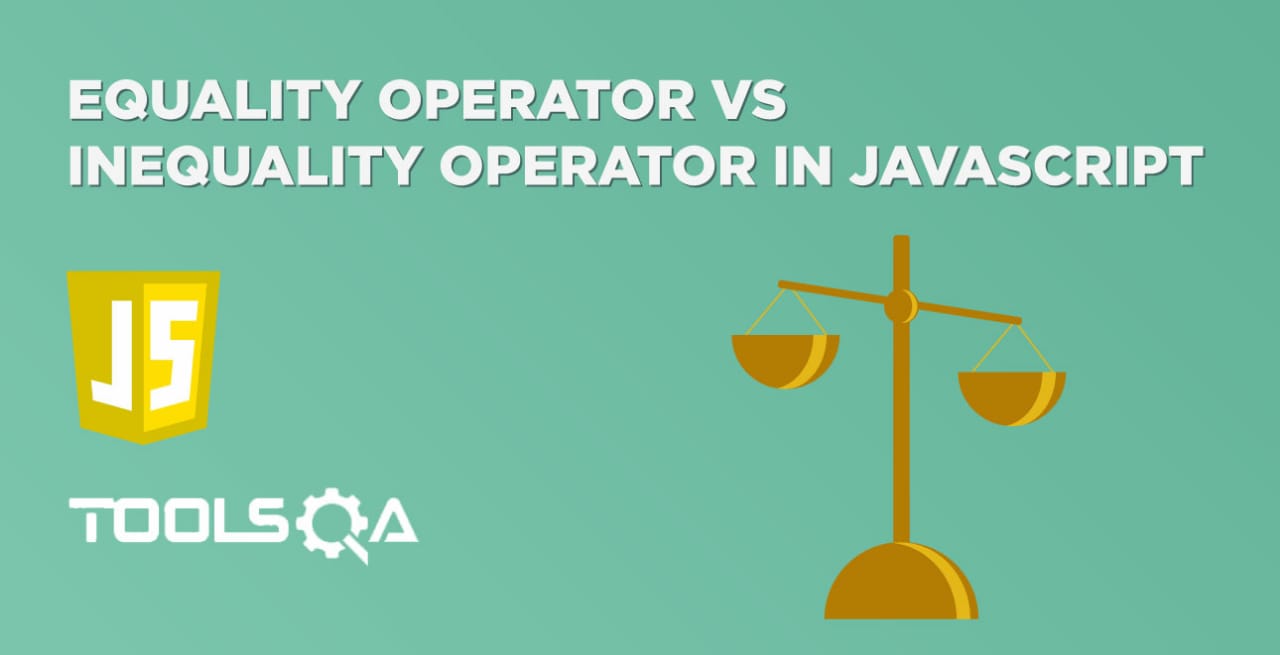
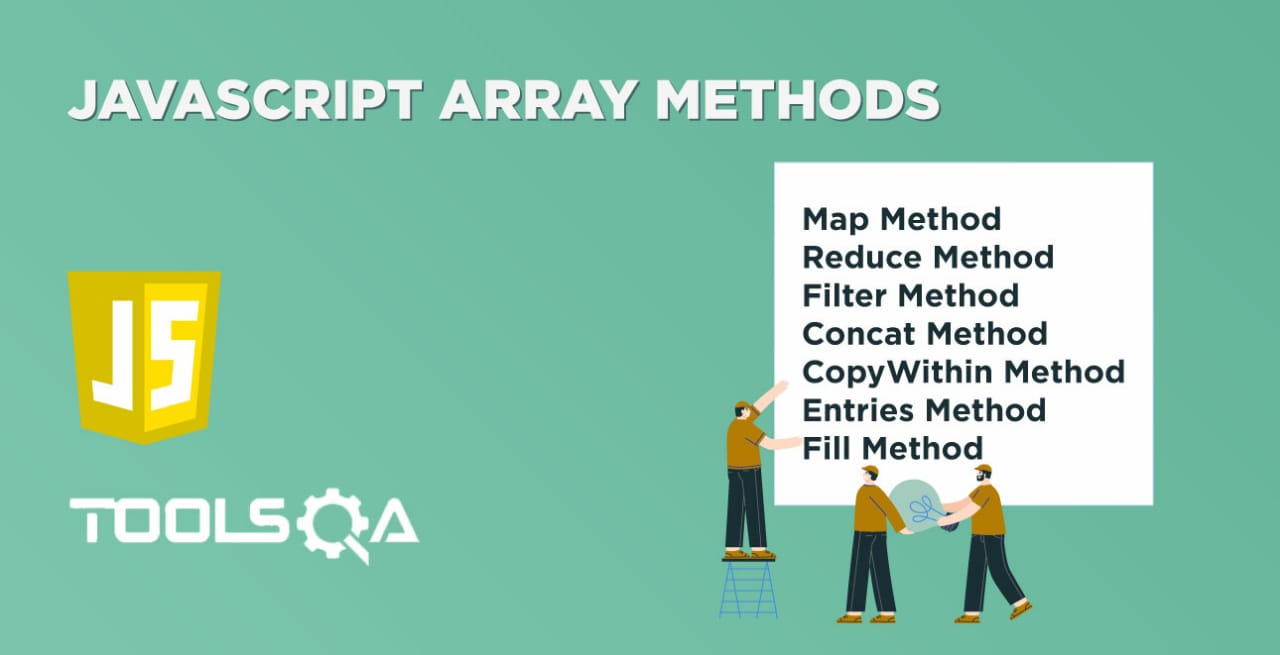