In the last chapters of Cucumber Hooks & Cucumber Tags , we learned that how what are Hooks & Tags and their importance and their usage in Cucumber tests. In this chapter we will look at the concept of Tagged Hook in Cucumber.
Now we know that if we need to do anything before or after the test, we can use @Before & @After hooks. But this scenario works till the time our prerequisites are the same for all the scenarios. For example till the time prerequisite for any test is to start the browser, hooks can solve our purpose. But what if we have different perquisites for different scenarios. And we need to have different hooks for different scenarios.
Again, Cucumbers has given a feature of Tagged Hooks to solve the above situation where we need to perform different tasks before and after scenarios.
Tagged Hooks in Cucumber
Lets again start with doing a simple exercise to get the concept straight. Just keep three different scenarios in the feature file with the same Given, When & Then steps.
1)-First step is to annotate required scenarios using @ + AnyName at the top of the Scenario. For this example, I just annotate each scenario with the sequence order of it, like @First, @Second & @Third.
Feature File
Feature: Test Tagged Hooks
@First
Scenario: This is First Scenario
Given this is the first step
When this is the second step
Then this is the third step
@Second
Scenario: This is Second Scenario
Given this is the first step
When this is the second step
Then this is the third step
@Third
Scenario: This is Third Scenario
Given this is the first step
When this is the second step
Then this is the third step
- Create a Step definition file and just print the execution order of the steps in the console.
Step Definitions
package stepDefinition;
import cucumber.api.java.en.Given;
import cucumber.api.java.en.Then;
import cucumber.api.java.en.When;
public class Hooks_Steps {
@Given("^this is the first step$")
public void This_Is_The_First_Step(){
System.out.println("This is the first step");
}
@When("^this is the second step$")
public void This_Is_The_Second_Step(){
System.out.println("This is the second step");
}
@Then("^this is the third step$")
public void This_Is_The_Third_Step(){
System.out.println("This is the third step");
}
}
- Define tagged hooks in Hooks class file. Hooks can be used like @Before("@TagName"). Create before and after hooks for every scenario. I have also added normal before and after hooks, in case you are not aware, please go to the previous chapter of Hooks in Cucumber.
Hooks
package utilities;
import cucumber.api.java.After;
import cucumber.api.java.Before;
public class Hooks {
@Before
public void beforeScenario(){
System.out.println("This will run before the every Scenario");
}
@After
public void afterScenario(){
System.out.println("This will run after the every Scenario");
}
@Before("@First")
public void beforeFirst(){
System.out.println("This will run only before the First Scenario");
}
@Before("@Second")
public void beforeSecond(){
System.out.println("This will run only before the Second Scenario");
}
@Before("@Third")
public void beforeThird(){
System.out.println("This will run only before the Third Scenario");
}
@After("@First")
public void afterFirst(){
System.out.println("This will run only after the First Scenario");
}
@After("@Second")
public void afterSecond(){
System.out.println("This will run only after the Second Scenario");
}
@After("@Third")
public void afterThird(){
System.out.println("This will run only after the Third Scenario");
}
}
Note: We learned that @Before & @After hooks run before & after every Scenario. But @Before("@First") will run only before the first scenario and likewise other tagged hooks. Again, these tags names can be anything and no need to be first, second and third.
- Run the feature file and observe the output.
Output
Feature: Test Tagged Hooks
This will run only before the First Scenario
This will run before the every Scenario
This is the first step
This is the second step
This is the third step
This will run after the every Scenario
This will run only after the First Scenario
This will run only before the Second Scenario
This will run before the every Scenario
This is the first step
This is the second step
This is the third step
This will run after the every Scenario
This will run only after the Second Scenario
This will run before the every Scenario
This will run only before the Third Scenario
This is the first step
This is the second step
This is the third step
This will run only after the Third Scenario
This will run after the every Scenario
Common Tagged Hooks for Multiple Scenarios
We can have common tagged hooks for multiple scenarios as well. In the below example, I just combined the @Before("First") and @Before("Third") by @Before("@First, @Third"). So in this way we do not need to have two different hooks logic.
Hooks
package utilities;
import cucumber.api.java.After;
import cucumber.api.java.Before;
public class Hooks {
@After
public void afterScenario(){
System.out.println("This will run after the every Scenario");
}
@Before
public void beforeScenario(){
System.out.println("This will run before the every Scenario");
}
@Before("@Second")
public void beforeSecond(){
System.out.println("This will run only before the Second Scenario");
}
@Before("@First,@Third")
public void beforeFirstAndThird(){
System.out.println("This will run before both First & Third Scenario");
}
@After("@First")
public void afterFirst(){
System.out.println("This will run only after the First Scenario");
}
@After("@Second")
public void afterSecond(){
System.out.println("This will run only after the Second Scenario");
}
@After("@Third")
public void afterThird(){
System.out.println("This will run only after the Third Scenario");
}
}
Output
Feature: Test Tagged Hooks
This will run before the every Scenario
This will run before both First & Third Scenario
This is the first step
This is the second step
This is the third step
This will run only after the First Scenario
This will run after the every Scenario
This will run before the every Scenario
This will run only before the Second Scenario
This is the first step
This is the second step
This is the third step
This will run only after the Second Scenario
This will run after the every Scenario
This will run before the every Scenario
This will run before both First & Third Scenario
This is the first step
This is the second step
This is the third step
This will run only after the Third Scenario
This will run after the every Scenario
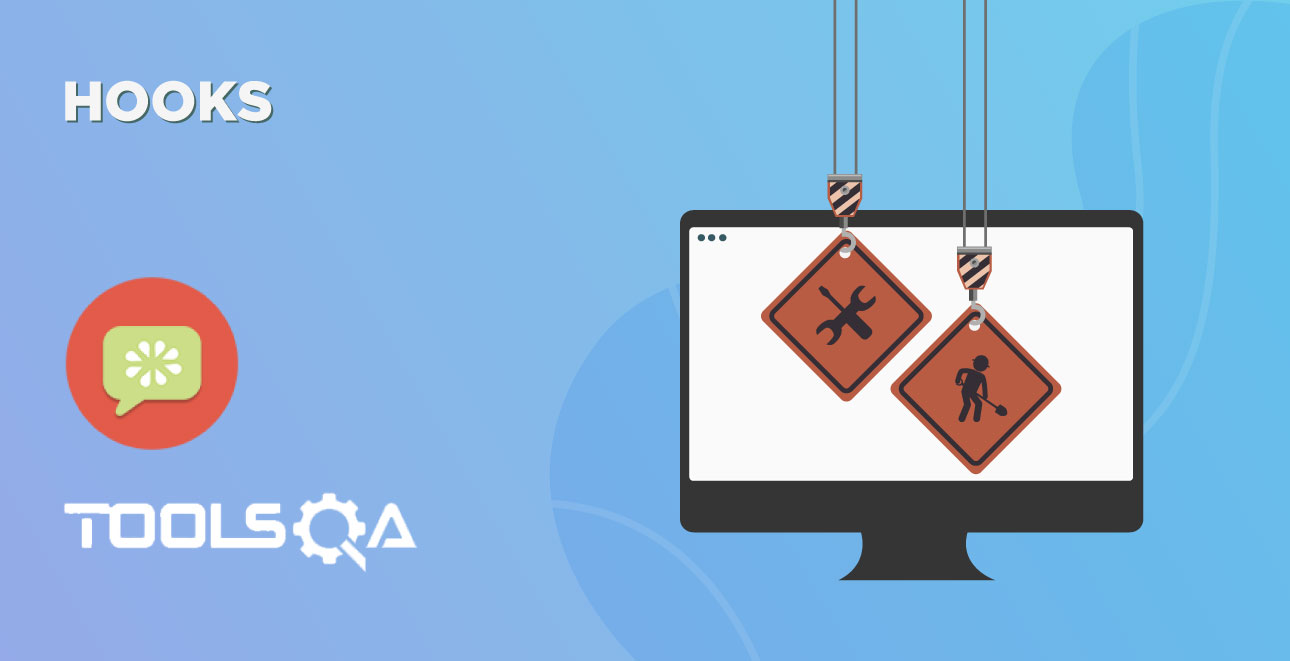
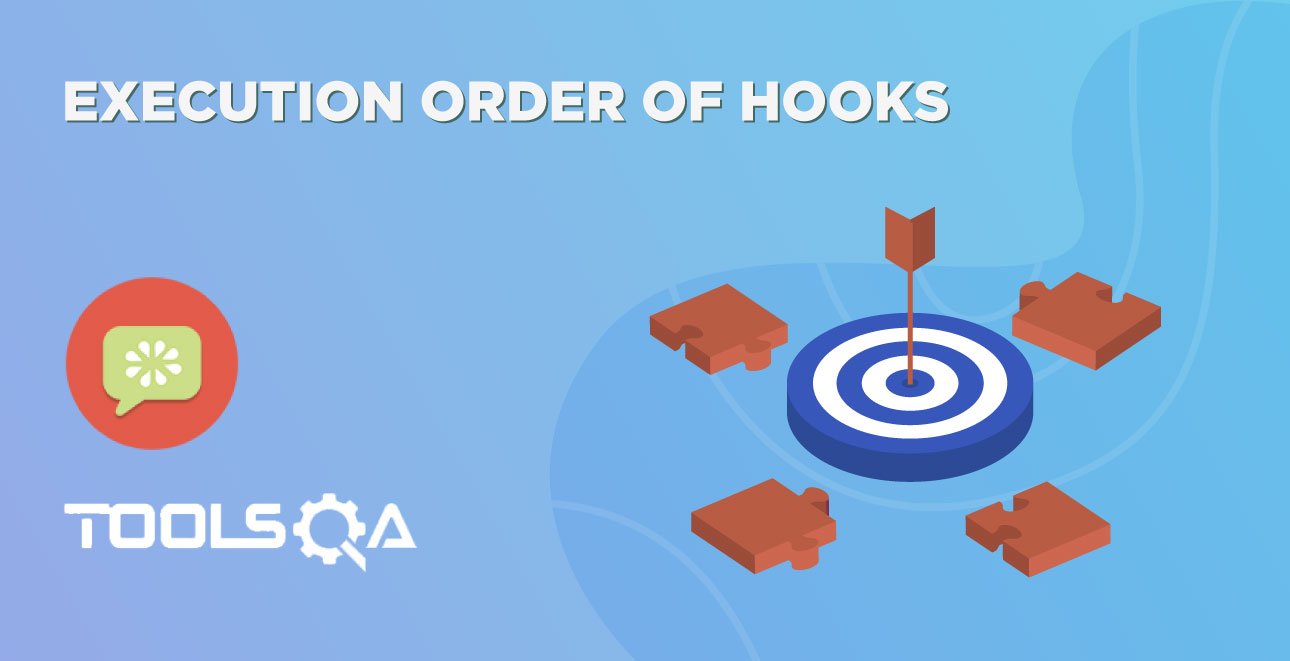