As a programmer, we would have certainly faced a scenario that whenever we try to read/edit a code (either written by yourself or a co-worker), which one wrote a couple of years back, we find it difficult to understand the code. The reason being, we generally need to go through each line of code to understand the logic and purpose of code. So, what was missing in this case? -- comments!. Yes, if the code had explicit comments, it would have made the life of a programmer effortless to understand the same. Let's deep dive further to understand the usage of comments in JavaScript through the following topics:
- What are Comments in Programming languages?
- How to write Comments in JavaScript?
- Procedure to write Single-Line Comment?
- How to write Multi-Line Comment?
What are Comments in Programming Languages?
Comments are one of the basic functionality provided by all the programming languages. Good comments convey essential information to the human reader of the code that the code cannot convey by itself. They make the source code more natural for humans to understand, and compilers and interpreters generally ignore them. Comments signify the intent of your code so that the other person who opens the code can understand it easily.
Another primary purpose of comments can be to skip some of the code from execution when you are debugging your code. As a programmer, you can comment on the code which you don't want to execute.
Generally, comments help in the following areas:
- Describing the code: Programmers can use comments to describe the intent of the piece of the code so that his/her intention is clear to all the other co-workers. E.g., If we consider the below section of code:
// This is a global variable which will be used across the whole scope
var globalVariable = 10;
The variable declaration in the comment, as mentioned above, makes it clear that the declaration of the variable in the global scope has happened.
- Debugging: One of the common ways of debugging code is by putting the "print" statement in the code. Which, in turn, easily depicts the needed information and becomes very handy for debugging any code. Generally, programmers can comment on those lines after debugging is complete. As a result, the same can be utilized in the future if the same code needs debugging again.
- Resource inclusion: Extra information like "Copyright notices, logos, developer information" can be embedded in the code itself and can be wrapped in comments. This is so the compilers or interpreters can ignore that same. A few of the IDE (Integrated development environment) auto-generates comments whenever a new file adds to the code-base.
- Automatic documentation generation: Multiple tools scan the code and gather all the comments from the specified location of the code. So, if the programmers have provided the comments in a pre-defined format and at a pre-defined site in the code, these tools can generate quick and easy documentation.
- Pseudocode specifications: Multiple times programmers will be aware of the logic of the code but need more exploration before implementing the same in the chosen programming language. In that scenario, they can put the pseudocode in the same code file where they are planning to write the actual code. Therefore, it will always be the right reference for them whenever they go for practical implementation.
So, be it providing additional information about the piece of code or be it excluding a piece of code from execution; comments are always a good friend for any programmer.
How to write JavaScripts Comments?
As we explained above that the comments are the best friend for any programmer. There can be a situation where you like to comment on just a single line and a situation where a whole block needs comment. For both situations, JavaScript provides a particular way to comment:
- Single-line comments: Comment out just a single line
- Multi-line comments: Comment out a whole block or multiple lines together
How to use Single Line comments in JavaScript?
Single line comments in JavaScript is created by placing two slashes "//" in front of the code or text you want the JavaScript interpreter to ignore. One uses these types of comments for commenting out single lines of code. Or for writing small notes for briefing the code. When the programmer places a "// " in front of the line, the interpreter will ignore the whole line. If a programmer inserts a "//" in between a line, then statement/code after "//" till the end of the line will be ignored by the interpreter. But the code from the start of the line till it "//" is part of the code. The below code snippet briefs a few usages of single-line comments:
Example:
<html>
<body>
Demonstrating Single line comments in javascript
</br>
<script type = "text/javascript">
//This the comment used to explain this code
//This entire section will not be complied by complier
document.write("After comments is executed");
//document.write("Commenting this code for temp purpose");
</script>
</body>
</html>
Save the file with name singleLineComment.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above code snippet and screenshot, we used single lines comments both to "give some additional information". And also "to comment-out the code which we don't want to be part of the execution".
How to use Multi-Line Comments in Javascript?
Consider a scenario where the programmer needs to comment on multiple lines of code. Or to provide information that spans across multiple lines. For such situations, you can use JavaScript's multi-line comment, which begins with /* and ends with */. The interpreter will ignore everything between the opening /* and */ in the code block above. These comments are "Block comments". The below code snippet briefs a usage of multi-line comments in JavaScript:
Example:
<html>
<body>
Demonstrating Multi line comments in javascript
</br>
<script type="text/javascript">
/*This the multi line comment used to explain this code
This entire section will not be complied by complier*/
document.write("After comments is executed");
/*document.write("Commenting this code for temp purpose");
document.write("Commenting this code for temp purpose");*/
</script>
</body>
</html>
Save the file with name multiLineComments.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above code snippet and screenshot, we use multi-lines comments both to "give some additional information". In addition to this, we also use it "to comment-out the code which we don't want to be part of the execution".
Key Takeaways
- We can use single-line comments (//) whenever we need to comment on only a single line of code. Or if we have to provide information that spans only in one line.
- Multi-line comments(/* */) are useful for scenarios where a block of code needs comment. Or the additional information that needs to be provided spans across multiple lines.
Let's move to the next article, where we will discuss the difference between Equality Operator (==) and Inequality Operator (===).
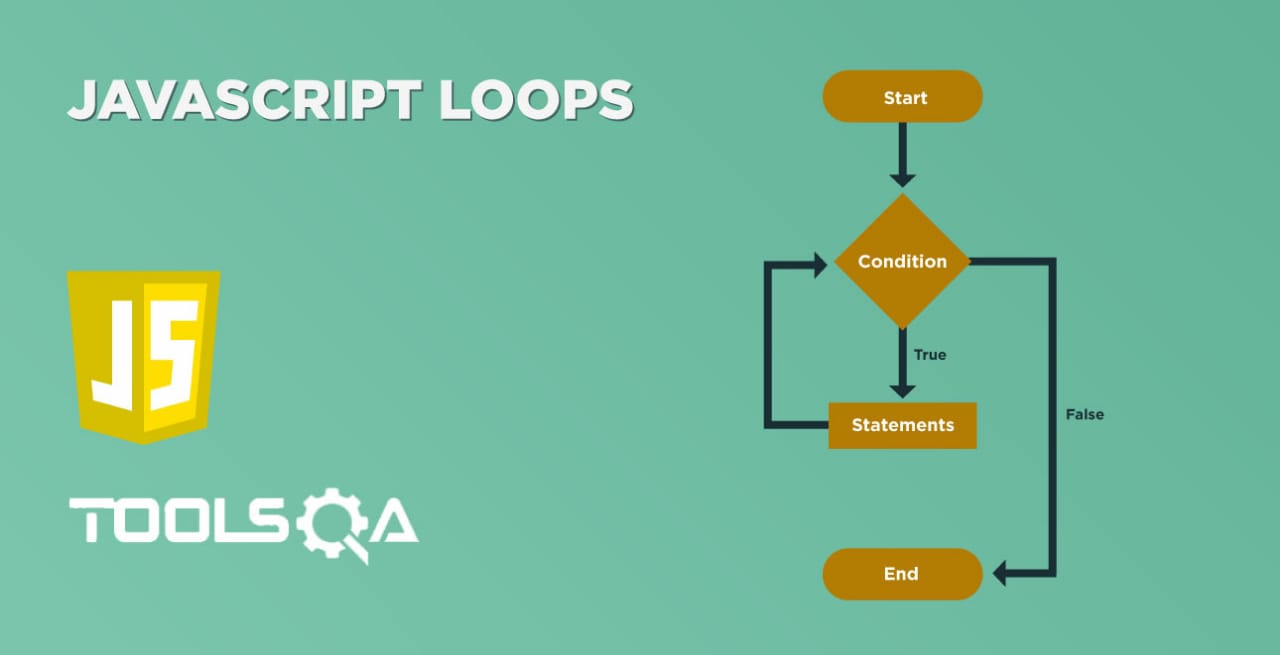
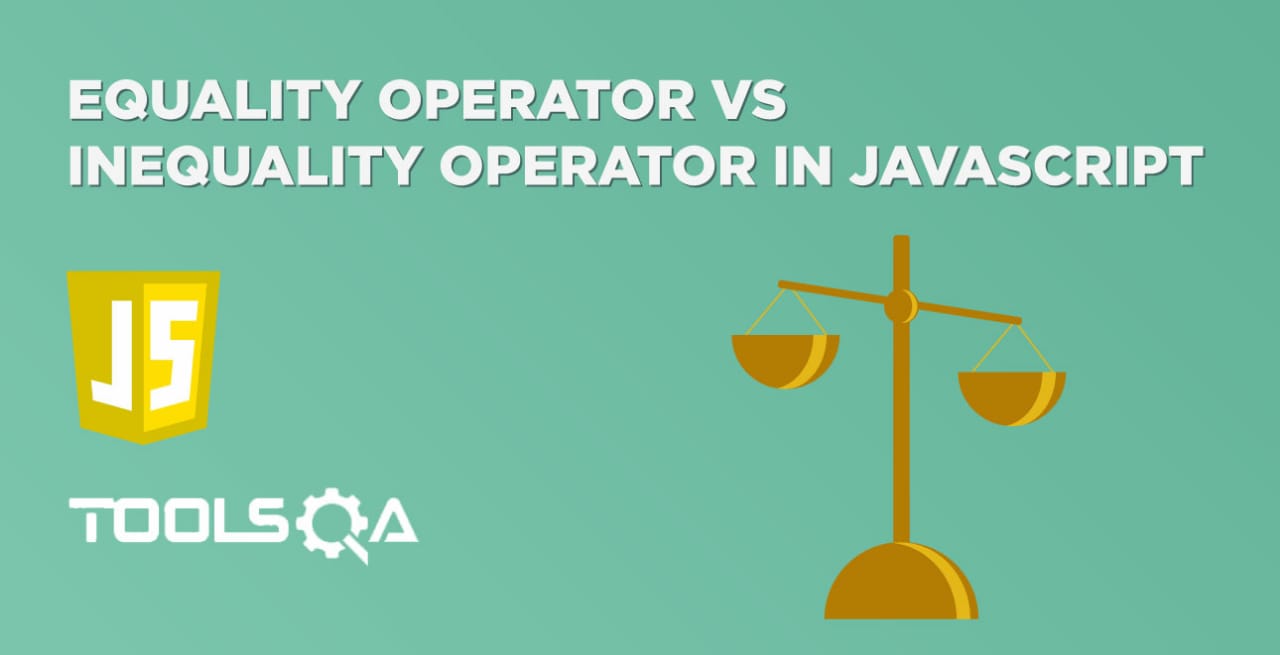