In the tutorial about *generating reports in TestNG, we discussed how we could create different types of reports in TestNG. The report types included the emailable reports and index reports with steps included in Eclipse while using selenium tests. So, are we discussing something different here other than reports? Actually, no! TestNG reporter is an inbuilt class in TestNG, which helps in logging the messages in the output reports. Since logs play a vital role in every tributary of computer science, logging with TestNG Reporter Logs* helps the testers and other team members analyze the details of the code without actually looking at the test code. Consequently, this tutorial will guide you through the following topics:
- What is the Reporter Class in TestNG?
- Syntax for using Reporter class
- How to Log Messages in Reports using TestNG Reporter Log?
- Additionally, how To View Logged Messages In Emailable Report In TestNG?
- How To View Reporter Logs In The Console In TestNG?
What is a Reporter class in TestNG?
Reporter class in TestNG helps the testers log the messages on to the test execution reports. These messages commonly denote the status, information, or any other detail about the test execution. But, with no hard and fast rules, these messages can be anything. A straightforward example of logging with the TestNG reporter log can be logging the chrome driver version used for testing with selenium or logging the inputs you provided into the function etc. Reporter class is elementary to use with and requires just an import into the test code.
Syntax For Using TestNG Reporter Log:
Reporter.log("message");
Import Required: import org.testng.Reporter;
That's it! You just have to call the log function of the reporter class, and the message passed will be logged onto the final reports. Remember to import the class as the reporter class is a separate class available in the org.testng package. It needs to be imported first before using it.
How to Log messages with TestNG Reporter Log using Selenium?
In this section, I will demonstrate how to log the messages in the reports using the reporter class in TestNG. Observe the following code:
java import org.openqa.selenium.WebDriver; import org.testng.Reporter; import org.testng.annotations.Test; import org.openqa.selenium.chrome.ChromeDriver;
public class TestNG { WebDriver driver = new ChromeDriver();
@Test (priority = 0)
public void CloseBrowser() {
driver.close();
Reporter.log("Driver Closed After Testing");
}
@Test (priority = -1)
public void OpenBrowser() {
Reporter.log("This test verifies the current selenium compatibility with TestNG by launching the chrome driver");
Reporter.log("Launching Google Chrome Driver for this test");
driver.get("https://www.demoqa.com");
Reporter.log("The website used was DemoQA for this test");
} }
Notice all the Reporter.log messages typed with the code.
Once you run the code, you will notice that nothing appears on the console. It is because the reporter class has logged the messages on to the reports only. Go to the test-output folder and open index.html report, as shown in the following image:
Open the index.html report in the browser and move to the Reporter Output section in the report.
It will open a panel with all the logged messages visible in the panel.
In this way, you can see the logged messages concerning every method in the test case class. Can we see similar outputs in the other type of report?
How to view Log messages in TestNG Emailable Report?
The logged messages are also available in the emailable report similar to the index report. Once you have run the code in eclipse, open the emailable-report from the test-output folder.
Open this report in the browser.
A nicer version of what we saw in the index reports is available here. So, as you must be thinking, the messages are available only in the reports and do not appear in the console. You can check your console! Can we make some adjustments in the same regard?
How to view TestNG Reporter Logs in the Console?
The log function of the reporter class can also log the messages onto the console simultaneously while logging the messages on the reports. For this, we need to pass an additional boolean parameter. Observe the following syntax for the same:
Reporter.log("message", true);
We can see it in the following code:
java import org.openqa.selenium.WebDriver; import org.testng.Reporter; import org.testng.annotations.Test; import org.openqa.selenium.chrome.ChromeDriver;
public class TestNG { WebDriver driver = new ChromeDriver();
@Test (priority = -1)
public void OpenBrowser() {
Reporter.log("This test verifies the current selenium compatibility with TestNG by launching the chrome driver");
Reporter.log("Launching Google Chrome Driver version 81 for this test");
driver.get("https://www.demoqa.com");
Reporter.log("The website used was DemoQA for this test", true);
driver.close();
} }
In the above code, we have just mentioned the boolean value "true" once with the message, "The website used was DemoQA for this test." Run this test and check the console:
The message gets logged into the console as well in the reports.
The reporter class provides four methods in general:
- Reporter.log(String s);
- Reporter.log(String s, Boolean logToStandardOut);
- Next is, Reporter.log(String s, int level);
- And, Reporter.log(String s, int level, Boolean logToStandardOut);
Logging the messages is an essential task while performing the testing. It is considered a perfect testing practice in the community. You can imagine a scenario where you are stuck somewhere while testing and wants to take the help of the community online. To make them understand the situation, you paste your code so that they can review it and provide support. Through the provided logged messages, the testers community will be able to understand within seconds what you are trying to do rather than understanding the codes. Now, strategically, we can divide logging into two parts: low-level logging and high-level logging.
Low-level logging is when you want to log every step you are taking while testing. Log4J is a popular choice for that. High-level logging is when we just log the critical steps that the tester wants, which the TestNG Reporter Log does.
It was all from my side on TestNG Reporter Logs. In the next tutorial, we will understand the TestNG Asserts and how to use them efficiently in TestNG.
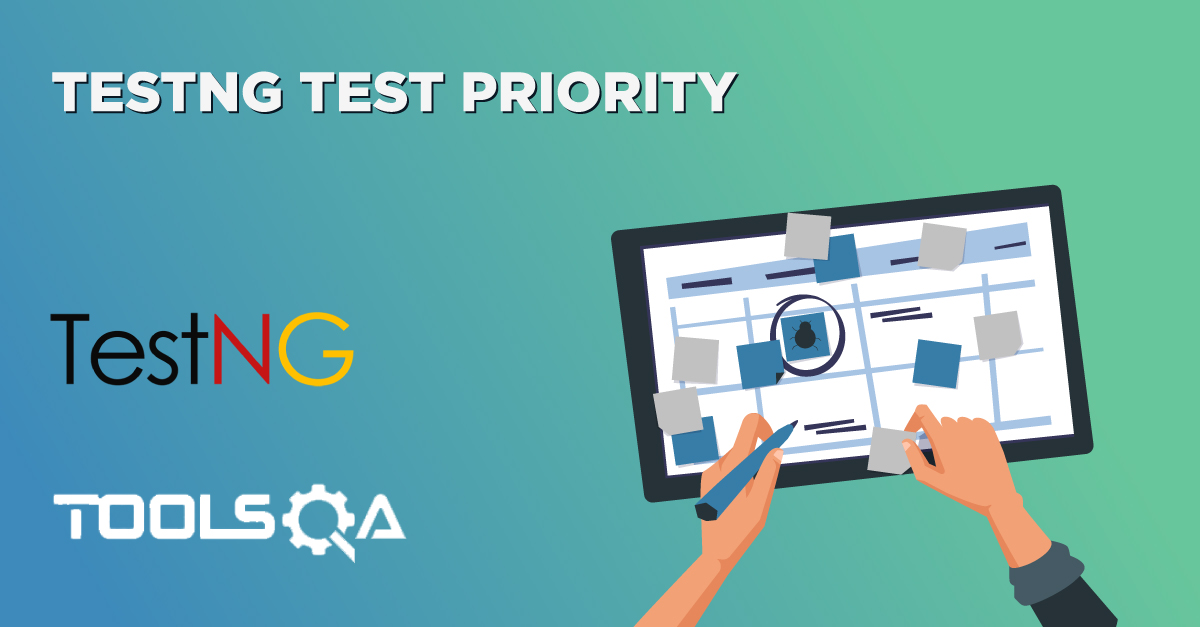
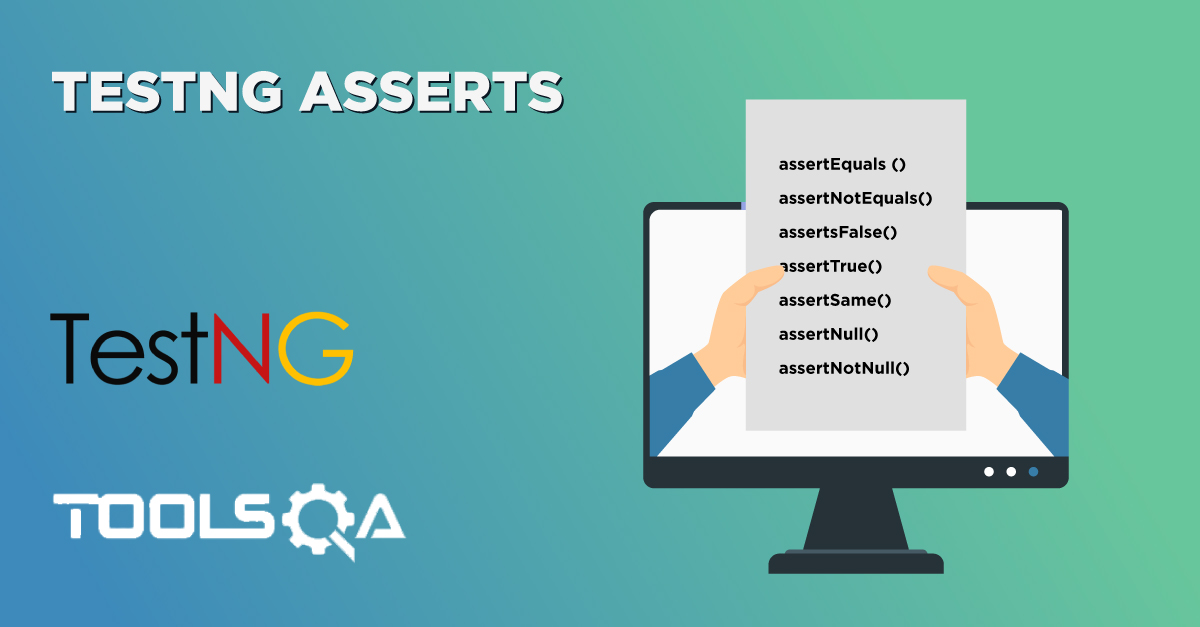