During programming, we all should have faced the scenarios when multiple instances of one type need to create. The object-oriented programming came into existence to support this functionality, which used the concept of classes to represent living types and allowed to create multiple objects of the same type. Similar to other OOPS based programming languages, JavaScript also supports the concept of classes. Classes got introduced in JavaScript from ES6 onwards. In this article, we will understand the concept of JavaScript Classes by covering the following topics:
- What is a Class in JavaScript?
- How to create objects of classes in JavaScript?
- What is a constructor in JavaScript Classes?
- How to define and invoke Class Constructors with no parameters?
- How to define and invoke Class Constructors with parameters?
- What are the static properties and static methods of a class?
- How to implement Class Inheritance in JavaScript?
What is a Class in JavaScript?
Classes in JavaScript are a type of function only, but instead of using the keyword "function", the keyword "class" is used to declare a class. Its syntax looks like below:
class classname {
//variables and methods which need to as part of an object
}
where "classname" represents the name of the class, and the curly brackets specify the start and end of the class definition.
Let's understand the details of the class with the help of a real-world example. Suppose we want to create a data structure that represents a "Student" and can we used to create different instances of the Student which resonates with various students. Now, each student can have different names, date of birth, father name, and mother name. So, we can represent such data structure with the help of a class, and we can create different objects of that class which represents various students. So, considering the syntax as mentioned above, we can describe the "Student" class as follows:
class Student{
//Properties/attributes of the Student
name, dob, fatherName, motherName;
// Methods for manipulating/exposing Student's properties
getStudentName()
{
console.log(this.name);
}
}
As is evident from the above example, the class Student has various properties like name, dob, fatherName, and motherName and has the method getStudentName(), which prints the name of the student.
How to create objects of classes in JavaScript?
To access the properties and methods of the class, we need to create an object of the class and then access the properties and methods using the dot(.) operator. Similar to functions, JavaScript provides the "new" operator to create the object of a class. Its syntax looks like below:
Syntax to object creation:
const object1 = new classname();
const object2 = new classname();
var object3 = new classname();
var object4 = new classname();
.
.
.
let objectN = new classname();
Syntax to accessing properties and methods using object of the class:
// Setting value of a property
object1.property1 = value1;
//Invoking method of a class
objeect1.method1();
where,
"property1" is one the property of the class which has been assigned value as "value1".
"method1" is the name of the class's method.
Let’s understand these details using the following example, where we are creating an object with the help of the class:
<html>
<meta charset="utf-8"/>
<body> Demonstrating creation of objects with the help of class</br>
<script type="text/javascript">
class Student{
name;
getStudentName(){
console.log(this.name);
}
}
const student1 = new Student();
student1.name="Arun";
const student2 = new Student();
student2.name="Ravinder";
const student3 = new Student();
student3.name="Lakshay";
student1.getStudentName();
student2.getStudentName();
student3.getStudentName();
</script>
</body>
</html>
Save the file with name classDemo.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we see in the above example, we have created the class of Student and created three objects (student1,student2, and student3) with the help of a new keyword. In the Student class, we have a variable name and method getStudentName.
What is a Constructor in JavaScript Classes?
If we want to perform some initialization or functionality while creating the object itself, you can achieve it with the help of defining those details inside a particular function called "constructor", which invokes automatically when the creation of the object of the class happens. Now depending on the implementation, the "constructor" function may or may not accept parameters. Let's understand these various implementations of the "constructor" function in the below sections:
How to define and invoke Class Constructors with no parameters?
If you don't define any constructors in the program, as we did in the above example of Student, JavaScript will create its own "default constructor" with an empty body. But, if you need to perform some operation while creating the object of the class, you can also define a constructor explicitly. Its syntax looks like below:
Syntax:
class classname{
constructor(){
//code need to executed
}
}
Let’s understand these details using the following example, where we are creating a constructor in the Student class:
<html>
<meta charset="utf-8"/>
<body> Demonstrating constructor inside the class</br>
<script type="text/javascript">
class Student{
constructor(){
console.log("I am in constructor")
}
name;
getStudentName(){
console.log(this.name);
}
}
const student1 = new Student();
student1.name="Arun";
student1.getStudentName();
const student2 = new Student();
student2.name="Ravinder";
student2.getStudentName();
const student3 = new Student();
student3.name="Lakshay";
student3.getStudentName();
</script>
</body>
</html>
Save the file with name constructorWithNoParameters.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we see in the above example, we have created the constructor, which is printing, "I am in the constructor". By creating this JavaScript is an overriding default constructor and executing the user-defined constructor whenever a new object is created.
How to define and invoke Class Constructor with Parameters?
If you want to assign some value to the properties of the class while creating the object itself, you can achieve this with the help of a parameterized constructor. Its syntax looks like below:
Syntax:
class classname{
constructor(parameter1,parameter2.....,parameterN){
//Code need to be executed
}
}
Let’s understand these details using the following example, where we are creating a constructor with the parameters in the class:
<html>
<meta charset="utf-8"/>
<body> Demonstrating constructor inside the class</br>
<script type="text/javascript">
class Student{
name;
constructor(name){
this.name = name;
console.log("I am in constructor")
}
getStudentName(){
console.log(this.name);
}
}
var student1 = new Student("Arun");
student1.getStudentName();
var student2 = new Student("Ravinder");
student2.getStudentName();
var student3 = new Student("Lakshay");
student3.getStudentName();
</script>
</body>
</html>
Save the file with name constructorWithParameters.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As we see in the above example, we can create the constructor with parameters and pass the data to the constructor while creating the object.
Note: Unlike other object-oriented languages, JavaScript allows only one constructor in a class.
What are the Static Properties and Static Methods of a class?
If you want certain properties and methods of a class to be associated with the class itself, instead of the objects of the class, they can declare as static. So, to invoke these methods and access these properties, the instance of the class is not needed, but they can invoke using the class name itself. Its syntax looks like below:
class classname {
static property1 = value1;
static method1(){
// Perform needeed action
}
}
These properties and methods can invoke directly using the class name, as shown below:
classname.property1;
classname.method1
Let's understand the details and usage of the static properties and methods by modifying the above example as below:
<html>
<meta charset="utf-8"/>
<body> Demonstrating static propeerties and methods of class in JavaScript</br>
<script type="text/javascript">
class Student{
static name = "Ravi";
static getStudentName(){
console.log(this.name);
}
}
Student.getStudentName();
</script>
</body>
</html>
Save the file with name staticMethodsInClass.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, we invoked the static method "getStudentName()" directly using the class name, and it was able to access the static property name.
How to Implement Class Inheritance in JavaScript?
In JavaScript, one class inherits other classes using the "extends" keyword. The child class will have access to all the methods of the parent class, and you can invoke all the methods of the parent class using the child class object.
JavaScript provides one method, named "super()", which can invoke inside the child class constructor and which in turn will automatically call the parent class constructor. Its syntax looks like below:
class classname1 extends classname2 {
constructor() {
super();
// Other statements for child class initialization
}
// methods of the child class
}
Let's understand the concept of inheritance deeply with the help of the following example:
<html>
<meta charset="utf-8"/>
<body> Demonstrating inheritance in JavaScript</br>
<script type="text/javascript">
class Student{
constructor(className){
console.log("I am in Student's constructor");
this.className = className;
}
getClassName(){
console.log(this.className);
}
}
class collegeStudent extends Student {
constructor(className) {
super(className);
console.log("I am in collegeStudent's constructor");
}
}
const object1 = new collegeStudent("Masters");
object1.getClassName(); // Invoke parent class method
</script>
</body>
</html>
Save the file with name inheritance.html and open it in any browser(Chrome, Firefox, or IE) . It should show the output as:
As we can see from the above code snippet and screenshot, that we inherited the class collegeStudent from the class Student using the "extends" keyword. It also used the super() method in the child class's constructor to invoke and pass parameters to the parent's class constructor. We can see from the output that the parent class constructor invoked before the child class constructor. Also, we invoked the parent class method getClassName using the child class object.
Key Takeaways
- A class is a user-defined blueprint or prototype from which we create objects. Moreover, it represents the set of properties or methods that are common to all objects of one type.
- Additionally, a constructor is a block of code that initializes the newly created object.
- A class in JavaScript can contain static properties and static methods, but they can bee invoked only using the class name and doesn't need any object for their invocation.
- In addition to the above, inheritance can implement in JavaScript by using the "extends" keyword.
To conclude, let’s now move to the next article to understand the concept of "Error handling in JavaScript".
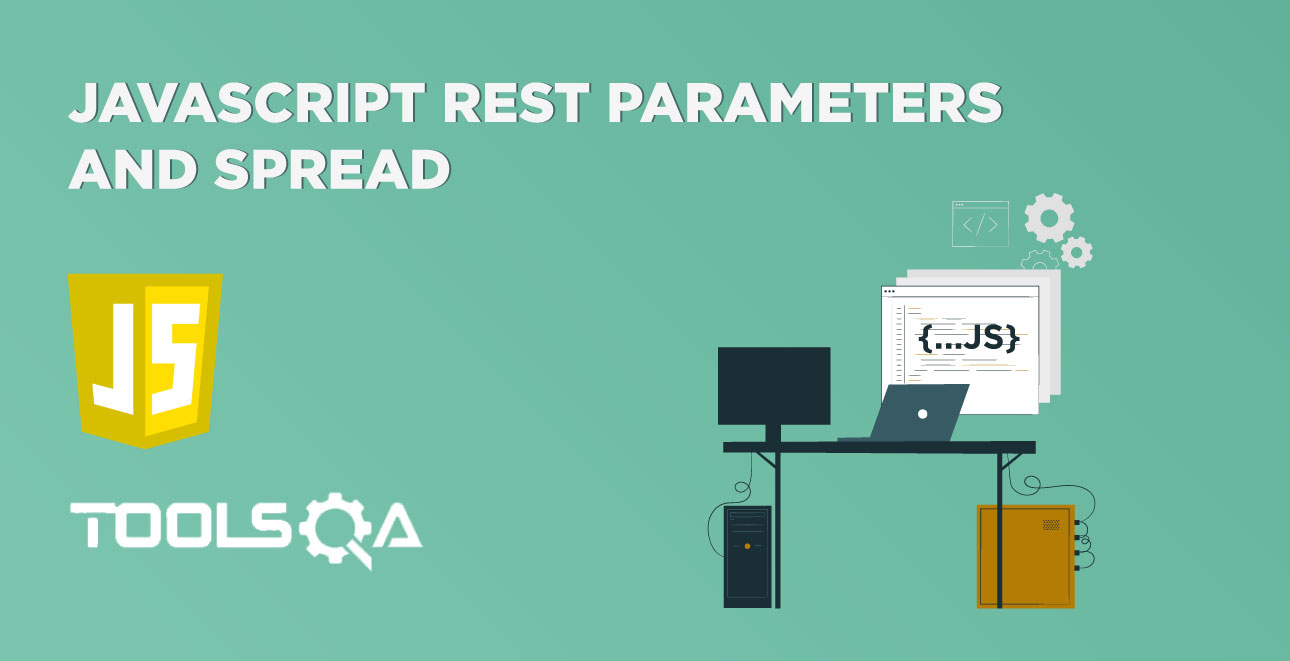
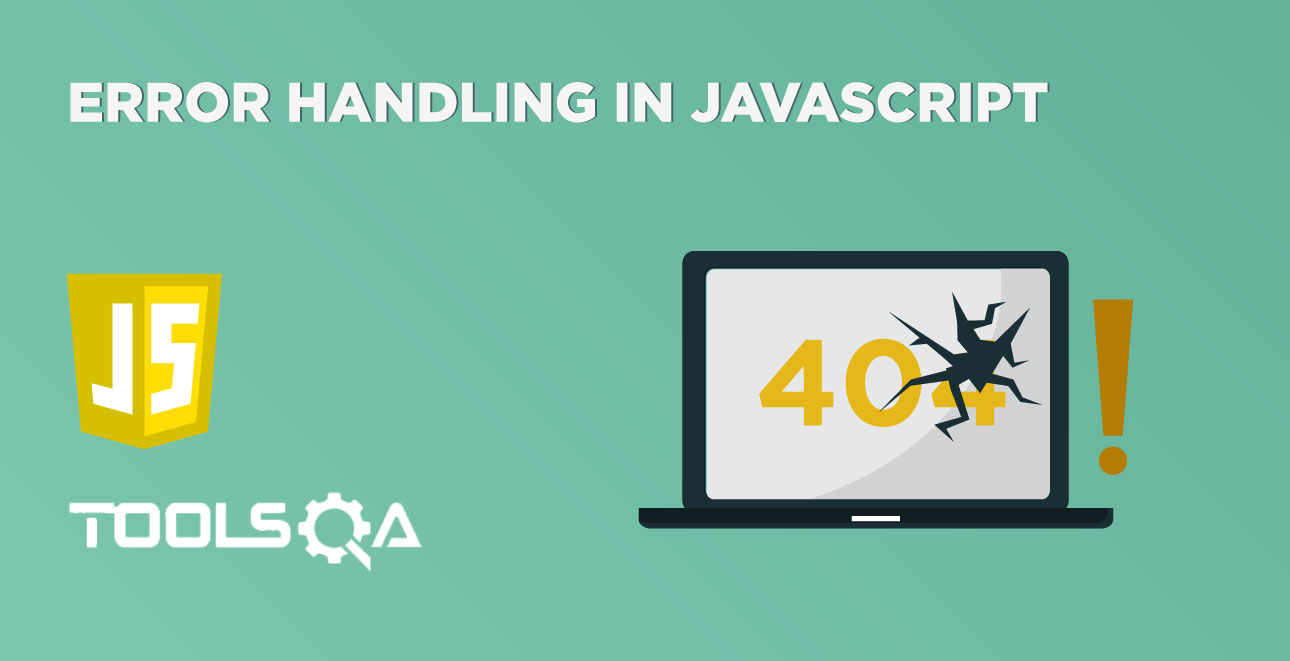