In our discussions of HTTP REST Methods, we went through the GET request in our earlier tutorials. We have also discussed validations like validating headers and the status of the response obtained from the server. In this article, we'll discuss the next method in REST API i.e. the POST request using Rest Assured library. We'll cover the following topics in this article.
- What is an HTTP POST request method?
- How to use POST request method using Rest Assured?
- Create a Request pointing to the Service Endpoint.
- Create a JSON request which contains all the fields.
- Add JSON body in the request and send the Request.
- Validate the Response.
- Changing the HTTP Method on a POST Request using Rest Assured.
What is an HTTP Post Request method?
We use the verb "Post" everywhere when we are dealing with the web. For example, when we are submitting any registration form on a particular webpage like Gmail. We provide the required data and click submit. So through this action of submitting data, we are actually POSTING or sending the data to the server. The verb "POST" in HTTP, also called as POST request method in HTTP sends data to the server. In our example, we are sending our names, email and decided password as data.
Now let's go to Swagger UI of API https://demoqa.com/BookStore/v1/Books Next, let's create a new user using POST request as shown in the following screenshot.
In the above example, we have provided all the details for the new user including id and ISBN. When we "execute" requests we initiate a POST request and send all the information to the server. On the server side, the new user will be created with all the provided information and a response will be sent back to the client. Look at the following screenshot.
Have a look at the "curl" command that has a POST request with all the data. The operation is successful and hence we get the response code as 201.
The content-type parameter is "application/JSON" in the above example. The data sent to the server by POST request can also be XML(content-type: application/XML). This data is in the body of the HTTP request. As we learned from the above example, the POST method usually creates a new record in the application database. Although, it is not always true. Sometimes in creating web applications we use POST request redirections to ensure that no one hits that URL directly without proper navigation channels. For example, you must have noticed that refreshing or pressing "back" on payment pages does not work directly. It is secure as the data being sent is not visible in the URL string. The data can also be encrypted using HTTPS (HTTP Secure) to enhance security.
As far as real-world applications are concerned, the POST request can have a very big size and also a complex body structure. Since it provides an extra layer of security, we often use it for passing sensitive business-related data. It is also noteworthy here that the POST request does not always require all the data to be filled by the user. It completely depends on the server implementation and therefore you should always go through the documentation before.
Let us now use Rest Assured to simulate an HTTP POST request.
How to use POST Request Method using Rest Assured?
In Rest Assured, we make use of the post() method to make an HTTP POST request.
For making an HTTP Post request using Rest Assured, let's add a simple JSON library in our classpath so that we can create JSON objects in the code. We can use the following URL to download simple JSON from the Maven: https://mvnrepository.com/artifact/com.googlecode.json-simple/json-simple. Once the jar is downloaded we can add it to the classpath.
Following are the steps we'll follow to make a POST Request using Rest Assured.
- Create a Request pointing to the service Endpoint.
- Create a JSON Request which contains all the fields.
- Add JSON body in the request and send the request.
- Validate the Request.
- Changing the HTTP Method on a POST Request.
Let us now perform each step one by one.
Create a Request pointing to the Service Endpoint
We can start coding once the JSON jar downloads.
Consider following code lines.
RestAssured.baseURI = "https://demoqa.com/BookStore/v1/Books";
RequestSpecification request = RestAssured.given();
In the above code, we initialize a base URI with a link to the bookstore and the 'createUser' API. Next, we create a 'request' using RequestSpecification.
Note: Above code is self-explanatory. In case of any problems, please refer to previous tutorials.
Create a JSON request which contains all the fields
Next, we will create a JSON request object that will contain the data we need to create a new user. Given below is the code for the same:
// JSONObject is a class that represents a Simple JSON.
// We can add Key - Value pairs using the put method
JSONObject requestParams = new JSONObject();
requestParams.put("userId", "TQ123");
requestParams.put("isbn", "9781449325862");
In the above code, we have created a JSONObject variable (JSONObject belongs to org.json.simple package). Here we provide a JSON String containing the data to post to the server. Here we have the requestParams object above for our test web service with multiple nodes in the JSON. Each node is added to the JSON string using the JSONObject.put(String, String) method. Once all the nodes are added in this manner, we get the String representation of JSONObject by calling JSONObject.toJSONString() method.
Add JSON body in the request and send the Request
Now that we have created the JSON string with the required data, the next step will be to add this JSON to the request body and send or post the request. Look at the following code:
// Add a header stating the Request body is a JSON
request.header("Content-Type", "application/json"); // Add the Json to the body of the request
request.body(requestParams.toJSONString()); // Post the request and check the response
So in this step, we simply add the JSON String to the body of the HTTP Request and set the Content-Type header field value to application/JSON. Next, we use the method RequestSpecification.body(JsonString) to put the JSON body into the request. Using this method we can update the content of the HTTP Request Body.
Next using the post () method on the request object we send this data to the server using the 'BookStoreV1BooksPost' API.
Note: Calling the RequestSpecification.body method multiple times updates the body each time to the latest JSON String.
Validate the Response
After posting the request we have to validate the response we received from the server as a result of a POST request. Given below is the code for validating the response:
Response response = request.post("/BookStoreV1BooksPost");
System.out.println("The status received: " + response.statusLine());
Here we read the status obtained using the statusLine() method and print it to the console.
Changing the HTTP Method on a POST Request using Rest Assured
So what happens when we change the HTTP Request method on a POST request? For example what happens when instead of the expected POST we send the GET? Let's discuss this scenario.
Following is the code wherein we have sent a GET request to an Endpoint when it actually expects POST.
public void UserRegistrationSuccessful()
{
RestAssured.baseURI ="https://demoqa.com/Account/v1";
RequestSpecification request = RestAssured.given();
JSONObject requestParams = new JSONObject();
requestParams.put("userName", "test_rest");
requestParams.put("password", "Testrest@123");
request.body(requestParams.toJSONString());
Response response = request.put("/User");
ResponseBody body = response.getBody();
System.out.println(response.getStatusLine());
System.out.println(body.asString());
}
When we execute the above code, we get the following response.
We can clearly see the output says the incorrect usage of the HTTP Request Method. Similarly, we have other negative scenarios listed below which we will leave to users to try themselves.
- Sending incomplete POST Data.
- Try Sending JSON data with incorrect syntax.
- Sending incorrect HTTP request method in the Request.
You can try the above scenarios on the same URL used above to demonstrate the POST request.
Note: Corresponding Postman tutorial for Post request can be found at Response in Postman.
The video tutorial for this topic is available at What is Post Request?
Key TakeAways
In this article, we discussed the HTTP POST request method using Rest Assured.
- Post request is used to send or post the data to the server.
- Post request mostly results in creating a new record in the database. It can also update the existing record in the database.
- Rest Assured uses a post () method to make HTTP POST requests.
- We usually send the JSON data along with the request object and then POST it to the server.
In the next tutorial, we'll cover the Deserialization technique to convert the JSON Response body into a Class instance.
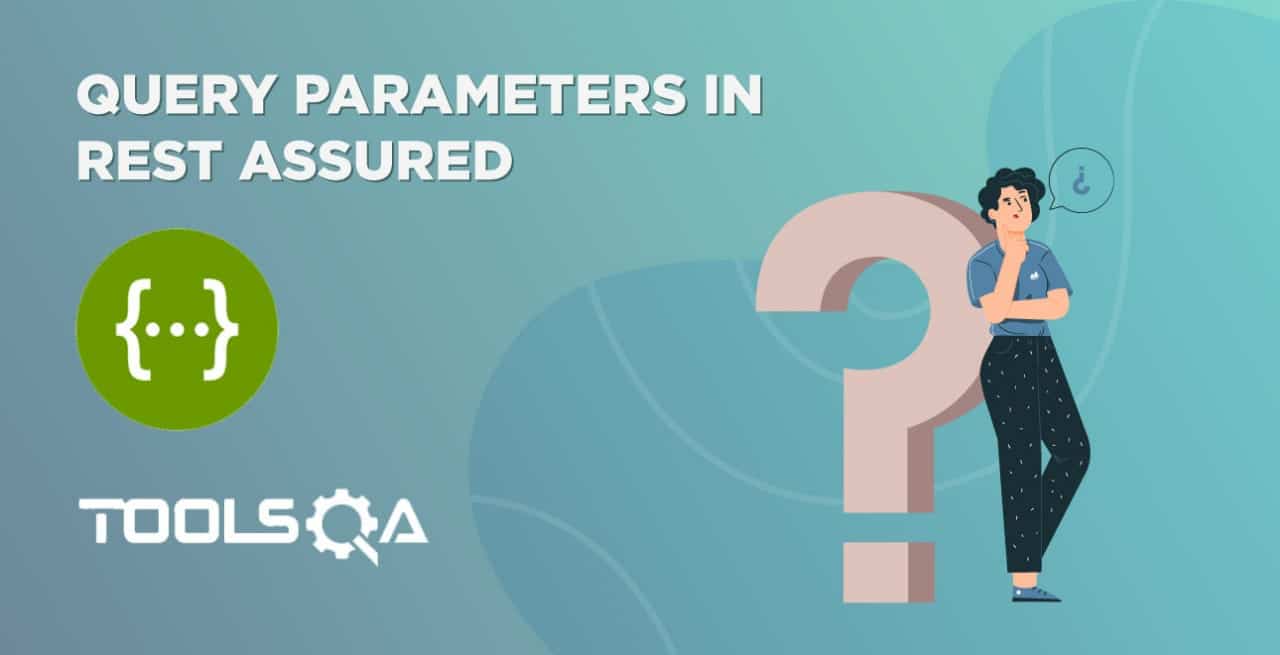
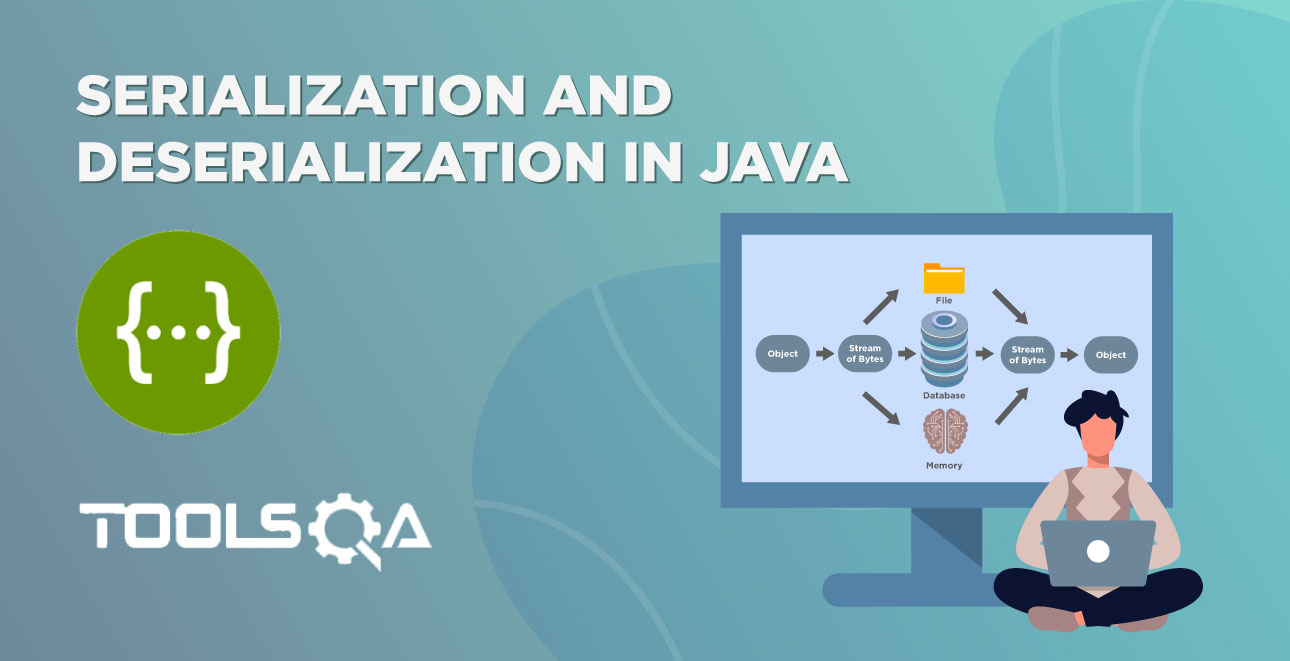