In this tutorial we will learn how to set up Hybrid - Keyword Driven Framework. I called it with the prefix Hybrid because, Originally it is Just a Keyword Driven Framework but when we implement Data Driven technique in it, it can be called Hybrid - Keyword Framework.
Steps to Set Up Keyword Driven Framework:
STEP 1: Automate an End 2 End flow
STEP 2: Identify & Implement Action Keywords
STEP 3: Set Up Data Engine - Excel Sheet
STEP 4: Implement Java Reflection class to create Action Keywords run time
STEP 5: Set Up Java Constant Variables for fixed data
STEP 6: Set Up Object Repository properties file
STEP 7: Set Up Test Suite Execution Engine
STEP 8: Set Up Log4j Logging in Framework
STEP 9: Set Up Exception Handling in Framework
STEP 10: Set Up Test Result Reporting
STEP 11: Set Up Data Driven Technique in Framework
STEP 12: Set Up Framework for Manual Testers
STEP 1: Automate an End 2 End flow
The very first step is to automate an end to end flow of the application. For e.g. If I choose to automate an E-Commerce application, the end to end flow will be like:
LogIn to the application Choose a Product Add the Product to the Cart View the Check Out page Provide Personal details Provide Payment details Confirm the Order and verify the Confirmation page
Prerequisite:
- Java is installed on your computer, to learn more visit Set Up Java.
- Eclipse IDE should be installed, to learn more visit Set Up Eclipse.
- WebDriver client is installed on your machine, to learn more visit Set Up WebDriver Java Client.
- Eclipse IDE is configured with Selenium WebDriver on your machine, to learn more visit Configure Eclipse with WebDriver.
Now lets write a simple test case of LogIn & LogOut which we discussed on previous chapter. I could have taken the complete end to end flow, but it will increase the size of the chapters a lot.
How to do it…
- Create a ‘New Package’ file and name it as ‘executionEngine’, by right click on the Project and select New > Package. We will be creating different packages for Utilities & Configuration files. It is always recommended to use this structure, as it is easy to understand, easy to use and easy to maintain.
- Create a ‘New Class’ file and name it as 'DriverScript', by right click on the above created Package and select New > Class. As this will be our main script, so do not forget to click the check box for 'public static void main(string [ ]args)' while creating class.
- Write the Selenium WebDriver test case for the below mentioned steps:
- Step 1 - Open a Browser
- Step 2 - Navigate to URL
- Step 3 - Click on My Account button
- Step 4 - Enter Username
- Step 5 - Enter Password
- Step 6 - Click on LogIn button
- Step 7 - Click on LogOut button
- Step 8 - Close the Browser
package executionEngine;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.*;
import org.openqa.selenium.firefox.FirefoxDriver;
public class DriverScript {
private static WebDriver driver = null;
public static void main(String[] args) {
driver = new FirefoxDriver();
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
driver.get("https://www.store.demoqa.com");
driver.findElement(By.xpath(".//*[@id='account']/a")).click();
driver.findElement(By.id("log")).sendKeys("testuser_3");
driver.findElement(By.id("pwd")).sendKeys("Test@123");
driver.findElement(By.id("login")).click();
driver.findElement (By.xpath(".//*[@id='account_logout']/a")).click();
driver.quit();
}
}
Try giving it a run. From next chapter, we will start setting up the Keyword Driven Framework by following step by step tutorial.
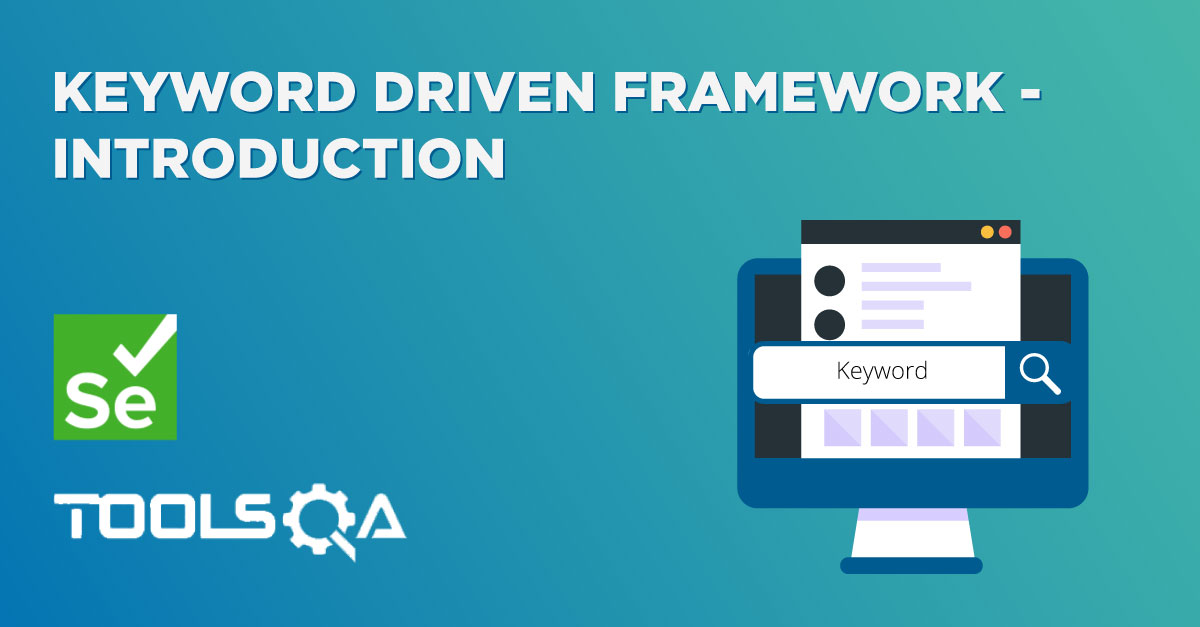
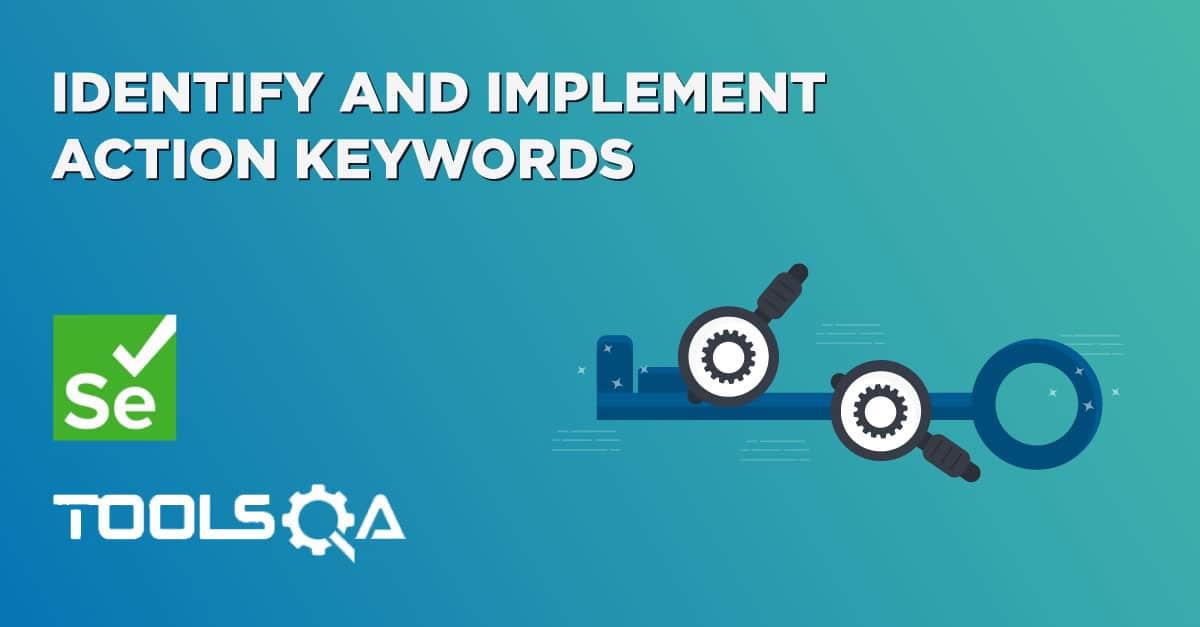