We have seen all the types of HTTP requests so far with respect to Rest Assured. Since these requests are distributed in various tutorials, a collective reference can serve as a bookmark for our readers as they get their hands dirty with rest assured. In this short post, we have combined rest assured examples with various HTTP requests with hands-on code for each of them. This is also reflected in the index as below:
- Rest Assured examples with HTTP API Requests
- HTTP GET Request Implementation
- POST Request Implementation
- HTTP PUT Request Implementation
- HTTP DELETE Request Implementation
REST assured examples with HTTP API Requests
Rest Assured API supports functionality for various HTTP Requests like GET, POST, PUT, DELETE. Let us briefly discuss each of these methods and also walkthrough implementation of each method in Rest Assured.
HTTP GET Request Implementation
The HTTP GET request is used widely in the API world. We can use this request to fetch/get a resource from a server without sending any data with our requests. Since we are focusing on examples only in this post, you can refer to the GET request post here.
Let us now implement the GET request using Rest Assured. The code for the same is shown below.
import io.restassured.RestAssured;
import io.restassured.http.Method;
import io.restassured.response.Response;
import io.restassured.specification.RequestSpecification;
public class RestAssuredAPITest {
@Test
public void GetBooksDetails() {
// Specify the base URL to the RESTful web service
RestAssured.baseURI = "https://demoqa.com/BookStore/v1/Books";
// Get the RequestSpecification of the request to be sent to the server.
RequestSpecification httpRequest = RestAssured.given();
// specify the method type (GET) and the parameters if any.
//In this case the request does not take any parameters
Response response = httpRequest.request(Method.GET, "");
// Print the status and message body of the response received from the server
System.out.println("Status received => " + response.getStatusLine());
System.out.println("Response=>" + response.prettyPrint());
}
}
In the above code, we send a GET request to fetch the list of books and details of each book.
POST Request Implementation
Suppose we want to post/send some data on the server or create a resource on the server, then we go for an HTTP POST request. For more information on the same, you can refer to Understanding HTTP POST Request Method using Rest Assured for more details.
The following code implements the HTTP POST request using Rest Assured.
public void postRequestBooksAPI()
{
RestAssured.baseURI = "https://demoqa.com";
RequestSpecification request = RestAssured.given();
// JSONObject is a class that represents a Simple JSON.
// We can add Key - Value pairs using the put method
JSONObject requestParams = new JSONObject();
requestParams.put("userId", "TQ123");
requestParams.put("isbn", "9781449325862");
// Add a header stating the Request body is a JSON
request.header("Content-Type", "application/json"); // Add the Json to the body of the request
request.body(requestParams.toJSONString()); // Post the request and check the response
Response response = request.post("/BookStore/V1/Books");
System.out.println("The status received: " + response.statusLine());
}
Here we post a request to BookStore and get the response from the API.
HTTP PUT Request Implementation - Rest Assured Examples
The HTTP PUT request either update a resource or substitutes the representation of the target resource with the full JSON request payload. For a detailed discussion on HTTP PUT requests, visit PUT Request using Rest Assured.
The code below makes a PUT request using Rest Assured.
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class UpdateBook {
String userId= "toolsqa_test";
String baseUrl="https://demoqa.com";
String token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyTmFtZSI6InRlc3RpbmcxMjMiLCJwYXNzd29yZCI6IlBhc3N3b3JkQDEiLCJpYXQiOjE2Mjg1NjQyMjF9.lW8JJvJF7jKebbqPiHOBGtCAus8D9Nv1BK6IoIIMJQ4";
String isbn ="9781449325865";
@Test
public void updateBook() {
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
//Calling the Delete API with request body
Response res = httpRequest.body("{ \"isbn\": \"" + isbn + "\", \"userId\": \"" + userId + "\"}").put("/BookStore/v1/Book/9781449325862");
//Fetching the response code from the request and validating the same
System.out.println("The response code - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),200);
}
}
Here, we update the book record with the given ISBN value by setting a new ISBN value and also setting the userId value.
HTTP DELETE Request Implementation
We can delete a resource from a server by making a DELETE request. We have a detailed description of the DELETE request in the article, DELETE Request using Rest Assured.
The following Rest assured example demonstrates the working of HTTP DELETE Request.
package bookstore;
import org.testng.Assert;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Test;
import io.restassured.RestAssured;
import io.restassured.response.Response;
import io.restassured.response.ResponseBody;
import io.restassured.specification.RequestSpecification;
public class DeleteBook {
String userId= "de5d75d1-59b4-487e-b632-f18bc0665c0d";
String baseUrl="https://demoqa.com";
String token = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VyTmFtZSI6InRlc3RpbmcxMjMiLCJwYXNzd29yZCI6IlBhc3N3b3JkQDEiLCJpYXQiOjE2Mjg1NjQyMjF9.lW8JJvJF7jKebbqPiHOBGtCAus8D9Nv1BK6IoIIMJQ4";
String isbn ="9781449337711";
@Test
public void deleteBook() {
RestAssured.baseURI = baseUrl;
RequestSpecification httpRequest = RestAssured.given().header("Authorization", "Bearer " + token)
.header("Content-Type", "application/json");
//Calling the Delete API with request body
Response res = httpRequest.body("{ \"isbn\": \"" + isbn + "\", \"userId\": \"" + userId + "\"}").delete("/BookStore/v1/Book");
//Fetching the response code from the request and validating the same
System.out.println("The response code is - " +res.getStatusCode());
Assert.assertEquals(res.getStatusCode(),204);
}
}
The above code is for the HTTP DELETE request. Here we pass the ISBN and the userId for which the resource is to be deleted. The response obtained confirms the deletion (or if not).
Key TakeAways
In this article, we have presented programming examples of various HTTP requests using the REST Assured library. You can go through the details of each of the requests using the link provided in the description of each method above.
- HTTP GET request is to fetch a particular resource from the server.
- The HTTP POST request posts or sends information or create a new resource on the server.
- HTTP PUT request updates a particular resource or substitutes the representation of the target resource.
- An HTTP DELETE request deletes a particular resource from the server.
Each of the above methods can be programmatically simulated using Rest Assured.
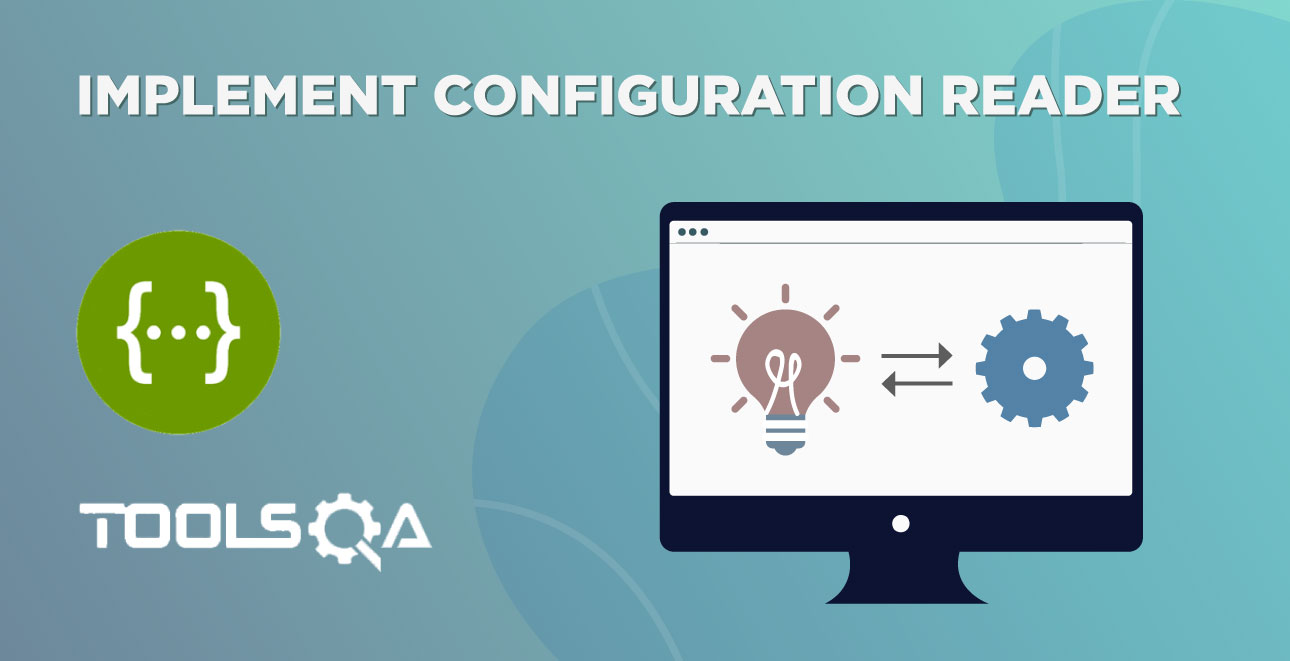