This chapter is all about How to Read Configurations from Property File in Selenium Cucumber Framework or in any framework. It is dangerous to store hard coded values in the project, also it is against the coding principles. And so far we have been using a lot of hard coded values in our code. With the help of properties file, we will be eliminating these hard coded value one by one.
What is a Property file in Java
.properties files are mainly used in Java programs to maintain project configuration data, database config or project settings, etc. Each parameter in properties file is stored as a pair of strings, in key-value pair format, where each key is on one line. You can easily read properties from some file using an object of type Properties. This is a utility provided by Java itself.
java.util.Properties;
Advantages of Property file in Java
If any information is changed from the properties file, you don't need to recompile the java class. In other words, the advantage of using properties file is we can configure things that are prone to change over a period of time without need of changing anything in code.
For E.g. We keep application Url in the property file, so in case you want to run test from on other test environment, just change the Url in a property file and that's it. You do not require to build the whole project again.
How to Read Configurations from Property File
Step 1: Create a Property file
- Create a New Folder and name it as configs, by right click on the root Project and select New >> Folder. We will be keeping all the config files with in the same folder.
- Create a New File by right click on the above created folder and select New >> File.
3). The only thing we need to do is to give the file name and give the extension as .properties. In our case we name it as Configuation.properties.
Step 2: Write Hard Coded Values in the Property File
If we look at our first method of Steps file, we will find that we have been using two hard coded values:
- Driver Path = System.setProperty("webdriver.chrome.driver","C:\ToolsQA\Libs\Drivers\chromedriver.exe");
- Implicit Wait Time = driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
and the URL in the HomePage.java class
- URL = driver.get("http://www.shop.demoqa.com");
- So, let's start writing these values in Property file in Key Value Pair.
Note: The above is the properties file that we will use for the Cucumber tests. Remember white space between the property name and property value is always ignored.
Step 3: Create a Config File Reader
-
Create a New Package and name it as dataProvider, by right click on the src/test/java and select New >> Package. We will keep all the data readers files here in this package.
-
Create a New Class file and name it as ConfigFileReader, by right click on the above-created package and select New >> Class.
ConfigFileReader.java
package dataProviders;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.Properties;
public class ConfigFileReader {
private Properties properties;
private final String propertyFilePath= "configs//Configuration.properties";
public ConfigFileReader(){
BufferedReader reader;
try {
reader = new BufferedReader(new FileReader(propertyFilePath));
properties = new Properties();
try {
properties.load(reader);
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
} catch (FileNotFoundException e) {
e.printStackTrace();
throw new RuntimeException("Configuration.properties not found at " + propertyFilePath);
}
}
public String getDriverPath(){
String driverPath = properties.getProperty("driverPath");
if(driverPath!= null) return driverPath;
else throw new RuntimeException("driverPath not specified in the Configuration.properties file.");
}
public long getImplicitlyWait() {
String implicitlyWait = properties.getProperty("implicitlyWait");
if(implicitlyWait != null) return Long.parseLong(implicitlyWait);
else throw new RuntimeException("implicitlyWait not specified in the Configuration.properties file.");
}
public String getApplicationUrl() {
String url = properties.getProperty("url");
if(url != null) return url;
else throw new RuntimeException("url not specified in the Configuration.properties file.");
}
}
Explanation
How to Load Property File
BufferedReader reader = new BufferedReader(new FileReader(propertyFilePath));
Properties properties = new Properties();
properties.load(reader);
- propertyFilePath : This is just a String variable which holds the information of the config file path.
- new FileReader(propertyFilePath) : Creates a new FileReader, given the name of the file to read from.
- new BufferedReader(new FileReader(propertyFilePath)) : Reads text from a character-input stream, buffering characters so as to provide for the efficient reading of characters, arrays, and lines.
- new Properties() : The Properties class represents a persistent set of properties. The Properties can be saved to a stream or loaded from a stream. Each key and its corresponding value in the property list is a string.
- properties.load(reader) : Reads a property list (key and element pairs) from the input character stream in a simple line-oriented format.
ConfigFileReader Method
public String getDriverPath(){
String driverPath = properties.getProperty("driverPath");
if(driverPath!= null) return driverPath;
else throw new RuntimeException("driverPath not specified in the Configuration.properties file.");
}
- properties.getProperty("driverPath") :Properties object gives us a .getProperty method which takes the Key of the property as a parameter and return the Value of the matched key from the .properties file.
- If the properties file does not have the specified key, it returns the null. This is why we have put the null check and in case of null we like to throw an exception to stop the test with the stack trace information.
Step 4: Use ConfigFileReader object in the Steps file
To use the ConfigFileReader object in the test, we need to fist create an object of the class. ConfigFileReader configFileReader= new ConfigFileReader();
Then we can replace the below statement System.setProperty("webdriver.chrome.driver","C:\ToolsQA\Libs\Drivers\chromedriver.exe"); with System.setProperty("webdriver.chrome.driver", configFileReader.getDriverPath());
Complete Steps file will look like this now:
Steps.java
package stepDefinitions;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import cucumber.api.java.en.Given;
import cucumber.api.java.en.When;
import dataProviders.ConfigFileReader;
import managers.PageObjectManager;
import pageObjects.CartPage;
import pageObjects.CheckoutPage;
import pageObjects.HomePage;
import pageObjects.ProductListingPage;
public class Steps {
WebDriver driver;
HomePage homePage;
ProductListingPage productListingPage;
CartPage cartPage;
CheckoutPage checkoutPage;
PageObjectManager pageObjectManager;
ConfigFileReader configFileReader;
@Given("^user is on Home Page$")
public void user_is_on_Home_Page(){
configFileReader= new ConfigFileReader();
System.setProperty("webdriver.chrome.driver", configFileReader.getDriverPath());
driver = new ChromeDriver();
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(configFileReader.getImplicitlyWait(), TimeUnit.SECONDS);
pageObjectManager = new PageObjectManager(driver);
homePage = pageObjectManager.getHomePage();
homePage.navigateTo_HomePage();
}
@When("^he search for \"([^\"]*)\"$")
public void he_search_for(String product) {
homePage.perform_Search(product);
}
@When("^choose to buy the first item$")
public void choose_to_buy_the_first_item() {
productListingPage = pageObjectManager.getProductListingPage();
productListingPage.select_Product(0);
productListingPage.clickOn_AddToCart();
}
@When("^moves to checkout from mini cart$")
public void moves_to_checkout_from_mini_cart(){
cartPage = pageObjectManager.getCartPage();
cartPage.clickOn_Cart();
cartPage.clickOn_ContinueToCheckout();
}
@When("^enter personal details on checkout page$")
public void enter_personal_details_on_checkout_page(){
checkoutPage = pageObjectManager.getCheckoutPage();
checkoutPage.fill_PersonalDetails();
}
@When("^select same delivery address$")
public void select_same_delivery_address(){
checkoutPage.check_ShipToDifferentAddress(false);
}
@When("^select payment method as \"([^\"]*)\" payment$")
public void select_payment_method_as_payment(String arg1){
checkoutPage.select_PaymentMethod("CheckPayment");
}
@When("^place the order$")
public void place_the_order() {
checkoutPage.check_TermsAndCondition(true);
checkoutPage.clickOn_PlaceOrder();
driver.quit();
}
}
And our Home Page object class file will also have the changes.
HomePage.java
package pageObjects;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.PageFactory;
import dataProviders.ConfigFileReader;
public class HomePage {
WebDriver driver;
ConfigFileReader configFileReader;
public HomePage(WebDriver driver){
this.driver = driver;
PageFactory.initElements(driver, this);
configFileReader= new ConfigFileReader();
}
public void perform_Search(String search) {
driver.navigate().to(configFileReader.getApplicationUrl() + "/?s=" + search + "&post_type=product");
}
public void navigateTo_HomePage() {
driver.get(configFileReader.getApplicationUrl());
}
}
Note: Although it is bad practice to create object of property file in every class. We created the object of the same in Steps file and now we created again in the HomePage class. But do not worry for now, I got something special for you in the next chapter.
Run the Cucumber Test
Run as JUnit
Now we are all set to run the Cucumber test. Right Click on TestRunner class and Click Run As >> JUnit Test. Cucumber will run the script the same way it runs in Selenium WebDriver and the result will be shown in the left hand side project explorer window in JUnit tab.
Project Explorer
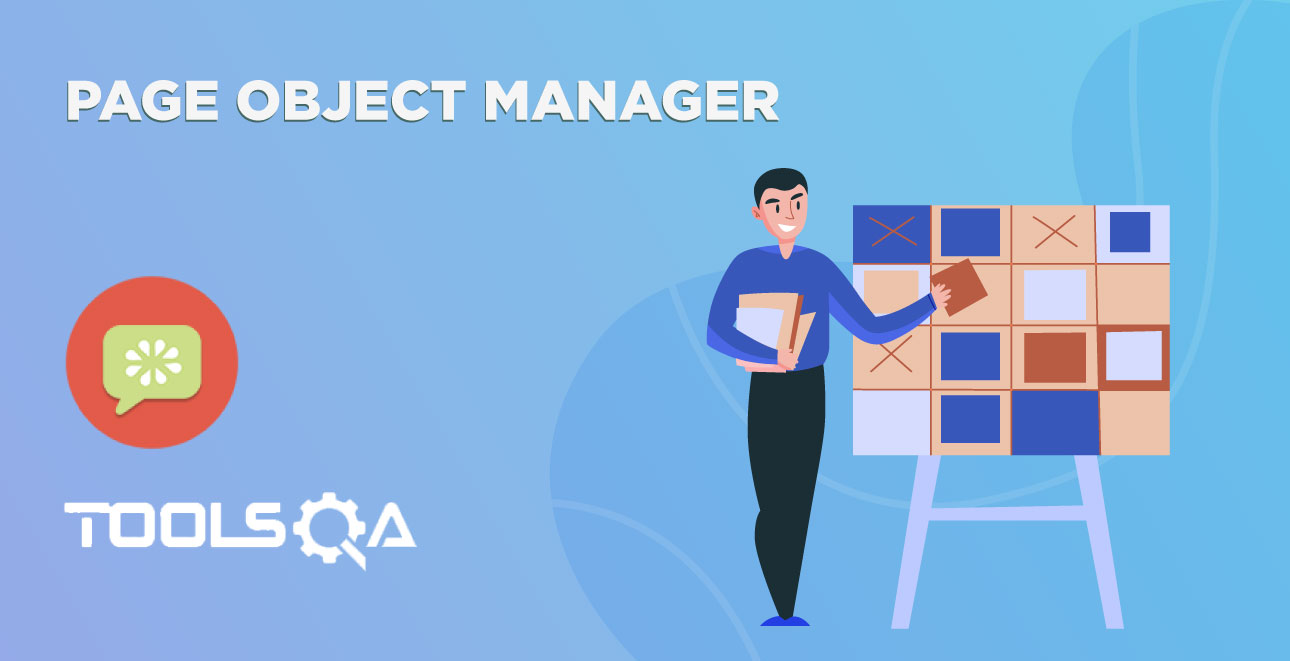
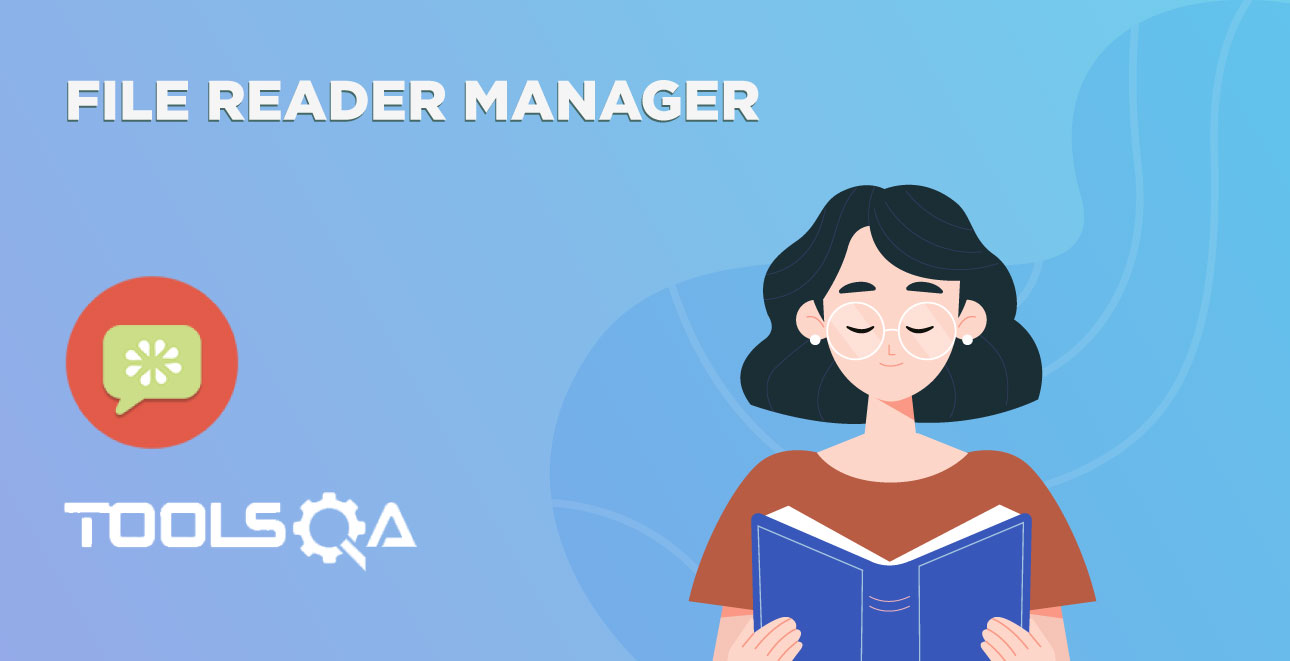