Let, Var, and Const are the various ways that JavaScript provides for declaration of JavaScript Variables. Var is an old way of declaring variables. Whereas, Let & Const came into the picture from the ES6 version. Before starting the discussion about JavaScript let Vs var Vs const, let's understand what ES is?
ES stands for Ecma Script, which is a scripting language specification specified by ECMA international. It standardized various implementations of JavaScript.
In this article, we will discuss differences of following ways of declaring a variable concerning their scope, use, and hoisting:
- Var keyword: What, How, and Where?
- Let keyword: What, How, and Where?
- Const keyword: What, How, and Where?
What is a "var" keyword
The "var" keyword is one of the ways using which we can declare a variable in JavaScript. Before the advent of ES6, var was the only way to declare variables. In other words, out of JavaScript let Vs var Vs const, var was the sole way to declare variables. Its syntax looks like below:
Syntax:
var variable = value;
Scope of var:
The scope specifies where we can access or use the variables. When we declare a variable outside a function, its scope is global. In other words, it means that the variables whose declaration happens with "var" outside a function (even within a block) are accessible in the whole window. Whereas, when the declaration of a variable occurs inside a function, it is available and accessible only within that function.
It is illustrated with the help of following code snippet:
<html>
<body> Demonstrating var scopes in javascript:</br>
<script type="text/javascript">
var globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
globalVariable = 10;
var localBlockVariable = 15;
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
}
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
document.write("The value of block local variable outside block is: ", localBlockVariable, "</br>");
function updateVariables() {
globalVariable = 20;
localBlockVariable = 25;
var localFunctionVariable = 30;
document.write("The value of global variable inside function is: ", globalVariable, "</br>");
document.write("The value of block local variable inside function is: ", localBlockVariable, "</br>");
}
updateVariables();
document.write("The value of global variable outside function is: ", globalVariable, "</br>");
document.write("The value of block local variable outside function is: ", localBlockVariable, "</br>");
// This following statement will give error, as the local function variable can't be accessed outside
// document.write("The value of function local variable outside function is: ", localFunctionVariable, "</br>");
</script>
</body>
</html>
Save the file with name varScopes.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident in the above screenshot, the variables declared in global and block scope are accessible in the whole window. In contrast, variables declared inside in the function can just be accessed within that function only.
Re-declaration of "var" variables:
The variables declared using var can be re-declared within the same scope also, and it will not raise any error.
Let's understand it with the help of following code snippet:
<html>
<body> Demonstrating var scopes in javascript:</br>
<script type="text/javascript">
var globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
var globalVariable = 10; // Re-declare in block
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
}
var globalVariable = 15; // Re-declare in same scope
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
</script>
</body>
</html>
Save the file with name varRedeclare.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is clear from the above screenshot, the same variable "globalVariable" has been declared multiple times without any error.
Hoisting of var:
Hoisting is a JavaScript mechanism where variables and function declarations move to the top of their scope before code execution.
E.g., See the below code snippet:
document.write(variable1);
var variable1 = "ToolsQA"
JavaScript will interpret it as:
var variable1;
document.write(variable1); // variable1 will be undefined
variable1 = "ToolsQA"
So var variables hoist to the top of its scope and initialize with a value of undefined.
Consider the below code snippet to validate the same:
<html>
<body> Demonstrating var hoisting in javascript:</br>
<script type="text/javascript">
document.write("The value of var variable is: ", varVariable, "</br>");
var varVariable = 5; // var variable declared later on
</script>
</body>
</html>
Save the file with name varHoisting.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
As is evident from the above screenshot, the value of a variable declared using var is printed as "undefined", if we access it before its declaration.
What is the "let" keyword?
In ES 2015 release, ES released one more keyword for declaration of variables, which is known as the "let". Its syntax looks like below:
Syntax:
let variable = value;
Scope of let:
let is block scoped. A block is a chunk of code bounded by {}. Moreover, a variable that one declares in a block with the "let" is only available for use within that block only.
Let's try to understand the same with the help of following code snippet:
<html>
<body> Demonstrating let scopes in javascript:</br>
<script type="text/javascript">
let globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
globalVariable = 10;
let localBlockVariable = 15;
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
}
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
// This following statement will give error, as the local block variable can't be accessed outside
// document.write("The value of block local variable outside block is: ", localBlockVariable, "</br>");
function updateVariables() {
globalVariable = 20;
localBlockVariable = 25; // This will raise error
let localFunctionVariable = 30;
document.write("The value of global variable inside function is: ", globalVariable, "</br>");
// This following statement will give error, as the local block variable can't be accessed outside
// document.write("The value of block local variable inside function is: ", localBlockVariable, "</br>");
}
updateVariables();
document.write("The value of global variable outside function is: ", globalVariable, "</br>");
// This following statement will give error, as the local block variable can't be accessed outside
// document.write("The value of block local variable outside function is: ", localBlockVariable, "</br>");
// This following statement will give error, as the local function variable can't be accessed outside
// document.write("The value of function local variable outside function is: ", localFunctionVariable, "</br>");
</script>
</body>
</html>
Save the file with name letScope.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
The above screenshot clearly shows that the variables declared using "let" are block-scoped and can't access outside the block in which the declaration happens.
Note: The error scenarios can be validated by uncommenting the lines one by one, which are marked as errors and validating the same in any of the browser console windows.
Re-declaration of "let" variables:
The variable declared using let can't be re-declared.
It can be demonstrated easily with the help of following code snippet:
<html>
<body> Demonstrating let re-declare in javascript:</br>
<script type="text/javascript">
let globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
// The following statement will raise an error
// let globalVariable = 10;
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
}
// The following statement will raise an error
// let globalVariable = 15; // Re-declare in same scope
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
</script>
</body>
</html>
Save the file with name letRedeclare.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
The above screenshot clearly shows that the variables using let can't be re-declared.
Note: The error scenarios can be validated by uncommenting the lines one by one, which are marked as errors and validating the same in any of the browser console windows.
Hoisting of let:
Just like var, let declarations hoist to the top. But, unlike var, which initializes as undefined, the let keyword does not initialize. So if you try to use a let variable before the declaration, you'll get a "Reference Error".
Consider the below code snippet to validate the same:
<html>
<body> Demonstrating let hoisting in javascript:</br>
<script type="text/javascript">
document.write("The value of let variable is: ", letVariable, "</br>");
let letVariable = 5; // let variable declared later on
</script>
</body>
</html>
Save the file with name letHoisting.html and open it in the Chrome browser. Also, open the Chrome dev-tools console to visualize the error raised. It should show the output as:
As is evident from the above screenshot, JavaScript raises "Uncaught ReferenceError" if we access the variable as let before its declaration.
What is the "const" keyword?
Variables declared with the "const" keyword maintain constant values and can't change their values during the scope. Its syntax looks like below:
Syntax:
const variable = value1;
Scope of const:
Similar to let, the scope of the const variables is also blocked.
The following code snippet will help us understand it better:
<html>
<body> Demonstrating const scopes in javascript:</br>
<script type="text/javascript">
const globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
// This following statement will give error, as const can't be assigned a new value
// globalVariable = 10;
const localBlockVariable = 15;
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
document.write("The value of block local variable inside block is: ", localBlockVariable, "</br>");
}
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
// This following statement will give error, as the local block variable can't be accessed outside
// document.write("The value of block local variable outside block is: ", localBlockVariable, "</br>");
function updateVariables() {
// This following statement will give error, as const can't be assigned a new value
//globalVariable = 20;
const localBlockVariable = 25; // This will be considered a new variable
const localFunctionVariable = 30;
document.write("The value of global variable inside function is: ", globalVariable, "</br>");
document.write("The value of block local variable inside function is: ", localBlockVariable, "</br>");
}
updateVariables();
document.write("The value of global variable outside function is: ", globalVariable, "</br>");
// This following statement will give error, as the local block variable can't be accessed outside
// document.write("The value of block local variable outside function is: ", localBlockVariable, "</br>");
// This following statement will give error, as the local function variable can't be accessed outside
// document.write("The value of function local variable outside function is: ", localFunctionVariable, "</br>");
</script>
</body>
</html>
Save the file with name constScope.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
The above screenshot depicts that const variables are block-scoped and can't update with a new value.
Note: try uncommenting the lines one by one, which are marked to raise an error and check the error in Chrome dev-tools console.
Re-declaration of const variables:
Similar to let variables, the variable declared using const can't be re-declared.
We can easily demonstrate it with the help of following code snippet:
<html>
<body> Demonstrating const re-declare in javascript:</br>
<script type="text/javascript">
const globalVariable = 5;
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
if (globalVariable == 5)
{
// The following statement will raise an error
// const globalVariable = 10;
document.write("The value of global variable inside block is: ", globalVariable, "</br>");
}
// The following statement will raise an error
// const globalVariable = 15; // Re-declare in same scope
document.write("The value of global variable outside block is: ", globalVariable, "</br>");
</script>
</body>
</html>
Save the file with name constRedeclare.html and open it in any browser (Chrome, Firefox, or IE). It should show the output as:
The above screenshot makes it clear that the const variables can't re-declare.
Note: try uncommenting the lines which are marked to raise an error and check the error in Chrome dev-tools console.
Hoisting of const:
Just like "let", "const" declarations hoist to the top but don't initialize.
Consider the below code snippet to validate the same:
<html>
<body> Demonstrating const hoisting in javascript:</br>
<script type="text/javascript">
document.write("The value of const variable is: ", varVariable, "</br>");
const constVariable = 5; // const variable declared later on
</script>
</body>
</html>
Save the file with name constHoisting.html After that, open it in the Chrome browser. Also, open the Chrome dev-tools console to visualize the error raised. It should show the output as:
As is evident from the above screenshot, JavaScript raises "Uncaught ReferenceError" if we access a variable that we declare as const before its declaration.
Key Takeaways for JavaScript Let vs Var vs Const
Below are the main learnings of our article on JavaScript let Vs var Vs const :
- If you declare a variable using the "var" keyword, it will be in the global scope(accessible to the whole program) if declared outside all functions. It will have a local scope(accessible within the function only) if defined inside a function.
- If you declare a variable using the "let" keyword, it will be blocked scope, i.e., any variable declared using let, will be accessible with the surrounding curly brackets ({ }) only.
- If you declare a variable using the "const" keyword, you will not be able to change its value later on. As per scope, it will be the same as variables declared using the "let" keyword. . To conclude, we now have a fair understanding of JavaScript let Vs var Vs const. Let's now move to the next article under the concept of callback functions in JavaScript.
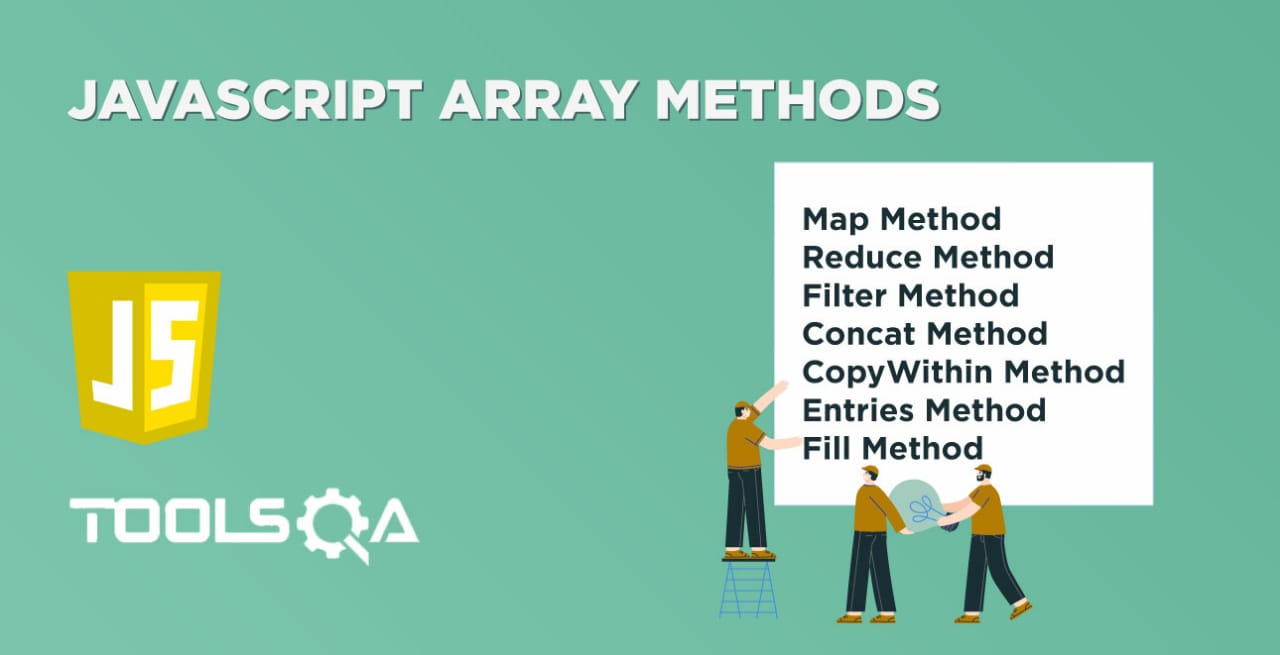
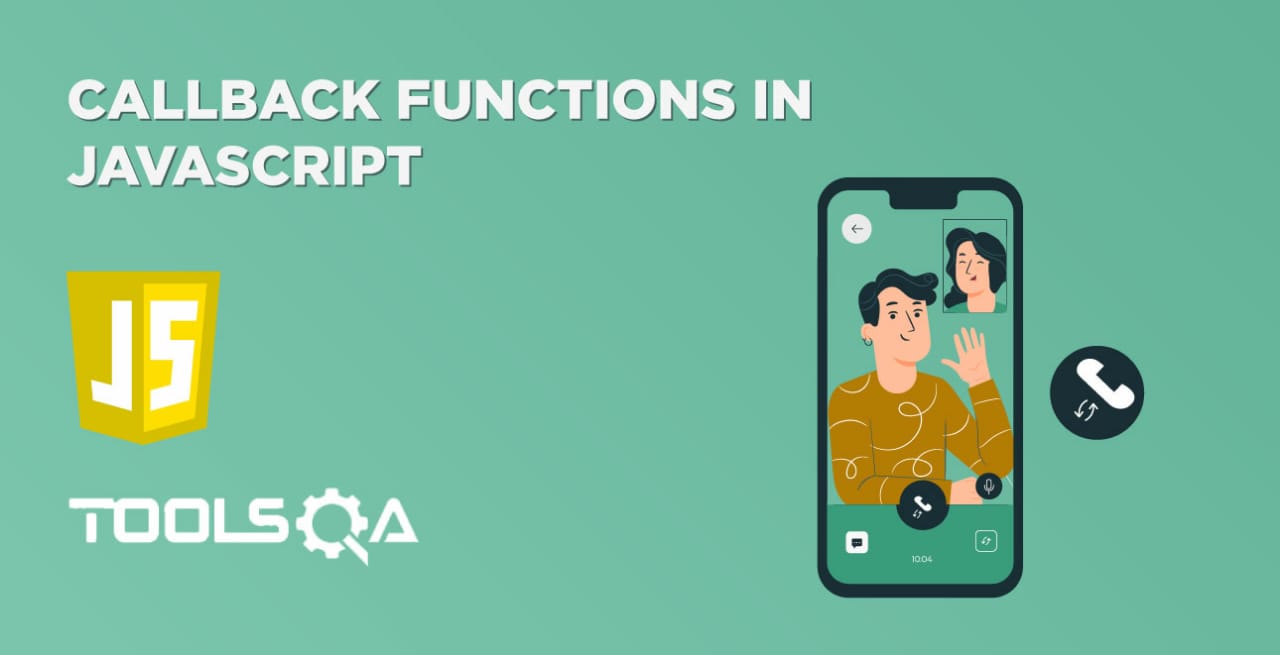