In our series, we have so far covered the basics of Rest Assured, the different types of requests like POST, PUT and DELETE. In addition, we also covered the basics of Authentication & Authorization concepts of Rest API. Practically in the projects, as we proceed with automation, we come across complex APIs. These require basic authentication implementation mixed along with other code. In this article, we will cover the handling of basic authentication in Rest Assured. We will focus on the below points-
- What is Basic Authentication(Auth)?
- What are the different authentication schemes provided by Rest Assured?
- Basic Authentication in Rest Assured.
- Preemptive Authentication.
- Digest Authentication.
- Form Authentication.
- OAuth Authentication.
- OAuth1
- OAuth2
- Basic Authentication in Rest Assured.
What is Basic Authentication (Auth)?
While going through the previous tutorials you must have noticed that we have used the username and the password (authentication credentials) for certain APIs. This credential setting is to enforce access control for the web resources and is generally passed in the header field of an HTTP request. The implementation of basic authentication is to ensure that the APIs are secured and only the users who are authorized have the access to view them. Hence, the authentication information is not encrypted or hashed but encoded as base-64. We will now see the different schemes used in Rest Assured for authentication and you may go through our previous article on Authentication and Authorization for more information.
What are the different authentication schemes provided by Rest Assured?
To test and validate any secured API, you will have to use some authentication scheme. Rest Assured provides several authentication schemes which we are going to discuss in this part.
Before proceeding to understand the use of authentication in Rest Assured, let us execute our Rest Assured test without using any sort of authentication. Below is the code for your reference-
package org.example;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class BasicAuth {
@Test
public void getData() {
RequestSpecification httpRequest = RestAssured.given();
Response res = httpRequest.get("https://postman-echo.com/basic-auth");
ResponseBody body = res.body();
//Converting the response body to string
String rbdy = body.asString();
System.out.println("Data from the GET API- "+rbdy);
}
}
The code is pretty simple and uses the get () method to send requests to the server. Do not worry if you don't understand. It will be explained in the later examples. On executing this code the result would be-
Observe the message in the first line - "Data from the GET API - Unauthorized". This is the issue that we are going to fix using the basic authentication in our rest assured tests. Let us quickly jump on to understanding the same.
Basic Authentication in Rest Assured
As discussed above, the basic authentication scheme uses the username and password in base64 encoded format. The request header needs to contain the credentials of the user for access to the resource. It is very easy to send the credentials using the basic auth and you may use the below syntax-
given().auth().basic("your username", "your password").get("your end point URL");
In the given method you need to append the method of authentication specification followed by the basic HTTP auth where you will pass the credentials as the parameters. Another type of basic authentication is preemptive which we will discuss next.
package org.example;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class BasicAuth {
@Test
public void getData() {
RequestSpecification httpRequest = RestAssured.given().auth().basic("postman", "password");
Response res = httpRequest.get("https://postman-echo.com/basic-auth");
ResponseBody body = res.body();
//Converting the response body to string
String rbdy = body.asString();
System.out.println("Data from the GET API- "+rbdy);
}
}
Preemptive Authentication
By default, Rest Assured uses the challenge-response mechanism. This means that it waits for the server to challenge rather than send the credentials directly. By using the preemptive directives we can avoid that additional call that the server makes and hence additional complications. In a way, it is similar to the basic auth we saw above, the only difference is that an additional premptive () directive adds after auth (). Let us see its syntax followed by a working code example.
given().auth().preemptive().basic("your username", "your password").get("your end point URL");
As you may see above, the preemptive authentication view sends the authentication details in the request header irrespective of being asked by the server. In the same line of implementation, we will see a simple API that uses preemptive authentication.
package org.example;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class BasicAuth {
@Test
public void getUserData() {
//Using the preemptive directive of basic auth to send credentials to the server
RequestSpecification httpRequest = RestAssured.given().auth().preemptive().basic("postman", "password");
Response res = httpRequest.get("https://postman-echo.com/basic-auth");
ResponseBody body = res.body();
//Converting the response body to string
String rbdy = body.asString();
System.out.println("Data from the GET API- "+rbdy);
}
}
The code example used above is a simple Get API where we are trying to fetch the details corresponding to the user. Note that the server needs the authentication details of the user to get a successful response. Let us glide through the code line-by-line.
RequestSpecification httpRequest = RestAssured.given().auth().preemptive().basic("postman", "password");
An object of RequestSpecification is created and using the preemptive directive the credentials of the user are sent in the header. Note that irrespective of being asked for the credentials these would be passed to the server.
Response res = httpRequest.get("https://postman-echo.com/basic-auth");
ResponseBody body = res.body();
The endpoint URL is accessed using the get method and the response is saved using the ResponseBody object.
String rbdy = body.asString();
System.out.println("Data from the GET API- "+rbdy);
Finally, we convert the response body to string and print the result. Similarly, you may add additional validations as per your requirements.
Console prints the response of the above code without errors.
And there you go! You have successfully retrieved the user data by simply adding the preemptive authentication in your code and passing the credentials.
Digest Authentication
It is somewhat similar to challenge-based authentication but is more secure as it uses a digestive key in subsequent requests. If at all it is intercepted by an eavesdropper, he will get access only to the transaction performed and not the user password. The transaction might be replayed but a new transaction cannot be made as the password is not exposed. Its syntax is similar to basic authentication-
given().auth().digest("your username", "your password").get("your end point URL")
Note that we cannot use the preemptive () similar to basic auth since this scheme uses only challenged authentication.
Form Authentication
There can be many cases when you need to pass the authentication credentials in an HTML form. This request is generally sent as a post method where the credentials entered in the form are used for authentication. So, if your application uses such a form-based authentication you can easily automate it using the form() scheme. The syntax for it follows-
given ().auth ().digest ("your username", "your password").get ("your endpoint URL")
given() .auth().form("your username", "your password").post("your end point URL")
If you use this approach then Rest Assured will first have to parse through the HTML response to find the fields for input and then send the form parameters. However, there is a high possibility that this approach might fail if the webpage is complex. Additionally, it would also fail if the context path is not included in the action attribute of the service. To optimize it to handle such cases, you may use the below format where you explicitly pass the required fields by providing the FormAuthConfig()-
given().auth().form("your username", "your password", new FormAuthConfig("/perform_signIn","user","password"))
OAuth Authentication
Another type of authentication is OAuth authentication. OAuth is an authorization framework that defines an identity protocol. It has wide usage in web applications and there are high chances that you will have to automate those authentication actions. These can be of two types viz, OAuth 1.0 and OAuth 2.0 which we will discuss now.
OAuth 1.0
Secured resources built using OAuth 1.0 requires passing consumer key, secret, access token, and token secret. The syntax it follows is -
given().auth().oauth(consumerKey, consumerSecret, accessToken, tokenSecret).get("your end point URL")
OAuth parameters read the required user input dynamically.
OAuth 2.0
There are cases when we need to generate an access token for a user session. This access token performs various transactions and helps maintain the user session. While using OAuth 2.0 you need to directly pass the access token generated when the user login using the below syntax-
given().auth().oauth2("Access token").get("your end point URL")
Using the access token you can easily request any of the resources secured using the OAuth scheme.
Now you may identify the types of authentication used in your web application. Similarly, you can use the corresponding authentication scheme to make full use of rest assured capabilities.
Note: Corresponding Postman tutorial for basic auth can be found at Basic Authentication in Postman.
Also, note that the video tutorial for this topic is available at Basic Authentication in Rest Assured
Key Takeaways
- Basic authentication helps you access the secured APIs and perform actions on the resources.
- Rest assured has four types of authentication schemes. They are basic, digest, form, and OAuth authentication.
- By default, rest assured uses a challenge-response mechanism. But, a preemptive directive sends the credentials without waiting for the server.
- OAuth has two types - OAuth1.0 or OAuth2.0.
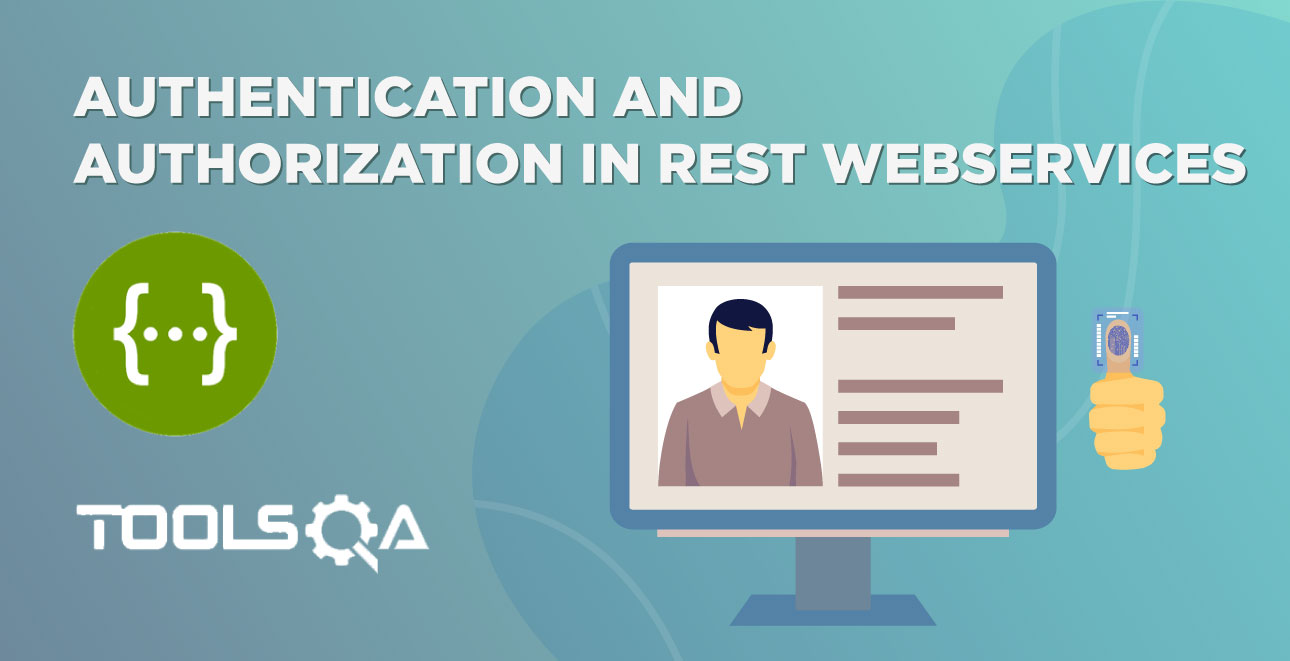
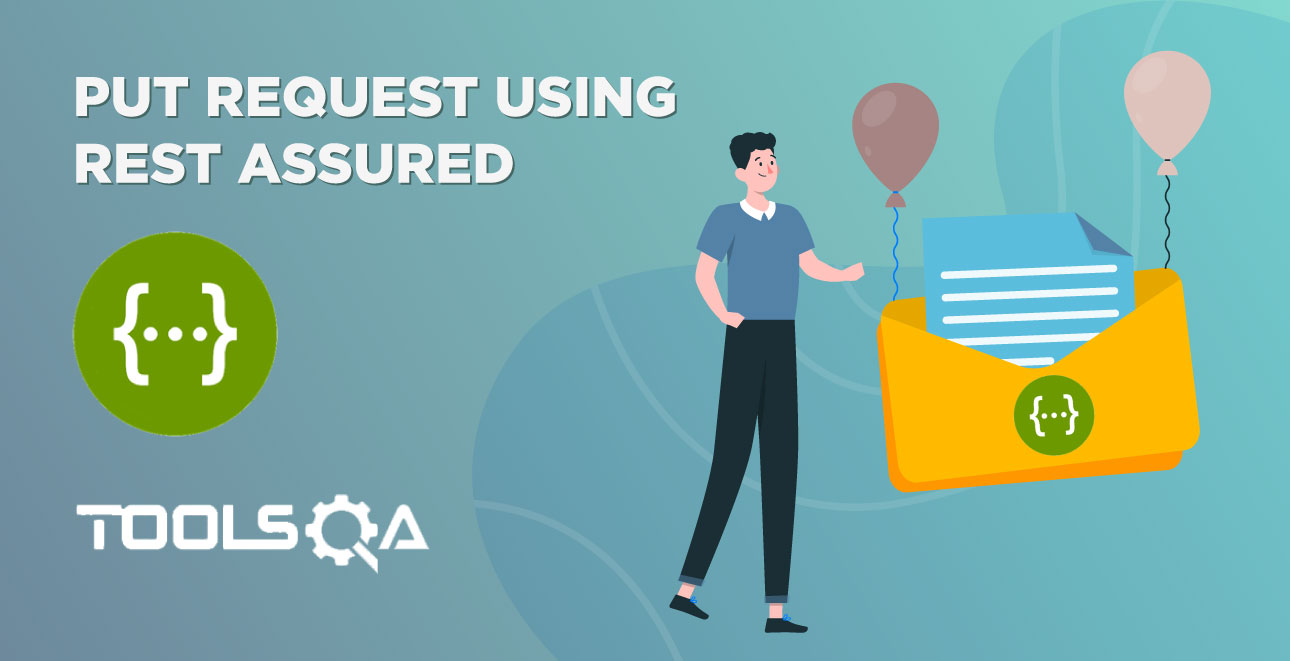